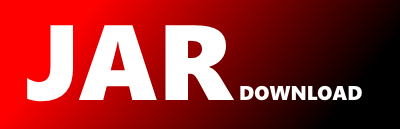
com.pulumi.azurenative.videoanalyzer.outputs.EccTokenKeyResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class EccTokenKeyResponse {
/**
* @return Elliptical curve algorithm to be used: ES256, ES384 or ES512.
*
*/
private String alg;
/**
* @return JWT token key id. Validation keys are looked up based on the key id present on the JWT token header.
*
*/
private String kid;
/**
* @return The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.EccTokenKey'.
*
*/
private String type;
/**
* @return X coordinate.
*
*/
private String x;
/**
* @return Y coordinate.
*
*/
private String y;
private EccTokenKeyResponse() {}
/**
* @return Elliptical curve algorithm to be used: ES256, ES384 or ES512.
*
*/
public String alg() {
return this.alg;
}
/**
* @return JWT token key id. Validation keys are looked up based on the key id present on the JWT token header.
*
*/
public String kid() {
return this.kid;
}
/**
* @return The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.EccTokenKey'.
*
*/
public String type() {
return this.type;
}
/**
* @return X coordinate.
*
*/
public String x() {
return this.x;
}
/**
* @return Y coordinate.
*
*/
public String y() {
return this.y;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EccTokenKeyResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String alg;
private String kid;
private String type;
private String x;
private String y;
public Builder() {}
public Builder(EccTokenKeyResponse defaults) {
Objects.requireNonNull(defaults);
this.alg = defaults.alg;
this.kid = defaults.kid;
this.type = defaults.type;
this.x = defaults.x;
this.y = defaults.y;
}
@CustomType.Setter
public Builder alg(String alg) {
if (alg == null) {
throw new MissingRequiredPropertyException("EccTokenKeyResponse", "alg");
}
this.alg = alg;
return this;
}
@CustomType.Setter
public Builder kid(String kid) {
if (kid == null) {
throw new MissingRequiredPropertyException("EccTokenKeyResponse", "kid");
}
this.kid = kid;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("EccTokenKeyResponse", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder x(String x) {
if (x == null) {
throw new MissingRequiredPropertyException("EccTokenKeyResponse", "x");
}
this.x = x;
return this;
}
@CustomType.Setter
public Builder y(String y) {
if (y == null) {
throw new MissingRequiredPropertyException("EccTokenKeyResponse", "y");
}
this.y = y;
return this;
}
public EccTokenKeyResponse build() {
final var _resultValue = new EccTokenKeyResponse();
_resultValue.alg = alg;
_resultValue.kid = kid;
_resultValue.type = type;
_resultValue.x = x;
_resultValue.y = y;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy