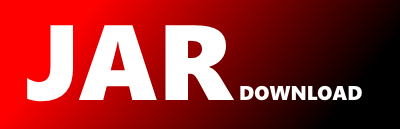
com.pulumi.azurenative.web.AppServiceEnvironment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.web.AppServiceEnvironmentArgs;
import com.pulumi.azurenative.web.outputs.AseV3NetworkingConfigurationResponse;
import com.pulumi.azurenative.web.outputs.CustomDnsSuffixConfigurationResponse;
import com.pulumi.azurenative.web.outputs.NameValuePairResponse;
import com.pulumi.azurenative.web.outputs.VirtualNetworkProfileResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* App Service Environment ARM resource.
* Azure REST API version: 2022-09-01. Prior API version in Azure Native 1.x: 2020-12-01.
*
* Other available API versions: 2019-08-01, 2020-10-01, 2021-01-15, 2023-01-01, 2023-12-01.
*
* ## Example Usage
* ### Create or update an App Service Environment.
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.web.AppServiceEnvironment;
* import com.pulumi.azurenative.web.AppServiceEnvironmentArgs;
* import com.pulumi.azurenative.web.inputs.VirtualNetworkProfileArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var appServiceEnvironment = new AppServiceEnvironment("appServiceEnvironment", AppServiceEnvironmentArgs.builder()
* .kind("Asev3")
* .location("South Central US")
* .name("test-ase")
* .resourceGroupName("test-rg")
* .virtualNetwork(VirtualNetworkProfileArgs.builder()
* .id("/subscriptions/34adfa4f-cedf-4dc0-ba29-b6d1a69ab345/resourceGroups/test-rg/providers/Microsoft.Network/virtualNetworks/test-vnet/subnets/delegated")
* .build())
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:web:AppServiceEnvironment test-ase /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Web/hostingEnvironments/{name}
* ```
*
*/
@ResourceType(type="azure-native:web:AppServiceEnvironment")
public class AppServiceEnvironment extends com.pulumi.resources.CustomResource {
/**
* Custom settings for changing the behavior of the App Service Environment.
*
*/
@Export(name="clusterSettings", refs={List.class,NameValuePairResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> clusterSettings;
/**
* @return Custom settings for changing the behavior of the App Service Environment.
*
*/
public Output>> clusterSettings() {
return Codegen.optional(this.clusterSettings);
}
/**
* Full view of the custom domain suffix configuration for ASEv3.
*
*/
@Export(name="customDnsSuffixConfiguration", refs={CustomDnsSuffixConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ CustomDnsSuffixConfigurationResponse> customDnsSuffixConfiguration;
/**
* @return Full view of the custom domain suffix configuration for ASEv3.
*
*/
public Output> customDnsSuffixConfiguration() {
return Codegen.optional(this.customDnsSuffixConfiguration);
}
/**
* Dedicated Host Count
*
*/
@Export(name="dedicatedHostCount", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> dedicatedHostCount;
/**
* @return Dedicated Host Count
*
*/
public Output> dedicatedHostCount() {
return Codegen.optional(this.dedicatedHostCount);
}
/**
* DNS suffix of the App Service Environment.
*
*/
@Export(name="dnsSuffix", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dnsSuffix;
/**
* @return DNS suffix of the App Service Environment.
*
*/
public Output> dnsSuffix() {
return Codegen.optional(this.dnsSuffix);
}
/**
* Scale factor for front-ends.
*
*/
@Export(name="frontEndScaleFactor", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> frontEndScaleFactor;
/**
* @return Scale factor for front-ends.
*
*/
public Output> frontEndScaleFactor() {
return Codegen.optional(this.frontEndScaleFactor);
}
/**
* Flag that displays whether an ASE has linux workers or not
*
*/
@Export(name="hasLinuxWorkers", refs={Boolean.class}, tree="[0]")
private Output hasLinuxWorkers;
/**
* @return Flag that displays whether an ASE has linux workers or not
*
*/
public Output hasLinuxWorkers() {
return this.hasLinuxWorkers;
}
/**
* Specifies which endpoints to serve internally in the Virtual Network for the App Service Environment.
*
*/
@Export(name="internalLoadBalancingMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> internalLoadBalancingMode;
/**
* @return Specifies which endpoints to serve internally in the Virtual Network for the App Service Environment.
*
*/
public Output> internalLoadBalancingMode() {
return Codegen.optional(this.internalLoadBalancingMode);
}
/**
* Number of IP SSL addresses reserved for the App Service Environment.
*
*/
@Export(name="ipsslAddressCount", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> ipsslAddressCount;
/**
* @return Number of IP SSL addresses reserved for the App Service Environment.
*
*/
public Output> ipsslAddressCount() {
return Codegen.optional(this.ipsslAddressCount);
}
/**
* Kind of resource.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kind;
/**
* @return Kind of resource.
*
*/
public Output> kind() {
return Codegen.optional(this.kind);
}
/**
* Resource Location.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Resource Location.
*
*/
public Output location() {
return this.location;
}
/**
* Maximum number of VMs in the App Service Environment.
*
*/
@Export(name="maximumNumberOfMachines", refs={Integer.class}, tree="[0]")
private Output maximumNumberOfMachines;
/**
* @return Maximum number of VMs in the App Service Environment.
*
*/
public Output maximumNumberOfMachines() {
return this.maximumNumberOfMachines;
}
/**
* Number of front-end instances.
*
*/
@Export(name="multiRoleCount", refs={Integer.class}, tree="[0]")
private Output multiRoleCount;
/**
* @return Number of front-end instances.
*
*/
public Output multiRoleCount() {
return this.multiRoleCount;
}
/**
* Front-end VM size, e.g. "Medium", "Large".
*
*/
@Export(name="multiSize", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> multiSize;
/**
* @return Front-end VM size, e.g. "Medium", "Large".
*
*/
public Output> multiSize() {
return Codegen.optional(this.multiSize);
}
/**
* Resource Name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource Name.
*
*/
public Output name() {
return this.name;
}
/**
* Full view of networking configuration for an ASE.
*
*/
@Export(name="networkingConfiguration", refs={AseV3NetworkingConfigurationResponse.class}, tree="[0]")
private Output* @Nullable */ AseV3NetworkingConfigurationResponse> networkingConfiguration;
/**
* @return Full view of networking configuration for an ASE.
*
*/
public Output> networkingConfiguration() {
return Codegen.optional(this.networkingConfiguration);
}
/**
* Provisioning state of the App Service Environment.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Provisioning state of the App Service Environment.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Current status of the App Service Environment.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return Current status of the App Service Environment.
*
*/
public Output status() {
return this.status;
}
/**
* <code>true</code> if the App Service Environment is suspended; otherwise, <code>false</code>. The environment can be suspended, e.g. when the management endpoint is no longer available
* (most likely because NSG blocked the incoming traffic).
*
*/
@Export(name="suspended", refs={Boolean.class}, tree="[0]")
private Output suspended;
/**
* @return <code>true</code> if the App Service Environment is suspended; otherwise, <code>false</code>. The environment can be suspended, e.g. when the management endpoint is no longer available
* (most likely because NSG blocked the incoming traffic).
*
*/
public Output suspended() {
return this.suspended;
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Resource type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type.
*
*/
public Output type() {
return this.type;
}
/**
* Whether an upgrade is available for this App Service Environment.
*
*/
@Export(name="upgradeAvailability", refs={String.class}, tree="[0]")
private Output upgradeAvailability;
/**
* @return Whether an upgrade is available for this App Service Environment.
*
*/
public Output upgradeAvailability() {
return this.upgradeAvailability;
}
/**
* Upgrade Preference
*
*/
@Export(name="upgradePreference", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> upgradePreference;
/**
* @return Upgrade Preference
*
*/
public Output> upgradePreference() {
return Codegen.optional(this.upgradePreference);
}
/**
* User added ip ranges to whitelist on ASE db
*
*/
@Export(name="userWhitelistedIpRanges", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> userWhitelistedIpRanges;
/**
* @return User added ip ranges to whitelist on ASE db
*
*/
public Output>> userWhitelistedIpRanges() {
return Codegen.optional(this.userWhitelistedIpRanges);
}
/**
* Description of the Virtual Network.
*
*/
@Export(name="virtualNetwork", refs={VirtualNetworkProfileResponse.class}, tree="[0]")
private Output virtualNetwork;
/**
* @return Description of the Virtual Network.
*
*/
public Output virtualNetwork() {
return this.virtualNetwork;
}
/**
* Whether or not this App Service Environment is zone-redundant.
*
*/
@Export(name="zoneRedundant", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> zoneRedundant;
/**
* @return Whether or not this App Service Environment is zone-redundant.
*
*/
public Output> zoneRedundant() {
return Codegen.optional(this.zoneRedundant);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public AppServiceEnvironment(java.lang.String name) {
this(name, AppServiceEnvironmentArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public AppServiceEnvironment(java.lang.String name, AppServiceEnvironmentArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public AppServiceEnvironment(java.lang.String name, AppServiceEnvironmentArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:web:AppServiceEnvironment", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private AppServiceEnvironment(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:web:AppServiceEnvironment", name, null, makeResourceOptions(options, id), false);
}
private static AppServiceEnvironmentArgs makeArgs(AppServiceEnvironmentArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AppServiceEnvironmentArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:web/v20150801:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20160901:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20180201:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20190801:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20200601:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20200901:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20201001:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20201201:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20210101:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20210115:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20210201:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20210301:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20220301:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20220901:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20230101:AppServiceEnvironment").build()),
Output.of(Alias.builder().type("azure-native:web/v20231201:AppServiceEnvironment").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static AppServiceEnvironment get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new AppServiceEnvironment(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy