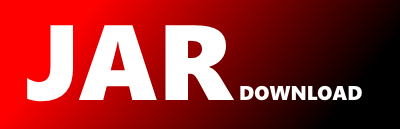
com.pulumi.azurenative.authorization.PolicyAssignmentArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.authorization;
import com.pulumi.azurenative.authorization.enums.EnforcementMode;
import com.pulumi.azurenative.authorization.inputs.IdentityArgs;
import com.pulumi.azurenative.authorization.inputs.NonComplianceMessageArgs;
import com.pulumi.azurenative.authorization.inputs.OverrideArgs;
import com.pulumi.azurenative.authorization.inputs.ParameterValuesValueArgs;
import com.pulumi.azurenative.authorization.inputs.ResourceSelectorArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PolicyAssignmentArgs extends com.pulumi.resources.ResourceArgs {
public static final PolicyAssignmentArgs Empty = new PolicyAssignmentArgs();
/**
* This message will be part of response in case of policy violation.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return This message will be part of response in case of policy violation.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy