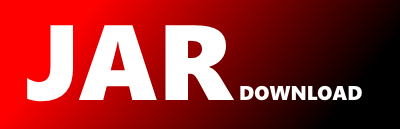
com.pulumi.azurenative.timeseriesinsights.IoTHubEventSource Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.timeseriesinsights;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.timeseriesinsights.IoTHubEventSourceArgs;
import com.pulumi.azurenative.timeseriesinsights.outputs.LocalTimestampResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* An event source that receives its data from an Azure IoTHub.
* Azure REST API version: 2020-05-15. Prior API version in Azure Native 1.x: 2020-05-15.
*
* ## Example Usage
* ### CreateEventHubEventSource
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.timeseriesinsights.IoTHubEventSource;
* import com.pulumi.azurenative.timeseriesinsights.IoTHubEventSourceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var ioTHubEventSource = new IoTHubEventSource("ioTHubEventSource", IoTHubEventSourceArgs.builder()
* .environmentName("env1")
* .eventSourceName("es1")
* .resourceGroupName("rg1")
* .build());
*
* }
* }
*
* }
*
* ### EventSourcesCreateEventHubWithCustomEnquedTime
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.timeseriesinsights.IoTHubEventSource;
* import com.pulumi.azurenative.timeseriesinsights.IoTHubEventSourceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var ioTHubEventSource = new IoTHubEventSource("ioTHubEventSource", IoTHubEventSourceArgs.builder()
* .environmentName("env1")
* .eventSourceName("es1")
* .resourceGroupName("rg1")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:timeseriesinsights:IoTHubEventSource es1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.TimeSeriesInsights/environments/{environmentName}/eventSources/{eventSourceName}
* ```
*
*/
@ResourceType(type="azure-native:timeseriesinsights:IoTHubEventSource")
public class IoTHubEventSource extends com.pulumi.resources.CustomResource {
/**
* The name of the iot hub's consumer group that holds the partitions from which events will be read.
*
*/
@Export(name="consumerGroupName", refs={String.class}, tree="[0]")
private Output consumerGroupName;
/**
* @return The name of the iot hub's consumer group that holds the partitions from which events will be read.
*
*/
public Output consumerGroupName() {
return this.consumerGroupName;
}
/**
* The time the resource was created.
*
*/
@Export(name="creationTime", refs={String.class}, tree="[0]")
private Output creationTime;
/**
* @return The time the resource was created.
*
*/
public Output creationTime() {
return this.creationTime;
}
/**
* The resource id of the event source in Azure Resource Manager.
*
*/
@Export(name="eventSourceResourceId", refs={String.class}, tree="[0]")
private Output eventSourceResourceId;
/**
* @return The resource id of the event source in Azure Resource Manager.
*
*/
public Output eventSourceResourceId() {
return this.eventSourceResourceId;
}
/**
* The name of the iot hub.
*
*/
@Export(name="iotHubName", refs={String.class}, tree="[0]")
private Output iotHubName;
/**
* @return The name of the iot hub.
*
*/
public Output iotHubName() {
return this.iotHubName;
}
/**
* The name of the Shared Access Policy key that grants the Time Series Insights service access to the iot hub. This shared access policy key must grant 'service connect' permissions to the iot hub.
*
*/
@Export(name="keyName", refs={String.class}, tree="[0]")
private Output keyName;
/**
* @return The name of the Shared Access Policy key that grants the Time Series Insights service access to the iot hub. This shared access policy key must grant 'service connect' permissions to the iot hub.
*
*/
public Output keyName() {
return this.keyName;
}
/**
* The kind of the event source.
* Expected value is 'Microsoft.IoTHub'.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return The kind of the event source.
* Expected value is 'Microsoft.IoTHub'.
*
*/
public Output kind() {
return this.kind;
}
/**
* An object that represents the local timestamp property. It contains the format of local timestamp that needs to be used and the corresponding timezone offset information. If a value isn't specified for localTimestamp, or if null, then the local timestamp will not be ingressed with the events.
*
*/
@Export(name="localTimestamp", refs={LocalTimestampResponse.class}, tree="[0]")
private Output* @Nullable */ LocalTimestampResponse> localTimestamp;
/**
* @return An object that represents the local timestamp property. It contains the format of local timestamp that needs to be used and the corresponding timezone offset information. If a value isn't specified for localTimestamp, or if null, then the local timestamp will not be ingressed with the events.
*
*/
public Output> localTimestamp() {
return Codegen.optional(this.localTimestamp);
}
/**
* Resource location
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Resource location
*
*/
public Output location() {
return this.location;
}
/**
* Resource name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name
*
*/
public Output name() {
return this.name;
}
/**
* Provisioning state of the resource.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Provisioning state of the resource.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Resource tags
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* ISO8601 UTC datetime with seconds precision (milliseconds are optional), specifying the date and time that will be the starting point for Events to be consumed.
*
*/
@Export(name="time", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> time;
/**
* @return ISO8601 UTC datetime with seconds precision (milliseconds are optional), specifying the date and time that will be the starting point for Events to be consumed.
*
*/
public Output> time() {
return Codegen.optional(this.time);
}
/**
* The event property that will be used as the event source's timestamp. If a value isn't specified for timestampPropertyName, or if null or empty-string is specified, the event creation time will be used.
*
*/
@Export(name="timestampPropertyName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> timestampPropertyName;
/**
* @return The event property that will be used as the event source's timestamp. If a value isn't specified for timestampPropertyName, or if null or empty-string is specified, the event creation time will be used.
*
*/
public Output> timestampPropertyName() {
return Codegen.optional(this.timestampPropertyName);
}
/**
* Resource type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public IoTHubEventSource(java.lang.String name) {
this(name, IoTHubEventSourceArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public IoTHubEventSource(java.lang.String name, IoTHubEventSourceArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public IoTHubEventSource(java.lang.String name, IoTHubEventSourceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:timeseriesinsights:IoTHubEventSource", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private IoTHubEventSource(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:timeseriesinsights:IoTHubEventSource", name, null, makeResourceOptions(options, id), false);
}
private static IoTHubEventSourceArgs makeArgs(IoTHubEventSourceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
var builder = args == null ? IoTHubEventSourceArgs.builder() : IoTHubEventSourceArgs.builder(args);
return builder
.kind("Microsoft.IoTHub")
.build();
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20170228preview:IoTHubEventSource").build()),
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20171115:IoTHubEventSource").build()),
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20180815preview:IoTHubEventSource").build()),
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20200515:IoTHubEventSource").build()),
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20210331preview:IoTHubEventSource").build()),
Output.of(Alias.builder().type("azure-native:timeseriesinsights/v20210630preview:IoTHubEventSource").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static IoTHubEventSource get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new IoTHubEventSource(name, id, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy