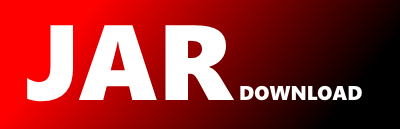
com.pulumi.azurenative.testbase.TestbaseFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.testbase;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.testbase.inputs.GetActionRequestArgs;
import com.pulumi.azurenative.testbase.inputs.GetActionRequestPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetBillingHubServiceFreeHourBalanceArgs;
import com.pulumi.azurenative.testbase.inputs.GetBillingHubServiceFreeHourBalancePlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetBillingHubServiceUsageArgs;
import com.pulumi.azurenative.testbase.inputs.GetBillingHubServiceUsagePlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetCredentialArgs;
import com.pulumi.azurenative.testbase.inputs.GetCredentialPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetCustomImageArgs;
import com.pulumi.azurenative.testbase.inputs.GetCustomImagePlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetCustomerEventArgs;
import com.pulumi.azurenative.testbase.inputs.GetCustomerEventPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetDraftPackageArgs;
import com.pulumi.azurenative.testbase.inputs.GetDraftPackagePathArgs;
import com.pulumi.azurenative.testbase.inputs.GetDraftPackagePathPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetDraftPackagePlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetFavoriteProcessArgs;
import com.pulumi.azurenative.testbase.inputs.GetFavoriteProcessPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetImageDefinitionArgs;
import com.pulumi.azurenative.testbase.inputs.GetImageDefinitionPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetPackageArgs;
import com.pulumi.azurenative.testbase.inputs.GetPackageDownloadURLArgs;
import com.pulumi.azurenative.testbase.inputs.GetPackageDownloadURLPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetPackagePlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetTestBaseAccountArgs;
import com.pulumi.azurenative.testbase.inputs.GetTestBaseAccountFileUploadUrlArgs;
import com.pulumi.azurenative.testbase.inputs.GetTestBaseAccountFileUploadUrlPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetTestBaseAccountPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetTestResultConsoleLogDownloadURLArgs;
import com.pulumi.azurenative.testbase.inputs.GetTestResultConsoleLogDownloadURLPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetTestResultDownloadURLArgs;
import com.pulumi.azurenative.testbase.inputs.GetTestResultDownloadURLPlainArgs;
import com.pulumi.azurenative.testbase.inputs.GetTestResultVideoDownloadURLArgs;
import com.pulumi.azurenative.testbase.inputs.GetTestResultVideoDownloadURLPlainArgs;
import com.pulumi.azurenative.testbase.outputs.GetActionRequestResult;
import com.pulumi.azurenative.testbase.outputs.GetBillingHubServiceFreeHourBalanceResult;
import com.pulumi.azurenative.testbase.outputs.GetBillingHubServiceUsageResult;
import com.pulumi.azurenative.testbase.outputs.GetCredentialResult;
import com.pulumi.azurenative.testbase.outputs.GetCustomImageResult;
import com.pulumi.azurenative.testbase.outputs.GetCustomerEventResult;
import com.pulumi.azurenative.testbase.outputs.GetDraftPackagePathResult;
import com.pulumi.azurenative.testbase.outputs.GetDraftPackageResult;
import com.pulumi.azurenative.testbase.outputs.GetFavoriteProcessResult;
import com.pulumi.azurenative.testbase.outputs.GetImageDefinitionResult;
import com.pulumi.azurenative.testbase.outputs.GetPackageDownloadURLResult;
import com.pulumi.azurenative.testbase.outputs.GetPackageResult;
import com.pulumi.azurenative.testbase.outputs.GetTestBaseAccountFileUploadUrlResult;
import com.pulumi.azurenative.testbase.outputs.GetTestBaseAccountResult;
import com.pulumi.azurenative.testbase.outputs.GetTestResultConsoleLogDownloadURLResult;
import com.pulumi.azurenative.testbase.outputs.GetTestResultDownloadURLResult;
import com.pulumi.azurenative.testbase.outputs.GetTestResultVideoDownloadURLResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class TestbaseFunctions {
/**
* Get the action request under the specified test base account.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getActionRequest(GetActionRequestArgs args) {
return getActionRequest(args, InvokeOptions.Empty);
}
/**
* Get the action request under the specified test base account.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getActionRequestPlain(GetActionRequestPlainArgs args) {
return getActionRequestPlain(args, InvokeOptions.Empty);
}
/**
* Get the action request under the specified test base account.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getActionRequest(GetActionRequestArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getActionRequest", TypeShape.of(GetActionRequestResult.class), args, Utilities.withVersion(options));
}
/**
* Get the action request under the specified test base account.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getActionRequestPlain(GetActionRequestPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getActionRequest", TypeShape.of(GetActionRequestResult.class), args, Utilities.withVersion(options));
}
/**
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getBillingHubServiceFreeHourBalance(GetBillingHubServiceFreeHourBalanceArgs args) {
return getBillingHubServiceFreeHourBalance(args, InvokeOptions.Empty);
}
/**
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getBillingHubServiceFreeHourBalancePlain(GetBillingHubServiceFreeHourBalancePlainArgs args) {
return getBillingHubServiceFreeHourBalancePlain(args, InvokeOptions.Empty);
}
/**
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getBillingHubServiceFreeHourBalance(GetBillingHubServiceFreeHourBalanceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getBillingHubServiceFreeHourBalance", TypeShape.of(GetBillingHubServiceFreeHourBalanceResult.class), args, Utilities.withVersion(options));
}
/**
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getBillingHubServiceFreeHourBalancePlain(GetBillingHubServiceFreeHourBalancePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getBillingHubServiceFreeHourBalance", TypeShape.of(GetBillingHubServiceFreeHourBalanceResult.class), args, Utilities.withVersion(options));
}
/**
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getBillingHubServiceUsage(GetBillingHubServiceUsageArgs args) {
return getBillingHubServiceUsage(args, InvokeOptions.Empty);
}
/**
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getBillingHubServiceUsagePlain(GetBillingHubServiceUsagePlainArgs args) {
return getBillingHubServiceUsagePlain(args, InvokeOptions.Empty);
}
/**
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getBillingHubServiceUsage(GetBillingHubServiceUsageArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getBillingHubServiceUsage", TypeShape.of(GetBillingHubServiceUsageResult.class), args, Utilities.withVersion(options));
}
/**
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getBillingHubServiceUsagePlain(GetBillingHubServiceUsagePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getBillingHubServiceUsage", TypeShape.of(GetBillingHubServiceUsageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a test base credential Resource
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getCredential(GetCredentialArgs args) {
return getCredential(args, InvokeOptions.Empty);
}
/**
* Gets a test base credential Resource
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getCredentialPlain(GetCredentialPlainArgs args) {
return getCredentialPlain(args, InvokeOptions.Empty);
}
/**
* Gets a test base credential Resource
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getCredential(GetCredentialArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getCredential", TypeShape.of(GetCredentialResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a test base credential Resource
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getCredentialPlain(GetCredentialPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getCredential", TypeShape.of(GetCredentialResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a test base custom image.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getCustomImage(GetCustomImageArgs args) {
return getCustomImage(args, InvokeOptions.Empty);
}
/**
* Gets a test base custom image.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getCustomImagePlain(GetCustomImagePlainArgs args) {
return getCustomImagePlain(args, InvokeOptions.Empty);
}
/**
* Gets a test base custom image.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getCustomImage(GetCustomImageArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getCustomImage", TypeShape.of(GetCustomImageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a test base custom image.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getCustomImagePlain(GetCustomImagePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getCustomImage", TypeShape.of(GetCustomImageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Test Base CustomerEvent.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getCustomerEvent(GetCustomerEventArgs args) {
return getCustomerEvent(args, InvokeOptions.Empty);
}
/**
* Gets a Test Base CustomerEvent.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getCustomerEventPlain(GetCustomerEventPlainArgs args) {
return getCustomerEventPlain(args, InvokeOptions.Empty);
}
/**
* Gets a Test Base CustomerEvent.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getCustomerEvent(GetCustomerEventArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getCustomerEvent", TypeShape.of(GetCustomerEventResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Test Base CustomerEvent.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getCustomerEventPlain(GetCustomerEventPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getCustomerEvent", TypeShape.of(GetCustomerEventResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Test Base Draft Package.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getDraftPackage(GetDraftPackageArgs args) {
return getDraftPackage(args, InvokeOptions.Empty);
}
/**
* Gets a Test Base Draft Package.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getDraftPackagePlain(GetDraftPackagePlainArgs args) {
return getDraftPackagePlain(args, InvokeOptions.Empty);
}
/**
* Gets a Test Base Draft Package.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getDraftPackage(GetDraftPackageArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getDraftPackage", TypeShape.of(GetDraftPackageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Test Base Draft Package.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getDraftPackagePlain(GetDraftPackagePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getDraftPackage", TypeShape.of(GetDraftPackageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets draft package path and temp working path with SAS.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getDraftPackagePath(GetDraftPackagePathArgs args) {
return getDraftPackagePath(args, InvokeOptions.Empty);
}
/**
* Gets draft package path and temp working path with SAS.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getDraftPackagePathPlain(GetDraftPackagePathPlainArgs args) {
return getDraftPackagePathPlain(args, InvokeOptions.Empty);
}
/**
* Gets draft package path and temp working path with SAS.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getDraftPackagePath(GetDraftPackagePathArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getDraftPackagePath", TypeShape.of(GetDraftPackagePathResult.class), args, Utilities.withVersion(options));
}
/**
* Gets draft package path and temp working path with SAS.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getDraftPackagePathPlain(GetDraftPackagePathPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getDraftPackagePath", TypeShape.of(GetDraftPackagePathResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a favorite process for a Test Base Package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getFavoriteProcess(GetFavoriteProcessArgs args) {
return getFavoriteProcess(args, InvokeOptions.Empty);
}
/**
* Gets a favorite process for a Test Base Package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getFavoriteProcessPlain(GetFavoriteProcessPlainArgs args) {
return getFavoriteProcessPlain(args, InvokeOptions.Empty);
}
/**
* Gets a favorite process for a Test Base Package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getFavoriteProcess(GetFavoriteProcessArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getFavoriteProcess", TypeShape.of(GetFavoriteProcessResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a favorite process for a Test Base Package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getFavoriteProcessPlain(GetFavoriteProcessPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getFavoriteProcess", TypeShape.of(GetFavoriteProcessResult.class), args, Utilities.withVersion(options));
}
/**
* Get image properties under the image definition name created by test base custom image which derived from 'VHD' source.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getImageDefinition(GetImageDefinitionArgs args) {
return getImageDefinition(args, InvokeOptions.Empty);
}
/**
* Get image properties under the image definition name created by test base custom image which derived from 'VHD' source.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getImageDefinitionPlain(GetImageDefinitionPlainArgs args) {
return getImageDefinitionPlain(args, InvokeOptions.Empty);
}
/**
* Get image properties under the image definition name created by test base custom image which derived from 'VHD' source.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static Output getImageDefinition(GetImageDefinitionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getImageDefinition", TypeShape.of(GetImageDefinitionResult.class), args, Utilities.withVersion(options));
}
/**
* Get image properties under the image definition name created by test base custom image which derived from 'VHD' source.
* Azure REST API version: 2023-11-01-preview.
*
*/
public static CompletableFuture getImageDefinitionPlain(GetImageDefinitionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getImageDefinition", TypeShape.of(GetImageDefinitionResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Test Base Package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getPackage(GetPackageArgs args) {
return getPackage(args, InvokeOptions.Empty);
}
/**
* Gets a Test Base Package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getPackagePlain(GetPackagePlainArgs args) {
return getPackagePlain(args, InvokeOptions.Empty);
}
/**
* Gets a Test Base Package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getPackage(GetPackageArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getPackage", TypeShape.of(GetPackageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Test Base Package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getPackagePlain(GetPackagePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getPackage", TypeShape.of(GetPackageResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the download URL of a package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getPackageDownloadURL(GetPackageDownloadURLArgs args) {
return getPackageDownloadURL(args, InvokeOptions.Empty);
}
/**
* Gets the download URL of a package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getPackageDownloadURLPlain(GetPackageDownloadURLPlainArgs args) {
return getPackageDownloadURLPlain(args, InvokeOptions.Empty);
}
/**
* Gets the download URL of a package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getPackageDownloadURL(GetPackageDownloadURLArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getPackageDownloadURL", TypeShape.of(GetPackageDownloadURLResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the download URL of a package.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getPackageDownloadURLPlain(GetPackageDownloadURLPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getPackageDownloadURL", TypeShape.of(GetPackageDownloadURLResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Test Base Account.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getTestBaseAccount(GetTestBaseAccountArgs args) {
return getTestBaseAccount(args, InvokeOptions.Empty);
}
/**
* Gets a Test Base Account.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getTestBaseAccountPlain(GetTestBaseAccountPlainArgs args) {
return getTestBaseAccountPlain(args, InvokeOptions.Empty);
}
/**
* Gets a Test Base Account.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getTestBaseAccount(GetTestBaseAccountArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getTestBaseAccount", TypeShape.of(GetTestBaseAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Gets a Test Base Account.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getTestBaseAccountPlain(GetTestBaseAccountPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getTestBaseAccount", TypeShape.of(GetTestBaseAccountResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the file upload URL of a Test Base Account.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getTestBaseAccountFileUploadUrl(GetTestBaseAccountFileUploadUrlArgs args) {
return getTestBaseAccountFileUploadUrl(args, InvokeOptions.Empty);
}
/**
* Gets the file upload URL of a Test Base Account.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getTestBaseAccountFileUploadUrlPlain(GetTestBaseAccountFileUploadUrlPlainArgs args) {
return getTestBaseAccountFileUploadUrlPlain(args, InvokeOptions.Empty);
}
/**
* Gets the file upload URL of a Test Base Account.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getTestBaseAccountFileUploadUrl(GetTestBaseAccountFileUploadUrlArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getTestBaseAccountFileUploadUrl", TypeShape.of(GetTestBaseAccountFileUploadUrlResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the file upload URL of a Test Base Account.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getTestBaseAccountFileUploadUrlPlain(GetTestBaseAccountFileUploadUrlPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getTestBaseAccountFileUploadUrl", TypeShape.of(GetTestBaseAccountFileUploadUrlResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the download URL of the test execution console log file.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getTestResultConsoleLogDownloadURL(GetTestResultConsoleLogDownloadURLArgs args) {
return getTestResultConsoleLogDownloadURL(args, InvokeOptions.Empty);
}
/**
* Gets the download URL of the test execution console log file.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getTestResultConsoleLogDownloadURLPlain(GetTestResultConsoleLogDownloadURLPlainArgs args) {
return getTestResultConsoleLogDownloadURLPlain(args, InvokeOptions.Empty);
}
/**
* Gets the download URL of the test execution console log file.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getTestResultConsoleLogDownloadURL(GetTestResultConsoleLogDownloadURLArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getTestResultConsoleLogDownloadURL", TypeShape.of(GetTestResultConsoleLogDownloadURLResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the download URL of the test execution console log file.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getTestResultConsoleLogDownloadURLPlain(GetTestResultConsoleLogDownloadURLPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getTestResultConsoleLogDownloadURL", TypeShape.of(GetTestResultConsoleLogDownloadURLResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the download URL of the test result.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getTestResultDownloadURL(GetTestResultDownloadURLArgs args) {
return getTestResultDownloadURL(args, InvokeOptions.Empty);
}
/**
* Gets the download URL of the test result.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getTestResultDownloadURLPlain(GetTestResultDownloadURLPlainArgs args) {
return getTestResultDownloadURLPlain(args, InvokeOptions.Empty);
}
/**
* Gets the download URL of the test result.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getTestResultDownloadURL(GetTestResultDownloadURLArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getTestResultDownloadURL", TypeShape.of(GetTestResultDownloadURLResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the download URL of the test result.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getTestResultDownloadURLPlain(GetTestResultDownloadURLPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getTestResultDownloadURL", TypeShape.of(GetTestResultDownloadURLResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the download URL of the test execution screen recording.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getTestResultVideoDownloadURL(GetTestResultVideoDownloadURLArgs args) {
return getTestResultVideoDownloadURL(args, InvokeOptions.Empty);
}
/**
* Gets the download URL of the test execution screen recording.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getTestResultVideoDownloadURLPlain(GetTestResultVideoDownloadURLPlainArgs args) {
return getTestResultVideoDownloadURLPlain(args, InvokeOptions.Empty);
}
/**
* Gets the download URL of the test execution screen recording.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static Output getTestResultVideoDownloadURL(GetTestResultVideoDownloadURLArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:testbase:getTestResultVideoDownloadURL", TypeShape.of(GetTestResultVideoDownloadURLResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the download URL of the test execution screen recording.
* Azure REST API version: 2022-04-01-preview.
*
* Other available API versions: 2023-11-01-preview.
*
*/
public static CompletableFuture getTestResultVideoDownloadURLPlain(GetTestResultVideoDownloadURLPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:testbase:getTestResultVideoDownloadURL", TypeShape.of(GetTestResultVideoDownloadURLResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy