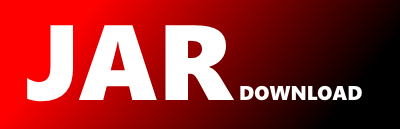
com.pulumi.azurenative.web.inputs.ListWebAppBackupStatusSecretsSlotArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.inputs;
import com.pulumi.azurenative.web.inputs.BackupScheduleArgs;
import com.pulumi.azurenative.web.inputs.DatabaseBackupSettingArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ListWebAppBackupStatusSecretsSlotArgs extends com.pulumi.resources.InvokeArgs {
public static final ListWebAppBackupStatusSecretsSlotArgs Empty = new ListWebAppBackupStatusSecretsSlotArgs();
/**
* ID of backup.
*
*/
@Import(name="backupId", required=true)
private Output backupId;
/**
* @return ID of backup.
*
*/
public Output backupId() {
return this.backupId;
}
/**
* Name of the backup.
*
*/
@Import(name="backupName")
private @Nullable Output backupName;
/**
* @return Name of the backup.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy