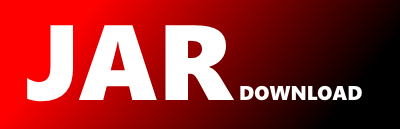
com.pulumi.azurenative.timeseriesinsights.outputs.GetGen2EnvironmentResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.timeseriesinsights.outputs;
import com.pulumi.azurenative.timeseriesinsights.outputs.EnvironmentStatusResponse;
import com.pulumi.azurenative.timeseriesinsights.outputs.Gen2StorageConfigurationOutputResponse;
import com.pulumi.azurenative.timeseriesinsights.outputs.SkuResponse;
import com.pulumi.azurenative.timeseriesinsights.outputs.TimeSeriesIdPropertyResponse;
import com.pulumi.azurenative.timeseriesinsights.outputs.WarmStoreConfigurationPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetGen2EnvironmentResult {
/**
* @return The time the resource was created.
*
*/
private String creationTime;
/**
* @return The fully qualified domain name used to access the environment data, e.g. to query the environment's events or upload reference data for the environment.
*
*/
private String dataAccessFqdn;
/**
* @return An id used to access the environment data, e.g. to query the environment's events or upload reference data for the environment.
*
*/
private String dataAccessId;
/**
* @return Resource Id
*
*/
private String id;
/**
* @return The kind of the environment.
* Expected value is 'Gen2'.
*
*/
private String kind;
/**
* @return Resource location
*
*/
private String location;
/**
* @return Resource name
*
*/
private String name;
/**
* @return Provisioning state of the resource.
*
*/
private String provisioningState;
/**
* @return The sku determines the type of environment, either Gen1 (S1 or S2) or Gen2 (L1). For Gen1 environments the sku determines the capacity of the environment, the ingress rate, and the billing rate.
*
*/
private SkuResponse sku;
/**
* @return An object that represents the status of the environment, and its internal state in the Time Series Insights service.
*
*/
private EnvironmentStatusResponse status;
/**
* @return The storage configuration provides the connection details that allows the Time Series Insights service to connect to the customer storage account that is used to store the environment's data.
*
*/
private Gen2StorageConfigurationOutputResponse storageConfiguration;
/**
* @return Resource tags
*
*/
private @Nullable Map tags;
/**
* @return The list of event properties which will be used to define the environment's time series id.
*
*/
private List timeSeriesIdProperties;
/**
* @return Resource type
*
*/
private String type;
/**
* @return The warm store configuration provides the details to create a warm store cache that will retain a copy of the environment's data available for faster query.
*
*/
private @Nullable WarmStoreConfigurationPropertiesResponse warmStoreConfiguration;
private GetGen2EnvironmentResult() {}
/**
* @return The time the resource was created.
*
*/
public String creationTime() {
return this.creationTime;
}
/**
* @return The fully qualified domain name used to access the environment data, e.g. to query the environment's events or upload reference data for the environment.
*
*/
public String dataAccessFqdn() {
return this.dataAccessFqdn;
}
/**
* @return An id used to access the environment data, e.g. to query the environment's events or upload reference data for the environment.
*
*/
public String dataAccessId() {
return this.dataAccessId;
}
/**
* @return Resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return The kind of the environment.
* Expected value is 'Gen2'.
*
*/
public String kind() {
return this.kind;
}
/**
* @return Resource location
*
*/
public String location() {
return this.location;
}
/**
* @return Resource name
*
*/
public String name() {
return this.name;
}
/**
* @return Provisioning state of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The sku determines the type of environment, either Gen1 (S1 or S2) or Gen2 (L1). For Gen1 environments the sku determines the capacity of the environment, the ingress rate, and the billing rate.
*
*/
public SkuResponse sku() {
return this.sku;
}
/**
* @return An object that represents the status of the environment, and its internal state in the Time Series Insights service.
*
*/
public EnvironmentStatusResponse status() {
return this.status;
}
/**
* @return The storage configuration provides the connection details that allows the Time Series Insights service to connect to the customer storage account that is used to store the environment's data.
*
*/
public Gen2StorageConfigurationOutputResponse storageConfiguration() {
return this.storageConfiguration;
}
/**
* @return Resource tags
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The list of event properties which will be used to define the environment's time series id.
*
*/
public List timeSeriesIdProperties() {
return this.timeSeriesIdProperties;
}
/**
* @return Resource type
*
*/
public String type() {
return this.type;
}
/**
* @return The warm store configuration provides the details to create a warm store cache that will retain a copy of the environment's data available for faster query.
*
*/
public Optional warmStoreConfiguration() {
return Optional.ofNullable(this.warmStoreConfiguration);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetGen2EnvironmentResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String creationTime;
private String dataAccessFqdn;
private String dataAccessId;
private String id;
private String kind;
private String location;
private String name;
private String provisioningState;
private SkuResponse sku;
private EnvironmentStatusResponse status;
private Gen2StorageConfigurationOutputResponse storageConfiguration;
private @Nullable Map tags;
private List timeSeriesIdProperties;
private String type;
private @Nullable WarmStoreConfigurationPropertiesResponse warmStoreConfiguration;
public Builder() {}
public Builder(GetGen2EnvironmentResult defaults) {
Objects.requireNonNull(defaults);
this.creationTime = defaults.creationTime;
this.dataAccessFqdn = defaults.dataAccessFqdn;
this.dataAccessId = defaults.dataAccessId;
this.id = defaults.id;
this.kind = defaults.kind;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.sku = defaults.sku;
this.status = defaults.status;
this.storageConfiguration = defaults.storageConfiguration;
this.tags = defaults.tags;
this.timeSeriesIdProperties = defaults.timeSeriesIdProperties;
this.type = defaults.type;
this.warmStoreConfiguration = defaults.warmStoreConfiguration;
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder dataAccessFqdn(String dataAccessFqdn) {
if (dataAccessFqdn == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "dataAccessFqdn");
}
this.dataAccessFqdn = dataAccessFqdn;
return this;
}
@CustomType.Setter
public Builder dataAccessId(String dataAccessId) {
if (dataAccessId == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "dataAccessId");
}
this.dataAccessId = dataAccessId;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder sku(SkuResponse sku) {
if (sku == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "sku");
}
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder status(EnvironmentStatusResponse status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder storageConfiguration(Gen2StorageConfigurationOutputResponse storageConfiguration) {
if (storageConfiguration == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "storageConfiguration");
}
this.storageConfiguration = storageConfiguration;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder timeSeriesIdProperties(List timeSeriesIdProperties) {
if (timeSeriesIdProperties == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "timeSeriesIdProperties");
}
this.timeSeriesIdProperties = timeSeriesIdProperties;
return this;
}
public Builder timeSeriesIdProperties(TimeSeriesIdPropertyResponse... timeSeriesIdProperties) {
return timeSeriesIdProperties(List.of(timeSeriesIdProperties));
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetGen2EnvironmentResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder warmStoreConfiguration(@Nullable WarmStoreConfigurationPropertiesResponse warmStoreConfiguration) {
this.warmStoreConfiguration = warmStoreConfiguration;
return this;
}
public GetGen2EnvironmentResult build() {
final var _resultValue = new GetGen2EnvironmentResult();
_resultValue.creationTime = creationTime;
_resultValue.dataAccessFqdn = dataAccessFqdn;
_resultValue.dataAccessId = dataAccessId;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.sku = sku;
_resultValue.status = status;
_resultValue.storageConfiguration = storageConfiguration;
_resultValue.tags = tags;
_resultValue.timeSeriesIdProperties = timeSeriesIdProperties;
_resultValue.type = type;
_resultValue.warmStoreConfiguration = warmStoreConfiguration;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy