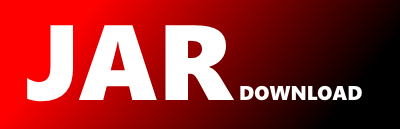
com.pulumi.azurenative.attestation.inputs.JSONWebKeyArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.attestation.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class JSONWebKeyArgs extends com.pulumi.resources.ResourceArgs {
public static final JSONWebKeyArgs Empty = new JSONWebKeyArgs();
/**
* The "alg" (algorithm) parameter identifies the algorithm intended for
* use with the key. The values used should either be registered in the
* IANA "JSON Web Signature and Encryption Algorithms" registry
* established by [JWA] or be a value that contains a Collision-
* Resistant Name.
*
*/
@Import(name="alg")
private @Nullable Output alg;
/**
* @return The "alg" (algorithm) parameter identifies the algorithm intended for
* use with the key. The values used should either be registered in the
* IANA "JSON Web Signature and Encryption Algorithms" registry
* established by [JWA] or be a value that contains a Collision-
* Resistant Name.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy