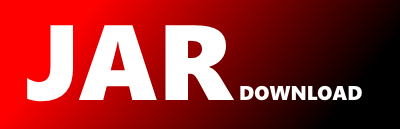
com.pulumi.azurenative.aadiam.outputs.GetDiagnosticSettingResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.aadiam.outputs;
import com.pulumi.azurenative.aadiam.outputs.LogSettingsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDiagnosticSettingResult {
/**
* @return The resource Id for the event hub authorization rule.
*
*/
private @Nullable String eventHubAuthorizationRuleId;
/**
* @return The name of the event hub. If none is specified, the default event hub will be selected.
*
*/
private @Nullable String eventHubName;
/**
* @return Azure resource Id
*
*/
private String id;
/**
* @return The list of logs settings.
*
*/
private @Nullable List logs;
/**
* @return Azure resource name
*
*/
private String name;
/**
* @return The service bus rule Id of the diagnostic setting. This is here to maintain backwards compatibility.
*
*/
private @Nullable String serviceBusRuleId;
/**
* @return The resource ID of the storage account to which you would like to send Diagnostic Logs.
*
*/
private @Nullable String storageAccountId;
/**
* @return Azure resource type
*
*/
private String type;
/**
* @return The workspace ID (resource ID of a Log Analytics workspace) for a Log Analytics workspace to which you would like to send Diagnostic Logs. Example: /subscriptions/4b9e8510-67ab-4e9a-95a9-e2f1e570ea9c/resourceGroups/insights-integration/providers/Microsoft.OperationalInsights/workspaces/viruela2
*
*/
private @Nullable String workspaceId;
private GetDiagnosticSettingResult() {}
/**
* @return The resource Id for the event hub authorization rule.
*
*/
public Optional eventHubAuthorizationRuleId() {
return Optional.ofNullable(this.eventHubAuthorizationRuleId);
}
/**
* @return The name of the event hub. If none is specified, the default event hub will be selected.
*
*/
public Optional eventHubName() {
return Optional.ofNullable(this.eventHubName);
}
/**
* @return Azure resource Id
*
*/
public String id() {
return this.id;
}
/**
* @return The list of logs settings.
*
*/
public List logs() {
return this.logs == null ? List.of() : this.logs;
}
/**
* @return Azure resource name
*
*/
public String name() {
return this.name;
}
/**
* @return The service bus rule Id of the diagnostic setting. This is here to maintain backwards compatibility.
*
*/
public Optional serviceBusRuleId() {
return Optional.ofNullable(this.serviceBusRuleId);
}
/**
* @return The resource ID of the storage account to which you would like to send Diagnostic Logs.
*
*/
public Optional storageAccountId() {
return Optional.ofNullable(this.storageAccountId);
}
/**
* @return Azure resource type
*
*/
public String type() {
return this.type;
}
/**
* @return The workspace ID (resource ID of a Log Analytics workspace) for a Log Analytics workspace to which you would like to send Diagnostic Logs. Example: /subscriptions/4b9e8510-67ab-4e9a-95a9-e2f1e570ea9c/resourceGroups/insights-integration/providers/Microsoft.OperationalInsights/workspaces/viruela2
*
*/
public Optional workspaceId() {
return Optional.ofNullable(this.workspaceId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDiagnosticSettingResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String eventHubAuthorizationRuleId;
private @Nullable String eventHubName;
private String id;
private @Nullable List logs;
private String name;
private @Nullable String serviceBusRuleId;
private @Nullable String storageAccountId;
private String type;
private @Nullable String workspaceId;
public Builder() {}
public Builder(GetDiagnosticSettingResult defaults) {
Objects.requireNonNull(defaults);
this.eventHubAuthorizationRuleId = defaults.eventHubAuthorizationRuleId;
this.eventHubName = defaults.eventHubName;
this.id = defaults.id;
this.logs = defaults.logs;
this.name = defaults.name;
this.serviceBusRuleId = defaults.serviceBusRuleId;
this.storageAccountId = defaults.storageAccountId;
this.type = defaults.type;
this.workspaceId = defaults.workspaceId;
}
@CustomType.Setter
public Builder eventHubAuthorizationRuleId(@Nullable String eventHubAuthorizationRuleId) {
this.eventHubAuthorizationRuleId = eventHubAuthorizationRuleId;
return this;
}
@CustomType.Setter
public Builder eventHubName(@Nullable String eventHubName) {
this.eventHubName = eventHubName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDiagnosticSettingResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder logs(@Nullable List logs) {
this.logs = logs;
return this;
}
public Builder logs(LogSettingsResponse... logs) {
return logs(List.of(logs));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDiagnosticSettingResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder serviceBusRuleId(@Nullable String serviceBusRuleId) {
this.serviceBusRuleId = serviceBusRuleId;
return this;
}
@CustomType.Setter
public Builder storageAccountId(@Nullable String storageAccountId) {
this.storageAccountId = storageAccountId;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDiagnosticSettingResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder workspaceId(@Nullable String workspaceId) {
this.workspaceId = workspaceId;
return this;
}
public GetDiagnosticSettingResult build() {
final var _resultValue = new GetDiagnosticSettingResult();
_resultValue.eventHubAuthorizationRuleId = eventHubAuthorizationRuleId;
_resultValue.eventHubName = eventHubName;
_resultValue.id = id;
_resultValue.logs = logs;
_resultValue.name = name;
_resultValue.serviceBusRuleId = serviceBusRuleId;
_resultValue.storageAccountId = storageAccountId;
_resultValue.type = type;
_resultValue.workspaceId = workspaceId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy