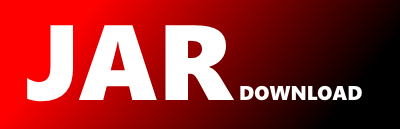
com.pulumi.azurenative.azurefleet.inputs.SpotPriorityProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurefleet.inputs;
import com.pulumi.azurenative.azurefleet.enums.EvictionPolicy;
import com.pulumi.azurenative.azurefleet.enums.SpotAllocationStrategy;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Configuration Options for Spot instances in Compute Fleet.
*
*/
public final class SpotPriorityProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final SpotPriorityProfileArgs Empty = new SpotPriorityProfileArgs();
/**
* Allocation strategy to follow when determining the VM sizes distribution for Spot VMs.
*
*/
@Import(name="allocationStrategy")
private @Nullable Output> allocationStrategy;
/**
* @return Allocation strategy to follow when determining the VM sizes distribution for Spot VMs.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy