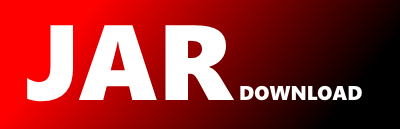
com.pulumi.azurenative.web.inputs.CloningInfoArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Information needed for cloning operation.
*
*/
public final class CloningInfoArgs extends com.pulumi.resources.ResourceArgs {
public static final CloningInfoArgs Empty = new CloningInfoArgs();
/**
* Application setting overrides for cloned app. If specified, these settings override the settings cloned
* from source app. Otherwise, application settings from source app are retained.
*
*/
@Import(name="appSettingsOverrides")
private @Nullable Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy