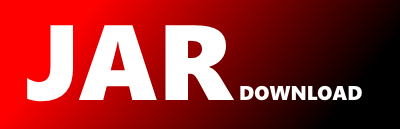
com.pulumi.azurenative.apicenter.ApicenterFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apicenter;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.apicenter.inputs.GetApiArgs;
import com.pulumi.azurenative.apicenter.inputs.GetApiDefinitionArgs;
import com.pulumi.azurenative.apicenter.inputs.GetApiDefinitionPlainArgs;
import com.pulumi.azurenative.apicenter.inputs.GetApiPlainArgs;
import com.pulumi.azurenative.apicenter.inputs.GetApiVersionArgs;
import com.pulumi.azurenative.apicenter.inputs.GetApiVersionPlainArgs;
import com.pulumi.azurenative.apicenter.inputs.GetDeploymentArgs;
import com.pulumi.azurenative.apicenter.inputs.GetDeploymentPlainArgs;
import com.pulumi.azurenative.apicenter.inputs.GetEnvironmentArgs;
import com.pulumi.azurenative.apicenter.inputs.GetEnvironmentPlainArgs;
import com.pulumi.azurenative.apicenter.inputs.GetMetadataSchemaArgs;
import com.pulumi.azurenative.apicenter.inputs.GetMetadataSchemaPlainArgs;
import com.pulumi.azurenative.apicenter.inputs.GetServiceArgs;
import com.pulumi.azurenative.apicenter.inputs.GetServicePlainArgs;
import com.pulumi.azurenative.apicenter.inputs.GetWorkspaceArgs;
import com.pulumi.azurenative.apicenter.inputs.GetWorkspacePlainArgs;
import com.pulumi.azurenative.apicenter.outputs.GetApiDefinitionResult;
import com.pulumi.azurenative.apicenter.outputs.GetApiResult;
import com.pulumi.azurenative.apicenter.outputs.GetApiVersionResult;
import com.pulumi.azurenative.apicenter.outputs.GetDeploymentResult;
import com.pulumi.azurenative.apicenter.outputs.GetEnvironmentResult;
import com.pulumi.azurenative.apicenter.outputs.GetMetadataSchemaResult;
import com.pulumi.azurenative.apicenter.outputs.GetServiceResult;
import com.pulumi.azurenative.apicenter.outputs.GetWorkspaceResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class ApicenterFunctions {
/**
* Returns details of the API.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getApi(GetApiArgs args) {
return getApi(args, InvokeOptions.Empty);
}
/**
* Returns details of the API.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getApiPlain(GetApiPlainArgs args) {
return getApiPlain(args, InvokeOptions.Empty);
}
/**
* Returns details of the API.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getApi(GetApiArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:apicenter:getApi", TypeShape.of(GetApiResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the API.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getApiPlain(GetApiPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:apicenter:getApi", TypeShape.of(GetApiResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the API definition.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getApiDefinition(GetApiDefinitionArgs args) {
return getApiDefinition(args, InvokeOptions.Empty);
}
/**
* Returns details of the API definition.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getApiDefinitionPlain(GetApiDefinitionPlainArgs args) {
return getApiDefinitionPlain(args, InvokeOptions.Empty);
}
/**
* Returns details of the API definition.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getApiDefinition(GetApiDefinitionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:apicenter:getApiDefinition", TypeShape.of(GetApiDefinitionResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the API definition.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getApiDefinitionPlain(GetApiDefinitionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:apicenter:getApiDefinition", TypeShape.of(GetApiDefinitionResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the API version.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getApiVersion(GetApiVersionArgs args) {
return getApiVersion(args, InvokeOptions.Empty);
}
/**
* Returns details of the API version.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getApiVersionPlain(GetApiVersionPlainArgs args) {
return getApiVersionPlain(args, InvokeOptions.Empty);
}
/**
* Returns details of the API version.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getApiVersion(GetApiVersionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:apicenter:getApiVersion", TypeShape.of(GetApiVersionResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the API version.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getApiVersionPlain(GetApiVersionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:apicenter:getApiVersion", TypeShape.of(GetApiVersionResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the API deployment.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getDeployment(GetDeploymentArgs args) {
return getDeployment(args, InvokeOptions.Empty);
}
/**
* Returns details of the API deployment.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getDeploymentPlain(GetDeploymentPlainArgs args) {
return getDeploymentPlain(args, InvokeOptions.Empty);
}
/**
* Returns details of the API deployment.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getDeployment(GetDeploymentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:apicenter:getDeployment", TypeShape.of(GetDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the API deployment.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getDeploymentPlain(GetDeploymentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:apicenter:getDeployment", TypeShape.of(GetDeploymentResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the environment.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getEnvironment(GetEnvironmentArgs args) {
return getEnvironment(args, InvokeOptions.Empty);
}
/**
* Returns details of the environment.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getEnvironmentPlain(GetEnvironmentPlainArgs args) {
return getEnvironmentPlain(args, InvokeOptions.Empty);
}
/**
* Returns details of the environment.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getEnvironment(GetEnvironmentArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:apicenter:getEnvironment", TypeShape.of(GetEnvironmentResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the environment.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getEnvironmentPlain(GetEnvironmentPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:apicenter:getEnvironment", TypeShape.of(GetEnvironmentResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the metadata schema.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getMetadataSchema(GetMetadataSchemaArgs args) {
return getMetadataSchema(args, InvokeOptions.Empty);
}
/**
* Returns details of the metadata schema.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getMetadataSchemaPlain(GetMetadataSchemaPlainArgs args) {
return getMetadataSchemaPlain(args, InvokeOptions.Empty);
}
/**
* Returns details of the metadata schema.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getMetadataSchema(GetMetadataSchemaArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:apicenter:getMetadataSchema", TypeShape.of(GetMetadataSchemaResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the metadata schema.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getMetadataSchemaPlain(GetMetadataSchemaPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:apicenter:getMetadataSchema", TypeShape.of(GetMetadataSchemaResult.class), args, Utilities.withVersion(options));
}
/**
* Get service
* Azure REST API version: 2023-07-01-preview.
*
* Other available API versions: 2024-03-01, 2024-03-15-preview.
*
*/
public static Output getService(GetServiceArgs args) {
return getService(args, InvokeOptions.Empty);
}
/**
* Get service
* Azure REST API version: 2023-07-01-preview.
*
* Other available API versions: 2024-03-01, 2024-03-15-preview.
*
*/
public static CompletableFuture getServicePlain(GetServicePlainArgs args) {
return getServicePlain(args, InvokeOptions.Empty);
}
/**
* Get service
* Azure REST API version: 2023-07-01-preview.
*
* Other available API versions: 2024-03-01, 2024-03-15-preview.
*
*/
public static Output getService(GetServiceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:apicenter:getService", TypeShape.of(GetServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Get service
* Azure REST API version: 2023-07-01-preview.
*
* Other available API versions: 2024-03-01, 2024-03-15-preview.
*
*/
public static CompletableFuture getServicePlain(GetServicePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:apicenter:getService", TypeShape.of(GetServiceResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the workspace.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getWorkspace(GetWorkspaceArgs args) {
return getWorkspace(args, InvokeOptions.Empty);
}
/**
* Returns details of the workspace.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getWorkspacePlain(GetWorkspacePlainArgs args) {
return getWorkspacePlain(args, InvokeOptions.Empty);
}
/**
* Returns details of the workspace.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static Output getWorkspace(GetWorkspaceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:apicenter:getWorkspace", TypeShape.of(GetWorkspaceResult.class), args, Utilities.withVersion(options));
}
/**
* Returns details of the workspace.
* Azure REST API version: 2024-03-01.
*
* Other available API versions: 2024-03-15-preview.
*
*/
public static CompletableFuture getWorkspacePlain(GetWorkspacePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:apicenter:getWorkspace", TypeShape.of(GetWorkspaceResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy