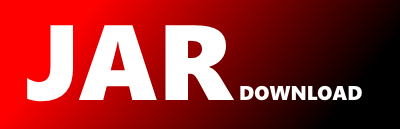
com.pulumi.azurenative.appplatform.outputs.NetworkProfileResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.appplatform.outputs;
import com.pulumi.azurenative.appplatform.outputs.IngressConfigResponse;
import com.pulumi.azurenative.appplatform.outputs.NetworkProfileResponseOutboundIPs;
import com.pulumi.azurenative.appplatform.outputs.RequiredTrafficResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class NetworkProfileResponse {
/**
* @return Name of the resource group containing network resources for customer apps in Azure Spring Apps
*
*/
private @Nullable String appNetworkResourceGroup;
/**
* @return Fully qualified resource Id of the subnet to host customer apps in Azure Spring Apps
*
*/
private @Nullable String appSubnetId;
/**
* @return Ingress configuration payload for Azure Spring Apps resource.
*
*/
private @Nullable IngressConfigResponse ingressConfig;
/**
* @return Desired outbound IP resources for Azure Spring Apps resource.
*
*/
private NetworkProfileResponseOutboundIPs outboundIPs;
/**
* @return The egress traffic type of Azure Spring Apps VNet instances.
*
*/
private @Nullable String outboundType;
/**
* @return Required inbound or outbound traffics for Azure Spring Apps resource.
*
*/
private List requiredTraffics;
/**
* @return Azure Spring Apps service reserved CIDR
*
*/
private @Nullable String serviceCidr;
/**
* @return Name of the resource group containing network resources of Azure Spring Apps Service Runtime
*
*/
private @Nullable String serviceRuntimeNetworkResourceGroup;
/**
* @return Fully qualified resource Id of the subnet to host Azure Spring Apps Service Runtime
*
*/
private @Nullable String serviceRuntimeSubnetId;
private NetworkProfileResponse() {}
/**
* @return Name of the resource group containing network resources for customer apps in Azure Spring Apps
*
*/
public Optional appNetworkResourceGroup() {
return Optional.ofNullable(this.appNetworkResourceGroup);
}
/**
* @return Fully qualified resource Id of the subnet to host customer apps in Azure Spring Apps
*
*/
public Optional appSubnetId() {
return Optional.ofNullable(this.appSubnetId);
}
/**
* @return Ingress configuration payload for Azure Spring Apps resource.
*
*/
public Optional ingressConfig() {
return Optional.ofNullable(this.ingressConfig);
}
/**
* @return Desired outbound IP resources for Azure Spring Apps resource.
*
*/
public NetworkProfileResponseOutboundIPs outboundIPs() {
return this.outboundIPs;
}
/**
* @return The egress traffic type of Azure Spring Apps VNet instances.
*
*/
public Optional outboundType() {
return Optional.ofNullable(this.outboundType);
}
/**
* @return Required inbound or outbound traffics for Azure Spring Apps resource.
*
*/
public List requiredTraffics() {
return this.requiredTraffics;
}
/**
* @return Azure Spring Apps service reserved CIDR
*
*/
public Optional serviceCidr() {
return Optional.ofNullable(this.serviceCidr);
}
/**
* @return Name of the resource group containing network resources of Azure Spring Apps Service Runtime
*
*/
public Optional serviceRuntimeNetworkResourceGroup() {
return Optional.ofNullable(this.serviceRuntimeNetworkResourceGroup);
}
/**
* @return Fully qualified resource Id of the subnet to host Azure Spring Apps Service Runtime
*
*/
public Optional serviceRuntimeSubnetId() {
return Optional.ofNullable(this.serviceRuntimeSubnetId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(NetworkProfileResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String appNetworkResourceGroup;
private @Nullable String appSubnetId;
private @Nullable IngressConfigResponse ingressConfig;
private NetworkProfileResponseOutboundIPs outboundIPs;
private @Nullable String outboundType;
private List requiredTraffics;
private @Nullable String serviceCidr;
private @Nullable String serviceRuntimeNetworkResourceGroup;
private @Nullable String serviceRuntimeSubnetId;
public Builder() {}
public Builder(NetworkProfileResponse defaults) {
Objects.requireNonNull(defaults);
this.appNetworkResourceGroup = defaults.appNetworkResourceGroup;
this.appSubnetId = defaults.appSubnetId;
this.ingressConfig = defaults.ingressConfig;
this.outboundIPs = defaults.outboundIPs;
this.outboundType = defaults.outboundType;
this.requiredTraffics = defaults.requiredTraffics;
this.serviceCidr = defaults.serviceCidr;
this.serviceRuntimeNetworkResourceGroup = defaults.serviceRuntimeNetworkResourceGroup;
this.serviceRuntimeSubnetId = defaults.serviceRuntimeSubnetId;
}
@CustomType.Setter
public Builder appNetworkResourceGroup(@Nullable String appNetworkResourceGroup) {
this.appNetworkResourceGroup = appNetworkResourceGroup;
return this;
}
@CustomType.Setter
public Builder appSubnetId(@Nullable String appSubnetId) {
this.appSubnetId = appSubnetId;
return this;
}
@CustomType.Setter
public Builder ingressConfig(@Nullable IngressConfigResponse ingressConfig) {
this.ingressConfig = ingressConfig;
return this;
}
@CustomType.Setter
public Builder outboundIPs(NetworkProfileResponseOutboundIPs outboundIPs) {
if (outboundIPs == null) {
throw new MissingRequiredPropertyException("NetworkProfileResponse", "outboundIPs");
}
this.outboundIPs = outboundIPs;
return this;
}
@CustomType.Setter
public Builder outboundType(@Nullable String outboundType) {
this.outboundType = outboundType;
return this;
}
@CustomType.Setter
public Builder requiredTraffics(List requiredTraffics) {
if (requiredTraffics == null) {
throw new MissingRequiredPropertyException("NetworkProfileResponse", "requiredTraffics");
}
this.requiredTraffics = requiredTraffics;
return this;
}
public Builder requiredTraffics(RequiredTrafficResponse... requiredTraffics) {
return requiredTraffics(List.of(requiredTraffics));
}
@CustomType.Setter
public Builder serviceCidr(@Nullable String serviceCidr) {
this.serviceCidr = serviceCidr;
return this;
}
@CustomType.Setter
public Builder serviceRuntimeNetworkResourceGroup(@Nullable String serviceRuntimeNetworkResourceGroup) {
this.serviceRuntimeNetworkResourceGroup = serviceRuntimeNetworkResourceGroup;
return this;
}
@CustomType.Setter
public Builder serviceRuntimeSubnetId(@Nullable String serviceRuntimeSubnetId) {
this.serviceRuntimeSubnetId = serviceRuntimeSubnetId;
return this;
}
public NetworkProfileResponse build() {
final var _resultValue = new NetworkProfileResponse();
_resultValue.appNetworkResourceGroup = appNetworkResourceGroup;
_resultValue.appSubnetId = appSubnetId;
_resultValue.ingressConfig = ingressConfig;
_resultValue.outboundIPs = outboundIPs;
_resultValue.outboundType = outboundType;
_resultValue.requiredTraffics = requiredTraffics;
_resultValue.serviceCidr = serviceCidr;
_resultValue.serviceRuntimeNetworkResourceGroup = serviceRuntimeNetworkResourceGroup;
_resultValue.serviceRuntimeSubnetId = serviceRuntimeSubnetId;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy