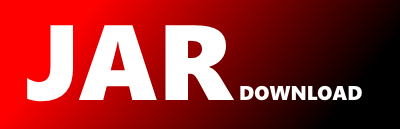
com.pulumi.azurenative.app.outputs.HttpRetryPolicyResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.app.outputs;
import com.pulumi.azurenative.app.outputs.HeaderMatchResponse;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class HttpRetryPolicyResponse {
/**
* @return Errors that can trigger a retry
*
*/
private @Nullable List errors;
/**
* @return Headers that must be present for a request to be retried
*
*/
private @Nullable List headers;
/**
* @return Additional http status codes that can trigger a retry
*
*/
private @Nullable List httpStatusCodes;
/**
* @return Initial delay, in milliseconds, before retrying a request
*
*/
private @Nullable Double initialDelayInMilliseconds;
/**
* @return Maximum interval, in milliseconds, between retries
*
*/
private @Nullable Double maxIntervalInMilliseconds;
/**
* @return Maximum number of times a request will retry
*
*/
private @Nullable Integer maxRetries;
private HttpRetryPolicyResponse() {}
/**
* @return Errors that can trigger a retry
*
*/
public List errors() {
return this.errors == null ? List.of() : this.errors;
}
/**
* @return Headers that must be present for a request to be retried
*
*/
public List headers() {
return this.headers == null ? List.of() : this.headers;
}
/**
* @return Additional http status codes that can trigger a retry
*
*/
public List httpStatusCodes() {
return this.httpStatusCodes == null ? List.of() : this.httpStatusCodes;
}
/**
* @return Initial delay, in milliseconds, before retrying a request
*
*/
public Optional initialDelayInMilliseconds() {
return Optional.ofNullable(this.initialDelayInMilliseconds);
}
/**
* @return Maximum interval, in milliseconds, between retries
*
*/
public Optional maxIntervalInMilliseconds() {
return Optional.ofNullable(this.maxIntervalInMilliseconds);
}
/**
* @return Maximum number of times a request will retry
*
*/
public Optional maxRetries() {
return Optional.ofNullable(this.maxRetries);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(HttpRetryPolicyResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List errors;
private @Nullable List headers;
private @Nullable List httpStatusCodes;
private @Nullable Double initialDelayInMilliseconds;
private @Nullable Double maxIntervalInMilliseconds;
private @Nullable Integer maxRetries;
public Builder() {}
public Builder(HttpRetryPolicyResponse defaults) {
Objects.requireNonNull(defaults);
this.errors = defaults.errors;
this.headers = defaults.headers;
this.httpStatusCodes = defaults.httpStatusCodes;
this.initialDelayInMilliseconds = defaults.initialDelayInMilliseconds;
this.maxIntervalInMilliseconds = defaults.maxIntervalInMilliseconds;
this.maxRetries = defaults.maxRetries;
}
@CustomType.Setter
public Builder errors(@Nullable List errors) {
this.errors = errors;
return this;
}
public Builder errors(String... errors) {
return errors(List.of(errors));
}
@CustomType.Setter
public Builder headers(@Nullable List headers) {
this.headers = headers;
return this;
}
public Builder headers(HeaderMatchResponse... headers) {
return headers(List.of(headers));
}
@CustomType.Setter
public Builder httpStatusCodes(@Nullable List httpStatusCodes) {
this.httpStatusCodes = httpStatusCodes;
return this;
}
public Builder httpStatusCodes(Integer... httpStatusCodes) {
return httpStatusCodes(List.of(httpStatusCodes));
}
@CustomType.Setter
public Builder initialDelayInMilliseconds(@Nullable Double initialDelayInMilliseconds) {
this.initialDelayInMilliseconds = initialDelayInMilliseconds;
return this;
}
@CustomType.Setter
public Builder maxIntervalInMilliseconds(@Nullable Double maxIntervalInMilliseconds) {
this.maxIntervalInMilliseconds = maxIntervalInMilliseconds;
return this;
}
@CustomType.Setter
public Builder maxRetries(@Nullable Integer maxRetries) {
this.maxRetries = maxRetries;
return this;
}
public HttpRetryPolicyResponse build() {
final var _resultValue = new HttpRetryPolicyResponse();
_resultValue.errors = errors;
_resultValue.headers = headers;
_resultValue.httpStatusCodes = httpStatusCodes;
_resultValue.initialDelayInMilliseconds = initialDelayInMilliseconds;
_resultValue.maxIntervalInMilliseconds = maxIntervalInMilliseconds;
_resultValue.maxRetries = maxRetries;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy