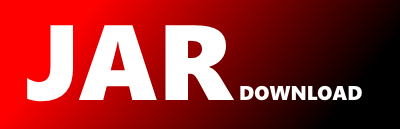
com.pulumi.azurenative.avs.outputs.IdentitySourceResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.avs.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class IdentitySourceResponse {
/**
* @return The domain's NetBIOS name
*
*/
private @Nullable String alias;
/**
* @return The base distinguished name for groups
*
*/
private @Nullable String baseGroupDN;
/**
* @return The base distinguished name for users
*
*/
private @Nullable String baseUserDN;
/**
* @return The domain's dns name
*
*/
private @Nullable String domain;
/**
* @return The name of the identity source
*
*/
private @Nullable String name;
/**
* @return The password of the Active Directory user with a minimum of read-only access to Base DN for users and groups.
*
*/
private @Nullable String password;
/**
* @return Primary server URL
*
*/
private @Nullable String primaryServer;
/**
* @return Secondary server URL
*
*/
private @Nullable String secondaryServer;
/**
* @return Protect LDAP communication using SSL certificate (LDAPS)
*
*/
private @Nullable String ssl;
/**
* @return The ID of an Active Directory user with a minimum of read-only access to Base DN for users and group
*
*/
private @Nullable String username;
private IdentitySourceResponse() {}
/**
* @return The domain's NetBIOS name
*
*/
public Optional alias() {
return Optional.ofNullable(this.alias);
}
/**
* @return The base distinguished name for groups
*
*/
public Optional baseGroupDN() {
return Optional.ofNullable(this.baseGroupDN);
}
/**
* @return The base distinguished name for users
*
*/
public Optional baseUserDN() {
return Optional.ofNullable(this.baseUserDN);
}
/**
* @return The domain's dns name
*
*/
public Optional domain() {
return Optional.ofNullable(this.domain);
}
/**
* @return The name of the identity source
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The password of the Active Directory user with a minimum of read-only access to Base DN for users and groups.
*
*/
public Optional password() {
return Optional.ofNullable(this.password);
}
/**
* @return Primary server URL
*
*/
public Optional primaryServer() {
return Optional.ofNullable(this.primaryServer);
}
/**
* @return Secondary server URL
*
*/
public Optional secondaryServer() {
return Optional.ofNullable(this.secondaryServer);
}
/**
* @return Protect LDAP communication using SSL certificate (LDAPS)
*
*/
public Optional ssl() {
return Optional.ofNullable(this.ssl);
}
/**
* @return The ID of an Active Directory user with a minimum of read-only access to Base DN for users and group
*
*/
public Optional username() {
return Optional.ofNullable(this.username);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IdentitySourceResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String alias;
private @Nullable String baseGroupDN;
private @Nullable String baseUserDN;
private @Nullable String domain;
private @Nullable String name;
private @Nullable String password;
private @Nullable String primaryServer;
private @Nullable String secondaryServer;
private @Nullable String ssl;
private @Nullable String username;
public Builder() {}
public Builder(IdentitySourceResponse defaults) {
Objects.requireNonNull(defaults);
this.alias = defaults.alias;
this.baseGroupDN = defaults.baseGroupDN;
this.baseUserDN = defaults.baseUserDN;
this.domain = defaults.domain;
this.name = defaults.name;
this.password = defaults.password;
this.primaryServer = defaults.primaryServer;
this.secondaryServer = defaults.secondaryServer;
this.ssl = defaults.ssl;
this.username = defaults.username;
}
@CustomType.Setter
public Builder alias(@Nullable String alias) {
this.alias = alias;
return this;
}
@CustomType.Setter
public Builder baseGroupDN(@Nullable String baseGroupDN) {
this.baseGroupDN = baseGroupDN;
return this;
}
@CustomType.Setter
public Builder baseUserDN(@Nullable String baseUserDN) {
this.baseUserDN = baseUserDN;
return this;
}
@CustomType.Setter
public Builder domain(@Nullable String domain) {
this.domain = domain;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder password(@Nullable String password) {
this.password = password;
return this;
}
@CustomType.Setter
public Builder primaryServer(@Nullable String primaryServer) {
this.primaryServer = primaryServer;
return this;
}
@CustomType.Setter
public Builder secondaryServer(@Nullable String secondaryServer) {
this.secondaryServer = secondaryServer;
return this;
}
@CustomType.Setter
public Builder ssl(@Nullable String ssl) {
this.ssl = ssl;
return this;
}
@CustomType.Setter
public Builder username(@Nullable String username) {
this.username = username;
return this;
}
public IdentitySourceResponse build() {
final var _resultValue = new IdentitySourceResponse();
_resultValue.alias = alias;
_resultValue.baseGroupDN = baseGroupDN;
_resultValue.baseUserDN = baseUserDN;
_resultValue.domain = domain;
_resultValue.name = name;
_resultValue.password = password;
_resultValue.primaryServer = primaryServer;
_resultValue.secondaryServer = secondaryServer;
_resultValue.ssl = ssl;
_resultValue.username = username;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy