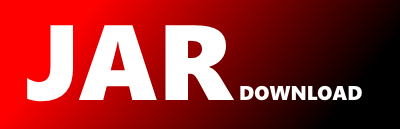
com.pulumi.azurenative.alertsmanagement.outputs.PrometheusRuleResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.alertsmanagement.outputs;
import com.pulumi.azurenative.alertsmanagement.outputs.PrometheusRuleGroupActionResponse;
import com.pulumi.azurenative.alertsmanagement.outputs.PrometheusRuleResolveConfigurationResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class PrometheusRuleResponse {
/**
* @return Actions that are performed when the alert rule becomes active, and when an alert condition is resolved.
*
*/
private @Nullable List actions;
/**
* @return Alert rule name.
*
*/
private @Nullable String alert;
/**
* @return The annotations clause specifies a set of informational labels that can be used to store longer additional information such as alert descriptions or runbook links. The annotation values can be templated.
*
*/
private @Nullable Map annotations;
/**
* @return Enable/disable rule.
*
*/
private @Nullable Boolean enabled;
/**
* @return The PromQL expression to evaluate. https://prometheus.io/docs/prometheus/latest/querying/basics/. Evaluated periodically as given by 'interval', and the result recorded as a new set of time series with the metric name as given by 'record'.
*
*/
private String expression;
/**
* @return The amount of time alert must be active before firing.
*
*/
private @Nullable String for_;
/**
* @return Labels to add or overwrite before storing the result.
*
*/
private @Nullable Map labels;
/**
* @return Recorded metrics name.
*
*/
private @Nullable String record;
/**
* @return Defines the configuration for resolving fired alerts. Only relevant for alerts.
*
*/
private @Nullable PrometheusRuleResolveConfigurationResponse resolveConfiguration;
/**
* @return The severity of the alerts fired by the rule. Must be between 0 and 4.
*
*/
private @Nullable Integer severity;
private PrometheusRuleResponse() {}
/**
* @return Actions that are performed when the alert rule becomes active, and when an alert condition is resolved.
*
*/
public List actions() {
return this.actions == null ? List.of() : this.actions;
}
/**
* @return Alert rule name.
*
*/
public Optional alert() {
return Optional.ofNullable(this.alert);
}
/**
* @return The annotations clause specifies a set of informational labels that can be used to store longer additional information such as alert descriptions or runbook links. The annotation values can be templated.
*
*/
public Map annotations() {
return this.annotations == null ? Map.of() : this.annotations;
}
/**
* @return Enable/disable rule.
*
*/
public Optional enabled() {
return Optional.ofNullable(this.enabled);
}
/**
* @return The PromQL expression to evaluate. https://prometheus.io/docs/prometheus/latest/querying/basics/. Evaluated periodically as given by 'interval', and the result recorded as a new set of time series with the metric name as given by 'record'.
*
*/
public String expression() {
return this.expression;
}
/**
* @return The amount of time alert must be active before firing.
*
*/
public Optional for_() {
return Optional.ofNullable(this.for_);
}
/**
* @return Labels to add or overwrite before storing the result.
*
*/
public Map labels() {
return this.labels == null ? Map.of() : this.labels;
}
/**
* @return Recorded metrics name.
*
*/
public Optional record() {
return Optional.ofNullable(this.record);
}
/**
* @return Defines the configuration for resolving fired alerts. Only relevant for alerts.
*
*/
public Optional resolveConfiguration() {
return Optional.ofNullable(this.resolveConfiguration);
}
/**
* @return The severity of the alerts fired by the rule. Must be between 0 and 4.
*
*/
public Optional severity() {
return Optional.ofNullable(this.severity);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(PrometheusRuleResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List actions;
private @Nullable String alert;
private @Nullable Map annotations;
private @Nullable Boolean enabled;
private String expression;
private @Nullable String for_;
private @Nullable Map labels;
private @Nullable String record;
private @Nullable PrometheusRuleResolveConfigurationResponse resolveConfiguration;
private @Nullable Integer severity;
public Builder() {}
public Builder(PrometheusRuleResponse defaults) {
Objects.requireNonNull(defaults);
this.actions = defaults.actions;
this.alert = defaults.alert;
this.annotations = defaults.annotations;
this.enabled = defaults.enabled;
this.expression = defaults.expression;
this.for_ = defaults.for_;
this.labels = defaults.labels;
this.record = defaults.record;
this.resolveConfiguration = defaults.resolveConfiguration;
this.severity = defaults.severity;
}
@CustomType.Setter
public Builder actions(@Nullable List actions) {
this.actions = actions;
return this;
}
public Builder actions(PrometheusRuleGroupActionResponse... actions) {
return actions(List.of(actions));
}
@CustomType.Setter
public Builder alert(@Nullable String alert) {
this.alert = alert;
return this;
}
@CustomType.Setter
public Builder annotations(@Nullable Map annotations) {
this.annotations = annotations;
return this;
}
@CustomType.Setter
public Builder enabled(@Nullable Boolean enabled) {
this.enabled = enabled;
return this;
}
@CustomType.Setter
public Builder expression(String expression) {
if (expression == null) {
throw new MissingRequiredPropertyException("PrometheusRuleResponse", "expression");
}
this.expression = expression;
return this;
}
@CustomType.Setter("for")
public Builder for_(@Nullable String for_) {
this.for_ = for_;
return this;
}
@CustomType.Setter
public Builder labels(@Nullable Map labels) {
this.labels = labels;
return this;
}
@CustomType.Setter
public Builder record(@Nullable String record) {
this.record = record;
return this;
}
@CustomType.Setter
public Builder resolveConfiguration(@Nullable PrometheusRuleResolveConfigurationResponse resolveConfiguration) {
this.resolveConfiguration = resolveConfiguration;
return this;
}
@CustomType.Setter
public Builder severity(@Nullable Integer severity) {
this.severity = severity;
return this;
}
public PrometheusRuleResponse build() {
final var _resultValue = new PrometheusRuleResponse();
_resultValue.actions = actions;
_resultValue.alert = alert;
_resultValue.annotations = annotations;
_resultValue.enabled = enabled;
_resultValue.expression = expression;
_resultValue.for_ = for_;
_resultValue.labels = labels;
_resultValue.record = record;
_resultValue.resolveConfiguration = resolveConfiguration;
_resultValue.severity = severity;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy