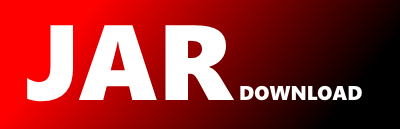
com.pulumi.azurenative.apimanagement.Subscription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.apimanagement;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.apimanagement.SubscriptionArgs;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Subscription details.
* Azure REST API version: 2022-08-01. Prior API version in Azure Native 1.x: 2020-12-01.
*
* Other available API versions: 2016-07-07, 2016-10-10, 2018-01-01, 2019-01-01, 2022-09-01-preview, 2023-03-01-preview, 2023-05-01-preview, 2023-09-01-preview, 2024-05-01.
*
* ## Example Usage
* ### ApiManagementCreateSubscription
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.apimanagement.Subscription;
* import com.pulumi.azurenative.apimanagement.SubscriptionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var subscription = new Subscription("subscription", SubscriptionArgs.builder()
* .displayName("testsub")
* .ownerId("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.ApiManagement/service/apimService1/users/57127d485157a511ace86ae7")
* .resourceGroupName("rg1")
* .scope("/subscriptions/subid/resourceGroups/rg1/providers/Microsoft.ApiManagement/service/apimService1/products/5600b59475ff190048060002")
* .serviceName("apimService1")
* .sid("testsub")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:apimanagement:Subscription testsub /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ApiManagement/service/{serviceName}/subscriptions/{sid}
* ```
*
*/
@ResourceType(type="azure-native:apimanagement:Subscription")
public class Subscription extends com.pulumi.resources.CustomResource {
/**
* Determines whether tracing is enabled
*
*/
@Export(name="allowTracing", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> allowTracing;
/**
* @return Determines whether tracing is enabled
*
*/
public Output> allowTracing() {
return Codegen.optional(this.allowTracing);
}
/**
* Subscription creation date. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
@Export(name="createdDate", refs={String.class}, tree="[0]")
private Output createdDate;
/**
* @return Subscription creation date. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Output createdDate() {
return this.createdDate;
}
/**
* The name of the subscription, or null if the subscription has no name.
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> displayName;
/**
* @return The name of the subscription, or null if the subscription has no name.
*
*/
public Output> displayName() {
return Codegen.optional(this.displayName);
}
/**
* Date when subscription was cancelled or expired. The setting is for audit purposes only and the subscription is not automatically cancelled. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
@Export(name="endDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> endDate;
/**
* @return Date when subscription was cancelled or expired. The setting is for audit purposes only and the subscription is not automatically cancelled. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Output> endDate() {
return Codegen.optional(this.endDate);
}
/**
* Subscription expiration date. The setting is for audit purposes only and the subscription is not automatically expired. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
@Export(name="expirationDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> expirationDate;
/**
* @return Subscription expiration date. The setting is for audit purposes only and the subscription is not automatically expired. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Output> expirationDate() {
return Codegen.optional(this.expirationDate);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Upcoming subscription expiration notification date. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
@Export(name="notificationDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> notificationDate;
/**
* @return Upcoming subscription expiration notification date. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Output> notificationDate() {
return Codegen.optional(this.notificationDate);
}
/**
* The user resource identifier of the subscription owner. The value is a valid relative URL in the format of /users/{userId} where {userId} is a user identifier.
*
*/
@Export(name="ownerId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> ownerId;
/**
* @return The user resource identifier of the subscription owner. The value is a valid relative URL in the format of /users/{userId} where {userId} is a user identifier.
*
*/
public Output> ownerId() {
return Codegen.optional(this.ownerId);
}
/**
* Subscription primary key. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*
*/
@Export(name="primaryKey", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> primaryKey;
/**
* @return Subscription primary key. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*
*/
public Output> primaryKey() {
return Codegen.optional(this.primaryKey);
}
/**
* Scope like /products/{productId} or /apis or /apis/{apiId}.
*
*/
@Export(name="scope", refs={String.class}, tree="[0]")
private Output scope;
/**
* @return Scope like /products/{productId} or /apis or /apis/{apiId}.
*
*/
public Output scope() {
return this.scope;
}
/**
* Subscription secondary key. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*
*/
@Export(name="secondaryKey", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> secondaryKey;
/**
* @return Subscription secondary key. This property will not be filled on 'GET' operations! Use '/listSecrets' POST request to get the value.
*
*/
public Output> secondaryKey() {
return Codegen.optional(this.secondaryKey);
}
/**
* Subscription activation date. The setting is for audit purposes only and the subscription is not automatically activated. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
@Export(name="startDate", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> startDate;
/**
* @return Subscription activation date. The setting is for audit purposes only and the subscription is not automatically activated. The subscription lifecycle can be managed by using the `state` property. The date conforms to the following format: `yyyy-MM-ddTHH:mm:ssZ` as specified by the ISO 8601 standard.
*
*/
public Output> startDate() {
return Codegen.optional(this.startDate);
}
/**
* Subscription state. Possible states are * active – the subscription is active, * suspended – the subscription is blocked, and the subscriber cannot call any APIs of the product, * submitted – the subscription request has been made by the developer, but has not yet been approved or rejected, * rejected – the subscription request has been denied by an administrator, * cancelled – the subscription has been cancelled by the developer or administrator, * expired – the subscription reached its expiration date and was deactivated.
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output state;
/**
* @return Subscription state. Possible states are * active – the subscription is active, * suspended – the subscription is blocked, and the subscriber cannot call any APIs of the product, * submitted – the subscription request has been made by the developer, but has not yet been approved or rejected, * rejected – the subscription request has been denied by an administrator, * cancelled – the subscription has been cancelled by the developer or administrator, * expired – the subscription reached its expiration date and was deactivated.
*
*/
public Output state() {
return this.state;
}
/**
* Optional subscription comment added by an administrator when the state is changed to the 'rejected'.
*
*/
@Export(name="stateComment", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> stateComment;
/**
* @return Optional subscription comment added by an administrator when the state is changed to the 'rejected'.
*
*/
public Output> stateComment() {
return Codegen.optional(this.stateComment);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Subscription(java.lang.String name) {
this(name, SubscriptionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Subscription(java.lang.String name, SubscriptionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Subscription(java.lang.String name, SubscriptionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:apimanagement:Subscription", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Subscription(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:apimanagement:Subscription", name, null, makeResourceOptions(options, id), false);
}
private static SubscriptionArgs makeArgs(SubscriptionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? SubscriptionArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:apimanagement/v20160707:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20161010:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20170301:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20180101:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20180601preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20190101:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20191201:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20191201preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20200601preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20201201:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20210101preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20210401preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20210801:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20211201preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20220401preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20220801:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20220901preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20230301preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20230501preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20230901preview:Subscription").build()),
Output.of(Alias.builder().type("azure-native:apimanagement/v20240501:Subscription").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Subscription get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Subscription(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy