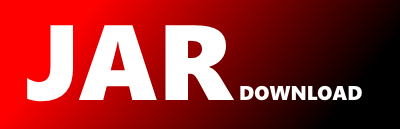
com.pulumi.azurenative.timeseriesinsights.ReferenceDataSetArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.timeseriesinsights;
import com.pulumi.azurenative.timeseriesinsights.enums.DataStringComparisonBehavior;
import com.pulumi.azurenative.timeseriesinsights.inputs.ReferenceDataSetKeyPropertyArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ReferenceDataSetArgs extends com.pulumi.resources.ResourceArgs {
public static final ReferenceDataSetArgs Empty = new ReferenceDataSetArgs();
/**
* The reference data set key comparison behavior can be set using this property. By default, the value is 'Ordinal' - which means case sensitive key comparison will be performed while joining reference data with events or while adding new reference data. When 'OrdinalIgnoreCase' is set, case insensitive comparison will be used.
*
*/
@Import(name="dataStringComparisonBehavior")
private @Nullable Output> dataStringComparisonBehavior;
/**
* @return The reference data set key comparison behavior can be set using this property. By default, the value is 'Ordinal' - which means case sensitive key comparison will be performed while joining reference data with events or while adding new reference data. When 'OrdinalIgnoreCase' is set, case insensitive comparison will be used.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy