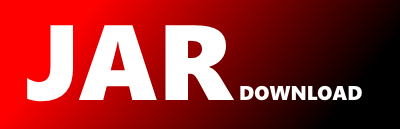
com.pulumi.azurenative.timeseriesinsights.outputs.GetReferenceDataSetResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.timeseriesinsights.outputs;
import com.pulumi.azurenative.timeseriesinsights.outputs.ReferenceDataSetKeyPropertyResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetReferenceDataSetResult {
/**
* @return The time the resource was created.
*
*/
private String creationTime;
/**
* @return The reference data set key comparison behavior can be set using this property. By default, the value is 'Ordinal' - which means case sensitive key comparison will be performed while joining reference data with events or while adding new reference data. When 'OrdinalIgnoreCase' is set, case insensitive comparison will be used.
*
*/
private @Nullable String dataStringComparisonBehavior;
/**
* @return Resource Id
*
*/
private String id;
/**
* @return The list of key properties for the reference data set.
*
*/
private List keyProperties;
/**
* @return Resource location
*
*/
private String location;
/**
* @return Resource name
*
*/
private String name;
/**
* @return Provisioning state of the resource.
*
*/
private String provisioningState;
/**
* @return Resource tags
*
*/
private @Nullable Map tags;
/**
* @return Resource type
*
*/
private String type;
private GetReferenceDataSetResult() {}
/**
* @return The time the resource was created.
*
*/
public String creationTime() {
return this.creationTime;
}
/**
* @return The reference data set key comparison behavior can be set using this property. By default, the value is 'Ordinal' - which means case sensitive key comparison will be performed while joining reference data with events or while adding new reference data. When 'OrdinalIgnoreCase' is set, case insensitive comparison will be used.
*
*/
public Optional dataStringComparisonBehavior() {
return Optional.ofNullable(this.dataStringComparisonBehavior);
}
/**
* @return Resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return The list of key properties for the reference data set.
*
*/
public List keyProperties() {
return this.keyProperties;
}
/**
* @return Resource location
*
*/
public String location() {
return this.location;
}
/**
* @return Resource name
*
*/
public String name() {
return this.name;
}
/**
* @return Provisioning state of the resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Resource tags
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Resource type
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetReferenceDataSetResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String creationTime;
private @Nullable String dataStringComparisonBehavior;
private String id;
private List keyProperties;
private String location;
private String name;
private String provisioningState;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetReferenceDataSetResult defaults) {
Objects.requireNonNull(defaults);
this.creationTime = defaults.creationTime;
this.dataStringComparisonBehavior = defaults.dataStringComparisonBehavior;
this.id = defaults.id;
this.keyProperties = defaults.keyProperties;
this.location = defaults.location;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetReferenceDataSetResult", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder dataStringComparisonBehavior(@Nullable String dataStringComparisonBehavior) {
this.dataStringComparisonBehavior = dataStringComparisonBehavior;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetReferenceDataSetResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder keyProperties(List keyProperties) {
if (keyProperties == null) {
throw new MissingRequiredPropertyException("GetReferenceDataSetResult", "keyProperties");
}
this.keyProperties = keyProperties;
return this;
}
public Builder keyProperties(ReferenceDataSetKeyPropertyResponse... keyProperties) {
return keyProperties(List.of(keyProperties));
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetReferenceDataSetResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetReferenceDataSetResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetReferenceDataSetResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetReferenceDataSetResult", "type");
}
this.type = type;
return this;
}
public GetReferenceDataSetResult build() {
final var _resultValue = new GetReferenceDataSetResult();
_resultValue.creationTime = creationTime;
_resultValue.dataStringComparisonBehavior = dataStringComparisonBehavior;
_resultValue.id = id;
_resultValue.keyProperties = keyProperties;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy