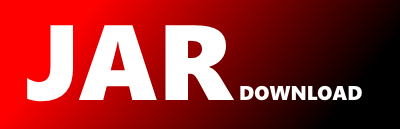
com.pulumi.azurenative.workloads.outputs.DiskDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.workloads.outputs;
import com.pulumi.azurenative.workloads.outputs.DiskSkuResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DiskDetailsResponse {
/**
* @return The disk tier, e.g. P10, E10.
*
*/
private @Nullable String diskTier;
/**
* @return The disk Iops.
*
*/
private @Nullable Double iopsReadWrite;
/**
* @return The maximum supported disk count.
*
*/
private @Nullable Double maximumSupportedDiskCount;
/**
* @return The disk provisioned throughput in MBps.
*
*/
private @Nullable Double mbpsReadWrite;
/**
* @return The minimum supported disk count.
*
*/
private @Nullable Double minimumSupportedDiskCount;
/**
* @return The disk size in GB.
*
*/
private @Nullable Double sizeGB;
/**
* @return The type of disk sku. For example, Standard_LRS, Standard_ZRS, Premium_LRS, Premium_ZRS.
*
*/
private @Nullable DiskSkuResponse sku;
private DiskDetailsResponse() {}
/**
* @return The disk tier, e.g. P10, E10.
*
*/
public Optional diskTier() {
return Optional.ofNullable(this.diskTier);
}
/**
* @return The disk Iops.
*
*/
public Optional iopsReadWrite() {
return Optional.ofNullable(this.iopsReadWrite);
}
/**
* @return The maximum supported disk count.
*
*/
public Optional maximumSupportedDiskCount() {
return Optional.ofNullable(this.maximumSupportedDiskCount);
}
/**
* @return The disk provisioned throughput in MBps.
*
*/
public Optional mbpsReadWrite() {
return Optional.ofNullable(this.mbpsReadWrite);
}
/**
* @return The minimum supported disk count.
*
*/
public Optional minimumSupportedDiskCount() {
return Optional.ofNullable(this.minimumSupportedDiskCount);
}
/**
* @return The disk size in GB.
*
*/
public Optional sizeGB() {
return Optional.ofNullable(this.sizeGB);
}
/**
* @return The type of disk sku. For example, Standard_LRS, Standard_ZRS, Premium_LRS, Premium_ZRS.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DiskDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String diskTier;
private @Nullable Double iopsReadWrite;
private @Nullable Double maximumSupportedDiskCount;
private @Nullable Double mbpsReadWrite;
private @Nullable Double minimumSupportedDiskCount;
private @Nullable Double sizeGB;
private @Nullable DiskSkuResponse sku;
public Builder() {}
public Builder(DiskDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.diskTier = defaults.diskTier;
this.iopsReadWrite = defaults.iopsReadWrite;
this.maximumSupportedDiskCount = defaults.maximumSupportedDiskCount;
this.mbpsReadWrite = defaults.mbpsReadWrite;
this.minimumSupportedDiskCount = defaults.minimumSupportedDiskCount;
this.sizeGB = defaults.sizeGB;
this.sku = defaults.sku;
}
@CustomType.Setter
public Builder diskTier(@Nullable String diskTier) {
this.diskTier = diskTier;
return this;
}
@CustomType.Setter
public Builder iopsReadWrite(@Nullable Double iopsReadWrite) {
this.iopsReadWrite = iopsReadWrite;
return this;
}
@CustomType.Setter
public Builder maximumSupportedDiskCount(@Nullable Double maximumSupportedDiskCount) {
this.maximumSupportedDiskCount = maximumSupportedDiskCount;
return this;
}
@CustomType.Setter
public Builder mbpsReadWrite(@Nullable Double mbpsReadWrite) {
this.mbpsReadWrite = mbpsReadWrite;
return this;
}
@CustomType.Setter
public Builder minimumSupportedDiskCount(@Nullable Double minimumSupportedDiskCount) {
this.minimumSupportedDiskCount = minimumSupportedDiskCount;
return this;
}
@CustomType.Setter
public Builder sizeGB(@Nullable Double sizeGB) {
this.sizeGB = sizeGB;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable DiskSkuResponse sku) {
this.sku = sku;
return this;
}
public DiskDetailsResponse build() {
final var _resultValue = new DiskDetailsResponse();
_resultValue.diskTier = diskTier;
_resultValue.iopsReadWrite = iopsReadWrite;
_resultValue.maximumSupportedDiskCount = maximumSupportedDiskCount;
_resultValue.mbpsReadWrite = mbpsReadWrite;
_resultValue.minimumSupportedDiskCount = minimumSupportedDiskCount;
_resultValue.sizeGB = sizeGB;
_resultValue.sku = sku;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy