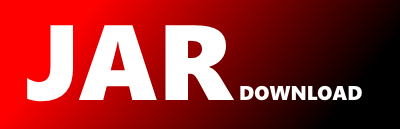
com.pulumi.azurenative.authorization.RoleAssignment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.authorization;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.authorization.RoleAssignmentArgs;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Role Assignments
* Azure REST API version: 2022-04-01. Prior API version in Azure Native 1.x: 2020-10-01-preview.
*
* Other available API versions: 2015-07-01, 2017-10-01-preview, 2020-03-01-preview, 2020-04-01-preview.
*
* ## Example Usage
* ### Create role assignment for resource
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.authorization.RoleAssignment;
* import com.pulumi.azurenative.authorization.RoleAssignmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var roleAssignment = new RoleAssignment("roleAssignment", RoleAssignmentArgs.builder()
* .principalId("ce2ce14e-85d7-4629-bdbc-454d0519d987")
* .principalType("User")
* .roleAssignmentName("05c5a614-a7d6-4502-b150-c2fb455033ff")
* .roleDefinitionId("/subscriptions/a925f2f7-5c63-4b7b-8799-25a5f97bc3b2/providers/Microsoft.Authorization/roleDefinitions/0b5fe924-9a61-425c-96af-cfe6e287ca2d")
* .scope("subscriptions/a925f2f7-5c63-4b7b-8799-25a5f97bc3b2/resourceGroups/testrg/providers/Microsoft.DocumentDb/databaseAccounts/test-db-account")
* .build());
*
* }
* }
*
* }
*
* ### Create role assignment for resource group
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.authorization.RoleAssignment;
* import com.pulumi.azurenative.authorization.RoleAssignmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var roleAssignment = new RoleAssignment("roleAssignment", RoleAssignmentArgs.builder()
* .principalId("ce2ce14e-85d7-4629-bdbc-454d0519d987")
* .principalType("User")
* .roleAssignmentName("05c5a614-a7d6-4502-b150-c2fb455033ff")
* .roleDefinitionId("/subscriptions/a925f2f7-5c63-4b7b-8799-25a5f97bc3b2/providers/Microsoft.Authorization/roleDefinitions/0b5fe924-9a61-425c-96af-cfe6e287ca2d")
* .scope("subscriptions/a925f2f7-5c63-4b7b-8799-25a5f97bc3b2/resourceGroups/testrg")
* .build());
*
* }
* }
*
* }
*
* ### Create role assignment for subscription
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.authorization.RoleAssignment;
* import com.pulumi.azurenative.authorization.RoleAssignmentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var roleAssignment = new RoleAssignment("roleAssignment", RoleAssignmentArgs.builder()
* .principalId("ce2ce14e-85d7-4629-bdbc-454d0519d987")
* .principalType("User")
* .roleAssignmentName("05c5a614-a7d6-4502-b150-c2fb455033ff")
* .roleDefinitionId("/subscriptions/a925f2f7-5c63-4b7b-8799-25a5f97bc3b2/providers/Microsoft.Authorization/roleDefinitions/0b5fe924-9a61-425c-96af-cfe6e287ca2d")
* .scope("subscriptions/a925f2f7-5c63-4b7b-8799-25a5f97bc3b2")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:authorization:RoleAssignment 05c5a614-a7d6-4502-b150-c2fb455033ff /{scope}/providers/Microsoft.Authorization/roleAssignments/{roleAssignmentName}
* ```
*
*/
@ResourceType(type="azure-native:authorization:RoleAssignment")
public class RoleAssignment extends com.pulumi.resources.CustomResource {
/**
* The conditions on the role assignment. This limits the resources it can be assigned to. e.g.: {@literal @}Resource[Microsoft.Storage/storageAccounts/blobServices/containers:ContainerName] StringEqualsIgnoreCase 'foo_storage_container'
*
*/
@Export(name="condition", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> condition;
/**
* @return The conditions on the role assignment. This limits the resources it can be assigned to. e.g.: {@literal @}Resource[Microsoft.Storage/storageAccounts/blobServices/containers:ContainerName] StringEqualsIgnoreCase 'foo_storage_container'
*
*/
public Output> condition() {
return Codegen.optional(this.condition);
}
/**
* Version of the condition. Currently the only accepted value is '2.0'
*
*/
@Export(name="conditionVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> conditionVersion;
/**
* @return Version of the condition. Currently the only accepted value is '2.0'
*
*/
public Output> conditionVersion() {
return Codegen.optional(this.conditionVersion);
}
/**
* Id of the user who created the assignment
*
*/
@Export(name="createdBy", refs={String.class}, tree="[0]")
private Output createdBy;
/**
* @return Id of the user who created the assignment
*
*/
public Output createdBy() {
return this.createdBy;
}
/**
* Time it was created
*
*/
@Export(name="createdOn", refs={String.class}, tree="[0]")
private Output createdOn;
/**
* @return Time it was created
*
*/
public Output createdOn() {
return this.createdOn;
}
/**
* Id of the delegated managed identity resource
*
*/
@Export(name="delegatedManagedIdentityResourceId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> delegatedManagedIdentityResourceId;
/**
* @return Id of the delegated managed identity resource
*
*/
public Output> delegatedManagedIdentityResourceId() {
return Codegen.optional(this.delegatedManagedIdentityResourceId);
}
/**
* Description of role assignment
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description of role assignment
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The role assignment name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The role assignment name.
*
*/
public Output name() {
return this.name;
}
/**
* The principal ID.
*
*/
@Export(name="principalId", refs={String.class}, tree="[0]")
private Output principalId;
/**
* @return The principal ID.
*
*/
public Output principalId() {
return this.principalId;
}
/**
* The principal type of the assigned principal ID.
*
*/
@Export(name="principalType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> principalType;
/**
* @return The principal type of the assigned principal ID.
*
*/
public Output> principalType() {
return Codegen.optional(this.principalType);
}
/**
* The role definition ID.
*
*/
@Export(name="roleDefinitionId", refs={String.class}, tree="[0]")
private Output roleDefinitionId;
/**
* @return The role definition ID.
*
*/
public Output roleDefinitionId() {
return this.roleDefinitionId;
}
/**
* The role assignment scope.
*
*/
@Export(name="scope", refs={String.class}, tree="[0]")
private Output scope;
/**
* @return The role assignment scope.
*
*/
public Output scope() {
return this.scope;
}
/**
* The role assignment type.
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The role assignment type.
*
*/
public Output type() {
return this.type;
}
/**
* Id of the user who updated the assignment
*
*/
@Export(name="updatedBy", refs={String.class}, tree="[0]")
private Output updatedBy;
/**
* @return Id of the user who updated the assignment
*
*/
public Output updatedBy() {
return this.updatedBy;
}
/**
* Time it was updated
*
*/
@Export(name="updatedOn", refs={String.class}, tree="[0]")
private Output updatedOn;
/**
* @return Time it was updated
*
*/
public Output updatedOn() {
return this.updatedOn;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public RoleAssignment(java.lang.String name) {
this(name, RoleAssignmentArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public RoleAssignment(java.lang.String name, RoleAssignmentArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public RoleAssignment(java.lang.String name, RoleAssignmentArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:authorization:RoleAssignment", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private RoleAssignment(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:authorization:RoleAssignment", name, null, makeResourceOptions(options, id), false);
}
private static RoleAssignmentArgs makeArgs(RoleAssignmentArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? RoleAssignmentArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:authorization/v20150701:RoleAssignment").build()),
Output.of(Alias.builder().type("azure-native:authorization/v20171001preview:RoleAssignment").build()),
Output.of(Alias.builder().type("azure-native:authorization/v20180101preview:RoleAssignment").build()),
Output.of(Alias.builder().type("azure-native:authorization/v20180901preview:RoleAssignment").build()),
Output.of(Alias.builder().type("azure-native:authorization/v20200301preview:RoleAssignment").build()),
Output.of(Alias.builder().type("azure-native:authorization/v20200401preview:RoleAssignment").build()),
Output.of(Alias.builder().type("azure-native:authorization/v20200801preview:RoleAssignment").build()),
Output.of(Alias.builder().type("azure-native:authorization/v20201001preview:RoleAssignment").build()),
Output.of(Alias.builder().type("azure-native:authorization/v20220401:RoleAssignment").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static RoleAssignment get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new RoleAssignment(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy