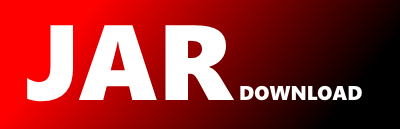
com.pulumi.azurenative.appconfiguration.KeyValueArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.appconfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class KeyValueArgs extends com.pulumi.resources.ResourceArgs {
public static final KeyValueArgs Empty = new KeyValueArgs();
/**
* The name of the configuration store.
*
*/
@Import(name="configStoreName", required=true)
private Output configStoreName;
/**
* @return The name of the configuration store.
*
*/
public Output configStoreName() {
return this.configStoreName;
}
/**
* The content type of the key-value's value.
* Providing a proper content-type can enable transformations of values when they are retrieved by applications.
*
*/
@Import(name="contentType")
private @Nullable Output contentType;
/**
* @return The content type of the key-value's value.
* Providing a proper content-type can enable transformations of values when they are retrieved by applications.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy