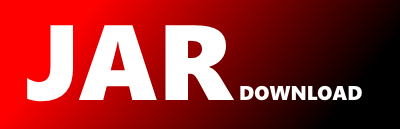
com.pulumi.azurenative.workloads.inputs.SapNetWeaverProviderInstancePropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.workloads.inputs;
import com.pulumi.azurenative.workloads.enums.SslPreference;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Gets or sets the provider properties.
*
*/
public final class SapNetWeaverProviderInstancePropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final SapNetWeaverProviderInstancePropertiesArgs Empty = new SapNetWeaverProviderInstancePropertiesArgs();
/**
* The provider type. For example, the value can be SapHana.
* Expected value is 'SapNetWeaver'.
*
*/
@Import(name="providerType", required=true)
private Output providerType;
/**
* @return The provider type. For example, the value can be SapHana.
* Expected value is 'SapNetWeaver'.
*
*/
public Output providerType() {
return this.providerType;
}
/**
* Gets or sets the SAP Client ID.
*
*/
@Import(name="sapClientId")
private @Nullable Output sapClientId;
/**
* @return Gets or sets the SAP Client ID.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy