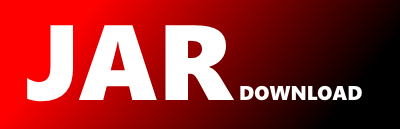
com.pulumi.azurenative.videoanalyzer.outputs.GetVideoResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer.outputs;
import com.pulumi.azurenative.videoanalyzer.outputs.SystemDataResponse;
import com.pulumi.azurenative.videoanalyzer.outputs.VideoArchivalResponse;
import com.pulumi.azurenative.videoanalyzer.outputs.VideoContentUrlsResponse;
import com.pulumi.azurenative.videoanalyzer.outputs.VideoFlagsResponse;
import com.pulumi.azurenative.videoanalyzer.outputs.VideoMediaInfoResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetVideoResult {
/**
* @return Video archival properties.
*
*/
private @Nullable VideoArchivalResponse archival;
/**
* @return Set of URLs to the video content.
*
*/
private VideoContentUrlsResponse contentUrls;
/**
* @return Optional video description provided by the user. Value can be up to 2048 characters long.
*
*/
private @Nullable String description;
/**
* @return Video flags contain information about the available video actions and its dynamic properties based on the current video state.
*
*/
private VideoFlagsResponse flags;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Contains information about the video and audio content.
*
*/
private @Nullable VideoMediaInfoResponse mediaInfo;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Optional video title provided by the user. Value can be up to 256 characters long.
*
*/
private @Nullable String title;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetVideoResult() {}
/**
* @return Video archival properties.
*
*/
public Optional archival() {
return Optional.ofNullable(this.archival);
}
/**
* @return Set of URLs to the video content.
*
*/
public VideoContentUrlsResponse contentUrls() {
return this.contentUrls;
}
/**
* @return Optional video description provided by the user. Value can be up to 2048 characters long.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return Video flags contain information about the available video actions and its dynamic properties based on the current video state.
*
*/
public VideoFlagsResponse flags() {
return this.flags;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Contains information about the video and audio content.
*
*/
public Optional mediaInfo() {
return Optional.ofNullable(this.mediaInfo);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Optional video title provided by the user. Value can be up to 256 characters long.
*
*/
public Optional title() {
return Optional.ofNullable(this.title);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetVideoResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable VideoArchivalResponse archival;
private VideoContentUrlsResponse contentUrls;
private @Nullable String description;
private VideoFlagsResponse flags;
private String id;
private @Nullable VideoMediaInfoResponse mediaInfo;
private String name;
private SystemDataResponse systemData;
private @Nullable String title;
private String type;
public Builder() {}
public Builder(GetVideoResult defaults) {
Objects.requireNonNull(defaults);
this.archival = defaults.archival;
this.contentUrls = defaults.contentUrls;
this.description = defaults.description;
this.flags = defaults.flags;
this.id = defaults.id;
this.mediaInfo = defaults.mediaInfo;
this.name = defaults.name;
this.systemData = defaults.systemData;
this.title = defaults.title;
this.type = defaults.type;
}
@CustomType.Setter
public Builder archival(@Nullable VideoArchivalResponse archival) {
this.archival = archival;
return this;
}
@CustomType.Setter
public Builder contentUrls(VideoContentUrlsResponse contentUrls) {
if (contentUrls == null) {
throw new MissingRequiredPropertyException("GetVideoResult", "contentUrls");
}
this.contentUrls = contentUrls;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder flags(VideoFlagsResponse flags) {
if (flags == null) {
throw new MissingRequiredPropertyException("GetVideoResult", "flags");
}
this.flags = flags;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetVideoResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder mediaInfo(@Nullable VideoMediaInfoResponse mediaInfo) {
this.mediaInfo = mediaInfo;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetVideoResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetVideoResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder title(@Nullable String title) {
this.title = title;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetVideoResult", "type");
}
this.type = type;
return this;
}
public GetVideoResult build() {
final var _resultValue = new GetVideoResult();
_resultValue.archival = archival;
_resultValue.contentUrls = contentUrls;
_resultValue.description = description;
_resultValue.flags = flags;
_resultValue.id = id;
_resultValue.mediaInfo = mediaInfo;
_resultValue.name = name;
_resultValue.systemData = systemData;
_resultValue.title = title;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy