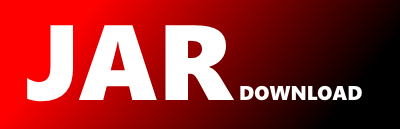
com.pulumi.azurenative.agfoodplatform.inputs.SolutionPropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.agfoodplatform.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Solution resource properties.
*
*/
public final class SolutionPropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final SolutionPropertiesArgs Empty = new SolutionPropertiesArgs();
/**
* SaaS application Marketplace Publisher Id.
*
*/
@Import(name="marketplacePublisherId", required=true)
private Output marketplacePublisherId;
/**
* @return SaaS application Marketplace Publisher Id.
*
*/
public Output marketplacePublisherId() {
return this.marketplacePublisherId;
}
/**
* SaaS application Offer Id.
*
*/
@Import(name="offerId", required=true)
private Output offerId;
/**
* @return SaaS application Offer Id.
*
*/
public Output offerId() {
return this.offerId;
}
/**
* SaaS application Plan Id.
*
*/
@Import(name="planId", required=true)
private Output planId;
/**
* @return SaaS application Plan Id.
*
*/
public Output planId() {
return this.planId;
}
/**
* Role Assignment Id.
*
*/
@Import(name="roleAssignmentId")
private @Nullable Output roleAssignmentId;
/**
* @return Role Assignment Id.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy