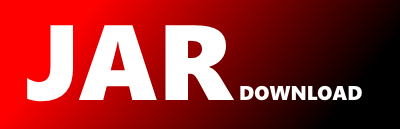
com.pulumi.azurenative.synapse.outputs.GetIotHubDataConnectionResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.synapse.outputs;
import com.pulumi.azurenative.synapse.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetIotHubDataConnectionResult {
/**
* @return The iot hub consumer group.
*
*/
private String consumerGroup;
/**
* @return The data format of the message. Optionally the data format can be added to each message.
*
*/
private @Nullable String dataFormat;
/**
* @return System properties of the iot hub
*
*/
private @Nullable List eventSystemProperties;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The resource ID of the Iot hub to be used to create a data connection.
*
*/
private String iotHubResourceId;
/**
* @return Kind of the endpoint for the data connection
* Expected value is 'IotHub'.
*
*/
private String kind;
/**
* @return Resource location.
*
*/
private @Nullable String location;
/**
* @return The mapping rule to be used to ingest the data. Optionally the mapping information can be added to each message.
*
*/
private @Nullable String mappingRuleName;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The provisioned state of the resource.
*
*/
private String provisioningState;
/**
* @return The name of the share access policy
*
*/
private String sharedAccessPolicyName;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The table where the data should be ingested. Optionally the table information can be added to each message.
*
*/
private @Nullable String tableName;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetIotHubDataConnectionResult() {}
/**
* @return The iot hub consumer group.
*
*/
public String consumerGroup() {
return this.consumerGroup;
}
/**
* @return The data format of the message. Optionally the data format can be added to each message.
*
*/
public Optional dataFormat() {
return Optional.ofNullable(this.dataFormat);
}
/**
* @return System properties of the iot hub
*
*/
public List eventSystemProperties() {
return this.eventSystemProperties == null ? List.of() : this.eventSystemProperties;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The resource ID of the Iot hub to be used to create a data connection.
*
*/
public String iotHubResourceId() {
return this.iotHubResourceId;
}
/**
* @return Kind of the endpoint for the data connection
* Expected value is 'IotHub'.
*
*/
public String kind() {
return this.kind;
}
/**
* @return Resource location.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return The mapping rule to be used to ingest the data. Optionally the mapping information can be added to each message.
*
*/
public Optional mappingRuleName() {
return Optional.ofNullable(this.mappingRuleName);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The provisioned state of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The name of the share access policy
*
*/
public String sharedAccessPolicyName() {
return this.sharedAccessPolicyName;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The table where the data should be ingested. Optionally the table information can be added to each message.
*
*/
public Optional tableName() {
return Optional.ofNullable(this.tableName);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetIotHubDataConnectionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String consumerGroup;
private @Nullable String dataFormat;
private @Nullable List eventSystemProperties;
private String id;
private String iotHubResourceId;
private String kind;
private @Nullable String location;
private @Nullable String mappingRuleName;
private String name;
private String provisioningState;
private String sharedAccessPolicyName;
private SystemDataResponse systemData;
private @Nullable String tableName;
private String type;
public Builder() {}
public Builder(GetIotHubDataConnectionResult defaults) {
Objects.requireNonNull(defaults);
this.consumerGroup = defaults.consumerGroup;
this.dataFormat = defaults.dataFormat;
this.eventSystemProperties = defaults.eventSystemProperties;
this.id = defaults.id;
this.iotHubResourceId = defaults.iotHubResourceId;
this.kind = defaults.kind;
this.location = defaults.location;
this.mappingRuleName = defaults.mappingRuleName;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.sharedAccessPolicyName = defaults.sharedAccessPolicyName;
this.systemData = defaults.systemData;
this.tableName = defaults.tableName;
this.type = defaults.type;
}
@CustomType.Setter
public Builder consumerGroup(String consumerGroup) {
if (consumerGroup == null) {
throw new MissingRequiredPropertyException("GetIotHubDataConnectionResult", "consumerGroup");
}
this.consumerGroup = consumerGroup;
return this;
}
@CustomType.Setter
public Builder dataFormat(@Nullable String dataFormat) {
this.dataFormat = dataFormat;
return this;
}
@CustomType.Setter
public Builder eventSystemProperties(@Nullable List eventSystemProperties) {
this.eventSystemProperties = eventSystemProperties;
return this;
}
public Builder eventSystemProperties(String... eventSystemProperties) {
return eventSystemProperties(List.of(eventSystemProperties));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetIotHubDataConnectionResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder iotHubResourceId(String iotHubResourceId) {
if (iotHubResourceId == null) {
throw new MissingRequiredPropertyException("GetIotHubDataConnectionResult", "iotHubResourceId");
}
this.iotHubResourceId = iotHubResourceId;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetIotHubDataConnectionResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder mappingRuleName(@Nullable String mappingRuleName) {
this.mappingRuleName = mappingRuleName;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetIotHubDataConnectionResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetIotHubDataConnectionResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder sharedAccessPolicyName(String sharedAccessPolicyName) {
if (sharedAccessPolicyName == null) {
throw new MissingRequiredPropertyException("GetIotHubDataConnectionResult", "sharedAccessPolicyName");
}
this.sharedAccessPolicyName = sharedAccessPolicyName;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetIotHubDataConnectionResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tableName(@Nullable String tableName) {
this.tableName = tableName;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetIotHubDataConnectionResult", "type");
}
this.type = type;
return this;
}
public GetIotHubDataConnectionResult build() {
final var _resultValue = new GetIotHubDataConnectionResult();
_resultValue.consumerGroup = consumerGroup;
_resultValue.dataFormat = dataFormat;
_resultValue.eventSystemProperties = eventSystemProperties;
_resultValue.id = id;
_resultValue.iotHubResourceId = iotHubResourceId;
_resultValue.kind = kind;
_resultValue.location = location;
_resultValue.mappingRuleName = mappingRuleName;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.sharedAccessPolicyName = sharedAccessPolicyName;
_resultValue.systemData = systemData;
_resultValue.tableName = tableName;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy