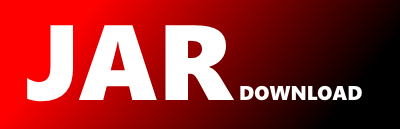
com.pulumi.azurenative.streamanalytics.outputs.OutputResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.streamanalytics.outputs;
import com.pulumi.azurenative.streamanalytics.outputs.AvroSerializationResponse;
import com.pulumi.azurenative.streamanalytics.outputs.AzureDataLakeStoreOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.AzureFunctionOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.AzureSqlDatabaseOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.AzureSynapseOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.AzureTableOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.BlobOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.CsvSerializationResponse;
import com.pulumi.azurenative.streamanalytics.outputs.DiagnosticsResponse;
import com.pulumi.azurenative.streamanalytics.outputs.DocumentDbOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.EventHubOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.EventHubV2OutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.GatewayMessageBusOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.JsonSerializationResponse;
import com.pulumi.azurenative.streamanalytics.outputs.ParquetSerializationResponse;
import com.pulumi.azurenative.streamanalytics.outputs.PowerBIOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.ServiceBusQueueOutputDataSourceResponse;
import com.pulumi.azurenative.streamanalytics.outputs.ServiceBusTopicOutputDataSourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.Object;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class OutputResponse {
/**
* @return Describes the data source that output will be written to. Required on PUT (CreateOrReplace) requests.
*
*/
private @Nullable Object datasource;
/**
* @return Describes conditions applicable to the Input, Output, or the job overall, that warrant customer attention.
*
*/
private DiagnosticsResponse diagnostics;
/**
* @return The current entity tag for the output. This is an opaque string. You can use it to detect whether the resource has changed between requests. You can also use it in the If-Match or If-None-Match headers for write operations for optimistic concurrency.
*
*/
private String etag;
/**
* @return Resource Id
*
*/
private String id;
/**
* @return Resource name
*
*/
private @Nullable String name;
/**
* @return Describes how data from an input is serialized or how data is serialized when written to an output. Required on PUT (CreateOrReplace) requests.
*
*/
private @Nullable Object serialization;
/**
* @return The size window to constrain a Stream Analytics output to.
*
*/
private @Nullable Integer sizeWindow;
/**
* @return The time frame for filtering Stream Analytics job outputs.
*
*/
private @Nullable String timeWindow;
/**
* @return Resource type
*
*/
private String type;
private OutputResponse() {}
/**
* @return Describes the data source that output will be written to. Required on PUT (CreateOrReplace) requests.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy