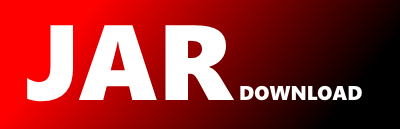
com.pulumi.azurenative.workloads.outputs.DBBackupPolicyPropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.workloads.outputs;
import com.pulumi.azurenative.workloads.outputs.SettingsResponse;
import com.pulumi.azurenative.workloads.outputs.SubProtectionPolicyResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DBBackupPolicyPropertiesResponse {
/**
* @return This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'AzureWorkload'.
*
*/
private String backupManagementType;
/**
* @return Fix the policy inconsistency
*
*/
private @Nullable Boolean makePolicyConsistent;
/**
* @return The name of the DB backup policy.
*
*/
private String name;
/**
* @return Number of items associated with this policy.
*
*/
private @Nullable Integer protectedItemsCount;
/**
* @return ResourceGuard Operation Requests
*
*/
private @Nullable List resourceGuardOperationRequests;
/**
* @return Common settings for the backup management
*
*/
private @Nullable SettingsResponse settings;
/**
* @return List of sub-protection policies which includes schedule and retention
*
*/
private @Nullable List subProtectionPolicy;
/**
* @return Type of workload for the backup management
*
*/
private @Nullable String workLoadType;
private DBBackupPolicyPropertiesResponse() {}
/**
* @return This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'AzureWorkload'.
*
*/
public String backupManagementType() {
return this.backupManagementType;
}
/**
* @return Fix the policy inconsistency
*
*/
public Optional makePolicyConsistent() {
return Optional.ofNullable(this.makePolicyConsistent);
}
/**
* @return The name of the DB backup policy.
*
*/
public String name() {
return this.name;
}
/**
* @return Number of items associated with this policy.
*
*/
public Optional protectedItemsCount() {
return Optional.ofNullable(this.protectedItemsCount);
}
/**
* @return ResourceGuard Operation Requests
*
*/
public List resourceGuardOperationRequests() {
return this.resourceGuardOperationRequests == null ? List.of() : this.resourceGuardOperationRequests;
}
/**
* @return Common settings for the backup management
*
*/
public Optional settings() {
return Optional.ofNullable(this.settings);
}
/**
* @return List of sub-protection policies which includes schedule and retention
*
*/
public List subProtectionPolicy() {
return this.subProtectionPolicy == null ? List.of() : this.subProtectionPolicy;
}
/**
* @return Type of workload for the backup management
*
*/
public Optional workLoadType() {
return Optional.ofNullable(this.workLoadType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DBBackupPolicyPropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String backupManagementType;
private @Nullable Boolean makePolicyConsistent;
private String name;
private @Nullable Integer protectedItemsCount;
private @Nullable List resourceGuardOperationRequests;
private @Nullable SettingsResponse settings;
private @Nullable List subProtectionPolicy;
private @Nullable String workLoadType;
public Builder() {}
public Builder(DBBackupPolicyPropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.backupManagementType = defaults.backupManagementType;
this.makePolicyConsistent = defaults.makePolicyConsistent;
this.name = defaults.name;
this.protectedItemsCount = defaults.protectedItemsCount;
this.resourceGuardOperationRequests = defaults.resourceGuardOperationRequests;
this.settings = defaults.settings;
this.subProtectionPolicy = defaults.subProtectionPolicy;
this.workLoadType = defaults.workLoadType;
}
@CustomType.Setter
public Builder backupManagementType(String backupManagementType) {
if (backupManagementType == null) {
throw new MissingRequiredPropertyException("DBBackupPolicyPropertiesResponse", "backupManagementType");
}
this.backupManagementType = backupManagementType;
return this;
}
@CustomType.Setter
public Builder makePolicyConsistent(@Nullable Boolean makePolicyConsistent) {
this.makePolicyConsistent = makePolicyConsistent;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("DBBackupPolicyPropertiesResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder protectedItemsCount(@Nullable Integer protectedItemsCount) {
this.protectedItemsCount = protectedItemsCount;
return this;
}
@CustomType.Setter
public Builder resourceGuardOperationRequests(@Nullable List resourceGuardOperationRequests) {
this.resourceGuardOperationRequests = resourceGuardOperationRequests;
return this;
}
public Builder resourceGuardOperationRequests(String... resourceGuardOperationRequests) {
return resourceGuardOperationRequests(List.of(resourceGuardOperationRequests));
}
@CustomType.Setter
public Builder settings(@Nullable SettingsResponse settings) {
this.settings = settings;
return this;
}
@CustomType.Setter
public Builder subProtectionPolicy(@Nullable List subProtectionPolicy) {
this.subProtectionPolicy = subProtectionPolicy;
return this;
}
public Builder subProtectionPolicy(SubProtectionPolicyResponse... subProtectionPolicy) {
return subProtectionPolicy(List.of(subProtectionPolicy));
}
@CustomType.Setter
public Builder workLoadType(@Nullable String workLoadType) {
this.workLoadType = workLoadType;
return this;
}
public DBBackupPolicyPropertiesResponse build() {
final var _resultValue = new DBBackupPolicyPropertiesResponse();
_resultValue.backupManagementType = backupManagementType;
_resultValue.makePolicyConsistent = makePolicyConsistent;
_resultValue.name = name;
_resultValue.protectedItemsCount = protectedItemsCount;
_resultValue.resourceGuardOperationRequests = resourceGuardOperationRequests;
_resultValue.settings = settings;
_resultValue.subProtectionPolicy = subProtectionPolicy;
_resultValue.workLoadType = workLoadType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy