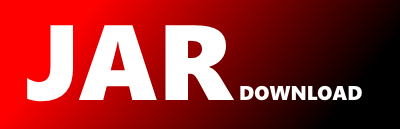
com.pulumi.azurenative.app.inputs.VnetConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.inputs;
import com.pulumi.azurenative.app.inputs.ManagedEnvironmentOutboundSettingsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Configuration properties for apps environment to join a Virtual Network
*
*/
public final class VnetConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final VnetConfigurationArgs Empty = new VnetConfigurationArgs();
/**
* CIDR notation IP range assigned to the Docker bridge, network. Must not overlap with any other provided IP ranges.
*
*/
@Import(name="dockerBridgeCidr")
private @Nullable Output dockerBridgeCidr;
/**
* @return CIDR notation IP range assigned to the Docker bridge, network. Must not overlap with any other provided IP ranges.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy