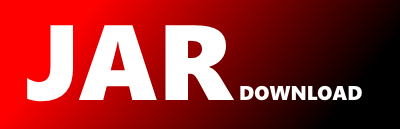
com.pulumi.azurenative.aad.outputs.DomainSecuritySettingsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.aad.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DomainSecuritySettingsResponse {
/**
* @return A flag to determine whether or not ChannelBinding is enabled or disabled.
*
*/
private @Nullable String channelBinding;
/**
* @return A flag to determine whether or not KerberosArmoring is enabled or disabled.
*
*/
private @Nullable String kerberosArmoring;
/**
* @return A flag to determine whether or not KerberosRc4Encryption is enabled or disabled.
*
*/
private @Nullable String kerberosRc4Encryption;
/**
* @return A flag to determine whether or not LdapSigning is enabled or disabled.
*
*/
private @Nullable String ldapSigning;
/**
* @return A flag to determine whether or not NtlmV1 is enabled or disabled.
*
*/
private @Nullable String ntlmV1;
/**
* @return A flag to determine whether or not SyncKerberosPasswords is enabled or disabled.
*
*/
private @Nullable String syncKerberosPasswords;
/**
* @return A flag to determine whether or not SyncNtlmPasswords is enabled or disabled.
*
*/
private @Nullable String syncNtlmPasswords;
/**
* @return A flag to determine whether or not SyncOnPremPasswords is enabled or disabled.
*
*/
private @Nullable String syncOnPremPasswords;
/**
* @return A flag to determine whether or not TlsV1 is enabled or disabled.
*
*/
private @Nullable String tlsV1;
private DomainSecuritySettingsResponse() {}
/**
* @return A flag to determine whether or not ChannelBinding is enabled or disabled.
*
*/
public Optional channelBinding() {
return Optional.ofNullable(this.channelBinding);
}
/**
* @return A flag to determine whether or not KerberosArmoring is enabled or disabled.
*
*/
public Optional kerberosArmoring() {
return Optional.ofNullable(this.kerberosArmoring);
}
/**
* @return A flag to determine whether or not KerberosRc4Encryption is enabled or disabled.
*
*/
public Optional kerberosRc4Encryption() {
return Optional.ofNullable(this.kerberosRc4Encryption);
}
/**
* @return A flag to determine whether or not LdapSigning is enabled or disabled.
*
*/
public Optional ldapSigning() {
return Optional.ofNullable(this.ldapSigning);
}
/**
* @return A flag to determine whether or not NtlmV1 is enabled or disabled.
*
*/
public Optional ntlmV1() {
return Optional.ofNullable(this.ntlmV1);
}
/**
* @return A flag to determine whether or not SyncKerberosPasswords is enabled or disabled.
*
*/
public Optional syncKerberosPasswords() {
return Optional.ofNullable(this.syncKerberosPasswords);
}
/**
* @return A flag to determine whether or not SyncNtlmPasswords is enabled or disabled.
*
*/
public Optional syncNtlmPasswords() {
return Optional.ofNullable(this.syncNtlmPasswords);
}
/**
* @return A flag to determine whether or not SyncOnPremPasswords is enabled or disabled.
*
*/
public Optional syncOnPremPasswords() {
return Optional.ofNullable(this.syncOnPremPasswords);
}
/**
* @return A flag to determine whether or not TlsV1 is enabled or disabled.
*
*/
public Optional tlsV1() {
return Optional.ofNullable(this.tlsV1);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DomainSecuritySettingsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String channelBinding;
private @Nullable String kerberosArmoring;
private @Nullable String kerberosRc4Encryption;
private @Nullable String ldapSigning;
private @Nullable String ntlmV1;
private @Nullable String syncKerberosPasswords;
private @Nullable String syncNtlmPasswords;
private @Nullable String syncOnPremPasswords;
private @Nullable String tlsV1;
public Builder() {}
public Builder(DomainSecuritySettingsResponse defaults) {
Objects.requireNonNull(defaults);
this.channelBinding = defaults.channelBinding;
this.kerberosArmoring = defaults.kerberosArmoring;
this.kerberosRc4Encryption = defaults.kerberosRc4Encryption;
this.ldapSigning = defaults.ldapSigning;
this.ntlmV1 = defaults.ntlmV1;
this.syncKerberosPasswords = defaults.syncKerberosPasswords;
this.syncNtlmPasswords = defaults.syncNtlmPasswords;
this.syncOnPremPasswords = defaults.syncOnPremPasswords;
this.tlsV1 = defaults.tlsV1;
}
@CustomType.Setter
public Builder channelBinding(@Nullable String channelBinding) {
this.channelBinding = channelBinding;
return this;
}
@CustomType.Setter
public Builder kerberosArmoring(@Nullable String kerberosArmoring) {
this.kerberosArmoring = kerberosArmoring;
return this;
}
@CustomType.Setter
public Builder kerberosRc4Encryption(@Nullable String kerberosRc4Encryption) {
this.kerberosRc4Encryption = kerberosRc4Encryption;
return this;
}
@CustomType.Setter
public Builder ldapSigning(@Nullable String ldapSigning) {
this.ldapSigning = ldapSigning;
return this;
}
@CustomType.Setter
public Builder ntlmV1(@Nullable String ntlmV1) {
this.ntlmV1 = ntlmV1;
return this;
}
@CustomType.Setter
public Builder syncKerberosPasswords(@Nullable String syncKerberosPasswords) {
this.syncKerberosPasswords = syncKerberosPasswords;
return this;
}
@CustomType.Setter
public Builder syncNtlmPasswords(@Nullable String syncNtlmPasswords) {
this.syncNtlmPasswords = syncNtlmPasswords;
return this;
}
@CustomType.Setter
public Builder syncOnPremPasswords(@Nullable String syncOnPremPasswords) {
this.syncOnPremPasswords = syncOnPremPasswords;
return this;
}
@CustomType.Setter
public Builder tlsV1(@Nullable String tlsV1) {
this.tlsV1 = tlsV1;
return this;
}
public DomainSecuritySettingsResponse build() {
final var _resultValue = new DomainSecuritySettingsResponse();
_resultValue.channelBinding = channelBinding;
_resultValue.kerberosArmoring = kerberosArmoring;
_resultValue.kerberosRc4Encryption = kerberosRc4Encryption;
_resultValue.ldapSigning = ldapSigning;
_resultValue.ntlmV1 = ntlmV1;
_resultValue.syncKerberosPasswords = syncKerberosPasswords;
_resultValue.syncNtlmPasswords = syncNtlmPasswords;
_resultValue.syncOnPremPasswords = syncOnPremPasswords;
_resultValue.tlsV1 = tlsV1;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy