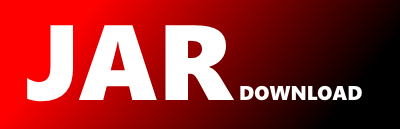
com.pulumi.azurenative.videoanalyzer.inputs.VideoEncoderH264Args Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer.inputs;
import com.pulumi.azurenative.videoanalyzer.inputs.VideoScaleArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A custom preset for encoding video with the H.264 (AVC) codec.
*
*/
public final class VideoEncoderH264Args extends com.pulumi.resources.ResourceArgs {
public static final VideoEncoderH264Args Empty = new VideoEncoderH264Args();
/**
* The maximum bitrate, in kilobits per second or Kbps, at which video should be encoded. If omitted, encoder sets it automatically to try and match the quality of the input video.
*
*/
@Import(name="bitrateKbps")
private @Nullable Output bitrateKbps;
/**
* @return The maximum bitrate, in kilobits per second or Kbps, at which video should be encoded. If omitted, encoder sets it automatically to try and match the quality of the input video.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy