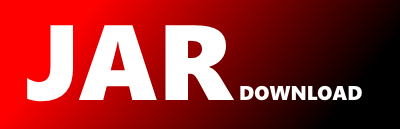
com.pulumi.azurenative.web.outputs.GetAppServicePlanResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.outputs;
import com.pulumi.azurenative.web.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.web.outputs.HostingEnvironmentProfileResponse;
import com.pulumi.azurenative.web.outputs.KubeEnvironmentProfileResponse;
import com.pulumi.azurenative.web.outputs.SkuDescriptionResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetAppServicePlanResult {
/**
* @return ServerFarm supports ElasticScale. Apps in this plan will scale as if the ServerFarm was ElasticPremium sku
*
*/
private @Nullable Boolean elasticScaleEnabled;
/**
* @return Extended Location.
*
*/
private @Nullable ExtendedLocationResponse extendedLocation;
/**
* @return The time when the server farm free offer expires.
*
*/
private @Nullable String freeOfferExpirationTime;
/**
* @return Geographical location for the App Service plan.
*
*/
private String geoRegion;
/**
* @return Specification for the App Service Environment to use for the App Service plan.
*
*/
private @Nullable HostingEnvironmentProfileResponse hostingEnvironmentProfile;
/**
* @return If Hyper-V container app service plan <code>true</code>, <code>false</code> otherwise.
*
*/
private @Nullable Boolean hyperV;
/**
* @return Resource Id.
*
*/
private String id;
/**
* @return If <code>true</code>, this App Service Plan owns spot instances.
*
*/
private @Nullable Boolean isSpot;
/**
* @return Obsolete: If Hyper-V container app service plan <code>true</code>, <code>false</code> otherwise.
*
*/
private @Nullable Boolean isXenon;
/**
* @return Kind of resource.
*
*/
private @Nullable String kind;
/**
* @return Specification for the Kubernetes Environment to use for the App Service plan.
*
*/
private @Nullable KubeEnvironmentProfileResponse kubeEnvironmentProfile;
/**
* @return Resource Location.
*
*/
private String location;
/**
* @return Maximum number of total workers allowed for this ElasticScaleEnabled App Service Plan
*
*/
private @Nullable Integer maximumElasticWorkerCount;
/**
* @return Maximum number of instances that can be assigned to this App Service plan.
*
*/
private Integer maximumNumberOfWorkers;
/**
* @return Resource Name.
*
*/
private String name;
/**
* @return Number of apps assigned to this App Service plan.
*
*/
private Integer numberOfSites;
/**
* @return The number of instances that are assigned to this App Service plan.
*
*/
private Integer numberOfWorkers;
/**
* @return If <code>true</code>, apps assigned to this App Service plan can be scaled independently.
* If <code>false</code>, apps assigned to this App Service plan will scale to all instances of the plan.
*
*/
private @Nullable Boolean perSiteScaling;
/**
* @return Provisioning state of the App Service Plan.
*
*/
private String provisioningState;
/**
* @return If Linux app service plan <code>true</code>, <code>false</code> otherwise.
*
*/
private @Nullable Boolean reserved;
/**
* @return Resource group of the App Service plan.
*
*/
private String resourceGroup;
/**
* @return Description of a SKU for a scalable resource.
*
*/
private @Nullable SkuDescriptionResponse sku;
/**
* @return The time when the server farm expires. Valid only if it is a spot server farm.
*
*/
private @Nullable String spotExpirationTime;
/**
* @return App Service plan status.
*
*/
private String status;
/**
* @return App Service plan subscription.
*
*/
private String subscription;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Scaling worker count.
*
*/
private @Nullable Integer targetWorkerCount;
/**
* @return Scaling worker size ID.
*
*/
private @Nullable Integer targetWorkerSizeId;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return Target worker tier assigned to the App Service plan.
*
*/
private @Nullable String workerTierName;
/**
* @return If <code>true</code>, this App Service Plan will perform availability zone balancing.
* If <code>false</code>, this App Service Plan will not perform availability zone balancing.
*
*/
private @Nullable Boolean zoneRedundant;
private GetAppServicePlanResult() {}
/**
* @return ServerFarm supports ElasticScale. Apps in this plan will scale as if the ServerFarm was ElasticPremium sku
*
*/
public Optional elasticScaleEnabled() {
return Optional.ofNullable(this.elasticScaleEnabled);
}
/**
* @return Extended Location.
*
*/
public Optional extendedLocation() {
return Optional.ofNullable(this.extendedLocation);
}
/**
* @return The time when the server farm free offer expires.
*
*/
public Optional freeOfferExpirationTime() {
return Optional.ofNullable(this.freeOfferExpirationTime);
}
/**
* @return Geographical location for the App Service plan.
*
*/
public String geoRegion() {
return this.geoRegion;
}
/**
* @return Specification for the App Service Environment to use for the App Service plan.
*
*/
public Optional hostingEnvironmentProfile() {
return Optional.ofNullable(this.hostingEnvironmentProfile);
}
/**
* @return If Hyper-V container app service plan <code>true</code>, <code>false</code> otherwise.
*
*/
public Optional hyperV() {
return Optional.ofNullable(this.hyperV);
}
/**
* @return Resource Id.
*
*/
public String id() {
return this.id;
}
/**
* @return If <code>true</code>, this App Service Plan owns spot instances.
*
*/
public Optional isSpot() {
return Optional.ofNullable(this.isSpot);
}
/**
* @return Obsolete: If Hyper-V container app service plan <code>true</code>, <code>false</code> otherwise.
*
*/
public Optional isXenon() {
return Optional.ofNullable(this.isXenon);
}
/**
* @return Kind of resource.
*
*/
public Optional kind() {
return Optional.ofNullable(this.kind);
}
/**
* @return Specification for the Kubernetes Environment to use for the App Service plan.
*
*/
public Optional kubeEnvironmentProfile() {
return Optional.ofNullable(this.kubeEnvironmentProfile);
}
/**
* @return Resource Location.
*
*/
public String location() {
return this.location;
}
/**
* @return Maximum number of total workers allowed for this ElasticScaleEnabled App Service Plan
*
*/
public Optional maximumElasticWorkerCount() {
return Optional.ofNullable(this.maximumElasticWorkerCount);
}
/**
* @return Maximum number of instances that can be assigned to this App Service plan.
*
*/
public Integer maximumNumberOfWorkers() {
return this.maximumNumberOfWorkers;
}
/**
* @return Resource Name.
*
*/
public String name() {
return this.name;
}
/**
* @return Number of apps assigned to this App Service plan.
*
*/
public Integer numberOfSites() {
return this.numberOfSites;
}
/**
* @return The number of instances that are assigned to this App Service plan.
*
*/
public Integer numberOfWorkers() {
return this.numberOfWorkers;
}
/**
* @return If <code>true</code>, apps assigned to this App Service plan can be scaled independently.
* If <code>false</code>, apps assigned to this App Service plan will scale to all instances of the plan.
*
*/
public Optional perSiteScaling() {
return Optional.ofNullable(this.perSiteScaling);
}
/**
* @return Provisioning state of the App Service Plan.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return If Linux app service plan <code>true</code>, <code>false</code> otherwise.
*
*/
public Optional reserved() {
return Optional.ofNullable(this.reserved);
}
/**
* @return Resource group of the App Service plan.
*
*/
public String resourceGroup() {
return this.resourceGroup;
}
/**
* @return Description of a SKU for a scalable resource.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return The time when the server farm expires. Valid only if it is a spot server farm.
*
*/
public Optional spotExpirationTime() {
return Optional.ofNullable(this.spotExpirationTime);
}
/**
* @return App Service plan status.
*
*/
public String status() {
return this.status;
}
/**
* @return App Service plan subscription.
*
*/
public String subscription() {
return this.subscription;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Scaling worker count.
*
*/
public Optional targetWorkerCount() {
return Optional.ofNullable(this.targetWorkerCount);
}
/**
* @return Scaling worker size ID.
*
*/
public Optional targetWorkerSizeId() {
return Optional.ofNullable(this.targetWorkerSizeId);
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return Target worker tier assigned to the App Service plan.
*
*/
public Optional workerTierName() {
return Optional.ofNullable(this.workerTierName);
}
/**
* @return If <code>true</code>, this App Service Plan will perform availability zone balancing.
* If <code>false</code>, this App Service Plan will not perform availability zone balancing.
*
*/
public Optional zoneRedundant() {
return Optional.ofNullable(this.zoneRedundant);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAppServicePlanResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean elasticScaleEnabled;
private @Nullable ExtendedLocationResponse extendedLocation;
private @Nullable String freeOfferExpirationTime;
private String geoRegion;
private @Nullable HostingEnvironmentProfileResponse hostingEnvironmentProfile;
private @Nullable Boolean hyperV;
private String id;
private @Nullable Boolean isSpot;
private @Nullable Boolean isXenon;
private @Nullable String kind;
private @Nullable KubeEnvironmentProfileResponse kubeEnvironmentProfile;
private String location;
private @Nullable Integer maximumElasticWorkerCount;
private Integer maximumNumberOfWorkers;
private String name;
private Integer numberOfSites;
private Integer numberOfWorkers;
private @Nullable Boolean perSiteScaling;
private String provisioningState;
private @Nullable Boolean reserved;
private String resourceGroup;
private @Nullable SkuDescriptionResponse sku;
private @Nullable String spotExpirationTime;
private String status;
private String subscription;
private @Nullable Map tags;
private @Nullable Integer targetWorkerCount;
private @Nullable Integer targetWorkerSizeId;
private String type;
private @Nullable String workerTierName;
private @Nullable Boolean zoneRedundant;
public Builder() {}
public Builder(GetAppServicePlanResult defaults) {
Objects.requireNonNull(defaults);
this.elasticScaleEnabled = defaults.elasticScaleEnabled;
this.extendedLocation = defaults.extendedLocation;
this.freeOfferExpirationTime = defaults.freeOfferExpirationTime;
this.geoRegion = defaults.geoRegion;
this.hostingEnvironmentProfile = defaults.hostingEnvironmentProfile;
this.hyperV = defaults.hyperV;
this.id = defaults.id;
this.isSpot = defaults.isSpot;
this.isXenon = defaults.isXenon;
this.kind = defaults.kind;
this.kubeEnvironmentProfile = defaults.kubeEnvironmentProfile;
this.location = defaults.location;
this.maximumElasticWorkerCount = defaults.maximumElasticWorkerCount;
this.maximumNumberOfWorkers = defaults.maximumNumberOfWorkers;
this.name = defaults.name;
this.numberOfSites = defaults.numberOfSites;
this.numberOfWorkers = defaults.numberOfWorkers;
this.perSiteScaling = defaults.perSiteScaling;
this.provisioningState = defaults.provisioningState;
this.reserved = defaults.reserved;
this.resourceGroup = defaults.resourceGroup;
this.sku = defaults.sku;
this.spotExpirationTime = defaults.spotExpirationTime;
this.status = defaults.status;
this.subscription = defaults.subscription;
this.tags = defaults.tags;
this.targetWorkerCount = defaults.targetWorkerCount;
this.targetWorkerSizeId = defaults.targetWorkerSizeId;
this.type = defaults.type;
this.workerTierName = defaults.workerTierName;
this.zoneRedundant = defaults.zoneRedundant;
}
@CustomType.Setter
public Builder elasticScaleEnabled(@Nullable Boolean elasticScaleEnabled) {
this.elasticScaleEnabled = elasticScaleEnabled;
return this;
}
@CustomType.Setter
public Builder extendedLocation(@Nullable ExtendedLocationResponse extendedLocation) {
this.extendedLocation = extendedLocation;
return this;
}
@CustomType.Setter
public Builder freeOfferExpirationTime(@Nullable String freeOfferExpirationTime) {
this.freeOfferExpirationTime = freeOfferExpirationTime;
return this;
}
@CustomType.Setter
public Builder geoRegion(String geoRegion) {
if (geoRegion == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "geoRegion");
}
this.geoRegion = geoRegion;
return this;
}
@CustomType.Setter
public Builder hostingEnvironmentProfile(@Nullable HostingEnvironmentProfileResponse hostingEnvironmentProfile) {
this.hostingEnvironmentProfile = hostingEnvironmentProfile;
return this;
}
@CustomType.Setter
public Builder hyperV(@Nullable Boolean hyperV) {
this.hyperV = hyperV;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isSpot(@Nullable Boolean isSpot) {
this.isSpot = isSpot;
return this;
}
@CustomType.Setter
public Builder isXenon(@Nullable Boolean isXenon) {
this.isXenon = isXenon;
return this;
}
@CustomType.Setter
public Builder kind(@Nullable String kind) {
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder kubeEnvironmentProfile(@Nullable KubeEnvironmentProfileResponse kubeEnvironmentProfile) {
this.kubeEnvironmentProfile = kubeEnvironmentProfile;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder maximumElasticWorkerCount(@Nullable Integer maximumElasticWorkerCount) {
this.maximumElasticWorkerCount = maximumElasticWorkerCount;
return this;
}
@CustomType.Setter
public Builder maximumNumberOfWorkers(Integer maximumNumberOfWorkers) {
if (maximumNumberOfWorkers == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "maximumNumberOfWorkers");
}
this.maximumNumberOfWorkers = maximumNumberOfWorkers;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder numberOfSites(Integer numberOfSites) {
if (numberOfSites == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "numberOfSites");
}
this.numberOfSites = numberOfSites;
return this;
}
@CustomType.Setter
public Builder numberOfWorkers(Integer numberOfWorkers) {
if (numberOfWorkers == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "numberOfWorkers");
}
this.numberOfWorkers = numberOfWorkers;
return this;
}
@CustomType.Setter
public Builder perSiteScaling(@Nullable Boolean perSiteScaling) {
this.perSiteScaling = perSiteScaling;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder reserved(@Nullable Boolean reserved) {
this.reserved = reserved;
return this;
}
@CustomType.Setter
public Builder resourceGroup(String resourceGroup) {
if (resourceGroup == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "resourceGroup");
}
this.resourceGroup = resourceGroup;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable SkuDescriptionResponse sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder spotExpirationTime(@Nullable String spotExpirationTime) {
this.spotExpirationTime = spotExpirationTime;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder subscription(String subscription) {
if (subscription == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "subscription");
}
this.subscription = subscription;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder targetWorkerCount(@Nullable Integer targetWorkerCount) {
this.targetWorkerCount = targetWorkerCount;
return this;
}
@CustomType.Setter
public Builder targetWorkerSizeId(@Nullable Integer targetWorkerSizeId) {
this.targetWorkerSizeId = targetWorkerSizeId;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetAppServicePlanResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder workerTierName(@Nullable String workerTierName) {
this.workerTierName = workerTierName;
return this;
}
@CustomType.Setter
public Builder zoneRedundant(@Nullable Boolean zoneRedundant) {
this.zoneRedundant = zoneRedundant;
return this;
}
public GetAppServicePlanResult build() {
final var _resultValue = new GetAppServicePlanResult();
_resultValue.elasticScaleEnabled = elasticScaleEnabled;
_resultValue.extendedLocation = extendedLocation;
_resultValue.freeOfferExpirationTime = freeOfferExpirationTime;
_resultValue.geoRegion = geoRegion;
_resultValue.hostingEnvironmentProfile = hostingEnvironmentProfile;
_resultValue.hyperV = hyperV;
_resultValue.id = id;
_resultValue.isSpot = isSpot;
_resultValue.isXenon = isXenon;
_resultValue.kind = kind;
_resultValue.kubeEnvironmentProfile = kubeEnvironmentProfile;
_resultValue.location = location;
_resultValue.maximumElasticWorkerCount = maximumElasticWorkerCount;
_resultValue.maximumNumberOfWorkers = maximumNumberOfWorkers;
_resultValue.name = name;
_resultValue.numberOfSites = numberOfSites;
_resultValue.numberOfWorkers = numberOfWorkers;
_resultValue.perSiteScaling = perSiteScaling;
_resultValue.provisioningState = provisioningState;
_resultValue.reserved = reserved;
_resultValue.resourceGroup = resourceGroup;
_resultValue.sku = sku;
_resultValue.spotExpirationTime = spotExpirationTime;
_resultValue.status = status;
_resultValue.subscription = subscription;
_resultValue.tags = tags;
_resultValue.targetWorkerCount = targetWorkerCount;
_resultValue.targetWorkerSizeId = targetWorkerSizeId;
_resultValue.type = type;
_resultValue.workerTierName = workerTierName;
_resultValue.zoneRedundant = zoneRedundant;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy