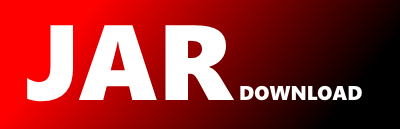
com.pulumi.azurenative.videoanalyzer.inputs.ParameterDeclarationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer.inputs;
import com.pulumi.azurenative.videoanalyzer.enums.ParameterType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Single topology parameter declaration. Declared parameters can and must be referenced throughout the topology and can optionally have default values to be used when they are not defined in the pipelines.
*
*/
public final class ParameterDeclarationArgs extends com.pulumi.resources.ResourceArgs {
public static final ParameterDeclarationArgs Empty = new ParameterDeclarationArgs();
/**
* The default value for the parameter to be used if the pipeline does not specify a value.
*
*/
@Import(name="default")
private @Nullable Output default_;
/**
* @return The default value for the parameter to be used if the pipeline does not specify a value.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy