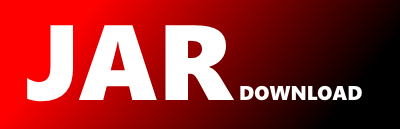
com.pulumi.azurenative.apimanagement.ApiArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement;
import com.pulumi.azurenative.apimanagement.enums.ApiType;
import com.pulumi.azurenative.apimanagement.enums.ContentFormat;
import com.pulumi.azurenative.apimanagement.enums.Protocol;
import com.pulumi.azurenative.apimanagement.enums.SoapApiType;
import com.pulumi.azurenative.apimanagement.enums.TranslateRequiredQueryParametersConduct;
import com.pulumi.azurenative.apimanagement.inputs.ApiContactInformationArgs;
import com.pulumi.azurenative.apimanagement.inputs.ApiCreateOrUpdatePropertiesWsdlSelectorArgs;
import com.pulumi.azurenative.apimanagement.inputs.ApiLicenseInformationArgs;
import com.pulumi.azurenative.apimanagement.inputs.ApiVersionSetContractDetailsArgs;
import com.pulumi.azurenative.apimanagement.inputs.AuthenticationSettingsContractArgs;
import com.pulumi.azurenative.apimanagement.inputs.SubscriptionKeyParameterNamesContractArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ApiArgs extends com.pulumi.resources.ResourceArgs {
public static final ApiArgs Empty = new ApiArgs();
/**
* API revision identifier. Must be unique in the current API Management service instance. Non-current revision has ;rev=n as a suffix where n is the revision number.
*
*/
@Import(name="apiId")
private @Nullable Output apiId;
/**
* @return API revision identifier. Must be unique in the current API Management service instance. Non-current revision has ;rev=n as a suffix where n is the revision number.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy