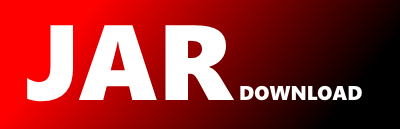
com.pulumi.azurenative.aad.DomainServiceArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.aad;
import com.pulumi.azurenative.aad.enums.FilteredSync;
import com.pulumi.azurenative.aad.enums.SyncScope;
import com.pulumi.azurenative.aad.inputs.ConfigDiagnosticsArgs;
import com.pulumi.azurenative.aad.inputs.DomainSecuritySettingsArgs;
import com.pulumi.azurenative.aad.inputs.LdapsSettingsArgs;
import com.pulumi.azurenative.aad.inputs.NotificationSettingsArgs;
import com.pulumi.azurenative.aad.inputs.ReplicaSetArgs;
import com.pulumi.azurenative.aad.inputs.ResourceForestSettingsArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DomainServiceArgs extends com.pulumi.resources.ResourceArgs {
public static final DomainServiceArgs Empty = new DomainServiceArgs();
/**
* Configuration diagnostics data containing latest execution from client.
*
*/
@Import(name="configDiagnostics")
private @Nullable Output configDiagnostics;
/**
* @return Configuration diagnostics data containing latest execution from client.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy