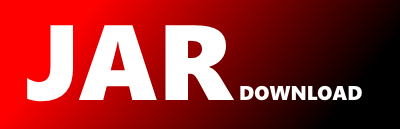
com.pulumi.azurenative.azurestack.outputs.GetProductResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurestack.outputs;
import com.pulumi.azurenative.azurestack.outputs.CompatibilityResponse;
import com.pulumi.azurenative.azurestack.outputs.IconUrisResponse;
import com.pulumi.azurenative.azurestack.outputs.ProductLinkResponse;
import com.pulumi.azurenative.azurestack.outputs.ProductPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetProductResult {
/**
* @return The part number used for billing purposes.
*
*/
private @Nullable String billingPartNumber;
/**
* @return Product compatibility with current device.
*
*/
private @Nullable CompatibilityResponse compatibility;
/**
* @return The description of the product.
*
*/
private @Nullable String description;
/**
* @return The display name of the product.
*
*/
private @Nullable String displayName;
/**
* @return The entity tag used for optimistic concurrency when modifying the resource.
*
*/
private @Nullable String etag;
/**
* @return The identifier of the gallery item corresponding to the product.
*
*/
private @Nullable String galleryItemIdentity;
/**
* @return Additional links available for this product.
*
*/
private @Nullable IconUrisResponse iconUris;
/**
* @return ID of the resource.
*
*/
private String id;
/**
* @return The legal terms.
*
*/
private @Nullable String legalTerms;
/**
* @return Additional links available for this product.
*
*/
private @Nullable List links;
/**
* @return Name of the resource.
*
*/
private String name;
/**
* @return The offer representing the product.
*
*/
private @Nullable String offer;
/**
* @return The version of the product offer.
*
*/
private @Nullable String offerVersion;
/**
* @return The length of product content.
*
*/
private @Nullable Double payloadLength;
/**
* @return The privacy policy.
*
*/
private @Nullable String privacyPolicy;
/**
* @return The kind of the product (virtualMachine or virtualMachineExtension)
*
*/
private @Nullable String productKind;
/**
* @return Additional properties for the product.
*
*/
private @Nullable ProductPropertiesResponse productProperties;
/**
* @return The user-friendly name of the product publisher.
*
*/
private @Nullable String publisherDisplayName;
/**
* @return Publisher identifier.
*
*/
private @Nullable String publisherIdentifier;
/**
* @return The product SKU.
*
*/
private @Nullable String sku;
/**
* @return Type of Resource.
*
*/
private String type;
/**
* @return The type of the Virtual Machine Extension.
*
*/
private @Nullable String vmExtensionType;
private GetProductResult() {}
/**
* @return The part number used for billing purposes.
*
*/
public Optional billingPartNumber() {
return Optional.ofNullable(this.billingPartNumber);
}
/**
* @return Product compatibility with current device.
*
*/
public Optional compatibility() {
return Optional.ofNullable(this.compatibility);
}
/**
* @return The description of the product.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return The display name of the product.
*
*/
public Optional displayName() {
return Optional.ofNullable(this.displayName);
}
/**
* @return The entity tag used for optimistic concurrency when modifying the resource.
*
*/
public Optional etag() {
return Optional.ofNullable(this.etag);
}
/**
* @return The identifier of the gallery item corresponding to the product.
*
*/
public Optional galleryItemIdentity() {
return Optional.ofNullable(this.galleryItemIdentity);
}
/**
* @return Additional links available for this product.
*
*/
public Optional iconUris() {
return Optional.ofNullable(this.iconUris);
}
/**
* @return ID of the resource.
*
*/
public String id() {
return this.id;
}
/**
* @return The legal terms.
*
*/
public Optional legalTerms() {
return Optional.ofNullable(this.legalTerms);
}
/**
* @return Additional links available for this product.
*
*/
public List links() {
return this.links == null ? List.of() : this.links;
}
/**
* @return Name of the resource.
*
*/
public String name() {
return this.name;
}
/**
* @return The offer representing the product.
*
*/
public Optional offer() {
return Optional.ofNullable(this.offer);
}
/**
* @return The version of the product offer.
*
*/
public Optional offerVersion() {
return Optional.ofNullable(this.offerVersion);
}
/**
* @return The length of product content.
*
*/
public Optional payloadLength() {
return Optional.ofNullable(this.payloadLength);
}
/**
* @return The privacy policy.
*
*/
public Optional privacyPolicy() {
return Optional.ofNullable(this.privacyPolicy);
}
/**
* @return The kind of the product (virtualMachine or virtualMachineExtension)
*
*/
public Optional productKind() {
return Optional.ofNullable(this.productKind);
}
/**
* @return Additional properties for the product.
*
*/
public Optional productProperties() {
return Optional.ofNullable(this.productProperties);
}
/**
* @return The user-friendly name of the product publisher.
*
*/
public Optional publisherDisplayName() {
return Optional.ofNullable(this.publisherDisplayName);
}
/**
* @return Publisher identifier.
*
*/
public Optional publisherIdentifier() {
return Optional.ofNullable(this.publisherIdentifier);
}
/**
* @return The product SKU.
*
*/
public Optional sku() {
return Optional.ofNullable(this.sku);
}
/**
* @return Type of Resource.
*
*/
public String type() {
return this.type;
}
/**
* @return The type of the Virtual Machine Extension.
*
*/
public Optional vmExtensionType() {
return Optional.ofNullable(this.vmExtensionType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetProductResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String billingPartNumber;
private @Nullable CompatibilityResponse compatibility;
private @Nullable String description;
private @Nullable String displayName;
private @Nullable String etag;
private @Nullable String galleryItemIdentity;
private @Nullable IconUrisResponse iconUris;
private String id;
private @Nullable String legalTerms;
private @Nullable List links;
private String name;
private @Nullable String offer;
private @Nullable String offerVersion;
private @Nullable Double payloadLength;
private @Nullable String privacyPolicy;
private @Nullable String productKind;
private @Nullable ProductPropertiesResponse productProperties;
private @Nullable String publisherDisplayName;
private @Nullable String publisherIdentifier;
private @Nullable String sku;
private String type;
private @Nullable String vmExtensionType;
public Builder() {}
public Builder(GetProductResult defaults) {
Objects.requireNonNull(defaults);
this.billingPartNumber = defaults.billingPartNumber;
this.compatibility = defaults.compatibility;
this.description = defaults.description;
this.displayName = defaults.displayName;
this.etag = defaults.etag;
this.galleryItemIdentity = defaults.galleryItemIdentity;
this.iconUris = defaults.iconUris;
this.id = defaults.id;
this.legalTerms = defaults.legalTerms;
this.links = defaults.links;
this.name = defaults.name;
this.offer = defaults.offer;
this.offerVersion = defaults.offerVersion;
this.payloadLength = defaults.payloadLength;
this.privacyPolicy = defaults.privacyPolicy;
this.productKind = defaults.productKind;
this.productProperties = defaults.productProperties;
this.publisherDisplayName = defaults.publisherDisplayName;
this.publisherIdentifier = defaults.publisherIdentifier;
this.sku = defaults.sku;
this.type = defaults.type;
this.vmExtensionType = defaults.vmExtensionType;
}
@CustomType.Setter
public Builder billingPartNumber(@Nullable String billingPartNumber) {
this.billingPartNumber = billingPartNumber;
return this;
}
@CustomType.Setter
public Builder compatibility(@Nullable CompatibilityResponse compatibility) {
this.compatibility = compatibility;
return this;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder displayName(@Nullable String displayName) {
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder etag(@Nullable String etag) {
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder galleryItemIdentity(@Nullable String galleryItemIdentity) {
this.galleryItemIdentity = galleryItemIdentity;
return this;
}
@CustomType.Setter
public Builder iconUris(@Nullable IconUrisResponse iconUris) {
this.iconUris = iconUris;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetProductResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder legalTerms(@Nullable String legalTerms) {
this.legalTerms = legalTerms;
return this;
}
@CustomType.Setter
public Builder links(@Nullable List links) {
this.links = links;
return this;
}
public Builder links(ProductLinkResponse... links) {
return links(List.of(links));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetProductResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder offer(@Nullable String offer) {
this.offer = offer;
return this;
}
@CustomType.Setter
public Builder offerVersion(@Nullable String offerVersion) {
this.offerVersion = offerVersion;
return this;
}
@CustomType.Setter
public Builder payloadLength(@Nullable Double payloadLength) {
this.payloadLength = payloadLength;
return this;
}
@CustomType.Setter
public Builder privacyPolicy(@Nullable String privacyPolicy) {
this.privacyPolicy = privacyPolicy;
return this;
}
@CustomType.Setter
public Builder productKind(@Nullable String productKind) {
this.productKind = productKind;
return this;
}
@CustomType.Setter
public Builder productProperties(@Nullable ProductPropertiesResponse productProperties) {
this.productProperties = productProperties;
return this;
}
@CustomType.Setter
public Builder publisherDisplayName(@Nullable String publisherDisplayName) {
this.publisherDisplayName = publisherDisplayName;
return this;
}
@CustomType.Setter
public Builder publisherIdentifier(@Nullable String publisherIdentifier) {
this.publisherIdentifier = publisherIdentifier;
return this;
}
@CustomType.Setter
public Builder sku(@Nullable String sku) {
this.sku = sku;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetProductResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder vmExtensionType(@Nullable String vmExtensionType) {
this.vmExtensionType = vmExtensionType;
return this;
}
public GetProductResult build() {
final var _resultValue = new GetProductResult();
_resultValue.billingPartNumber = billingPartNumber;
_resultValue.compatibility = compatibility;
_resultValue.description = description;
_resultValue.displayName = displayName;
_resultValue.etag = etag;
_resultValue.galleryItemIdentity = galleryItemIdentity;
_resultValue.iconUris = iconUris;
_resultValue.id = id;
_resultValue.legalTerms = legalTerms;
_resultValue.links = links;
_resultValue.name = name;
_resultValue.offer = offer;
_resultValue.offerVersion = offerVersion;
_resultValue.payloadLength = payloadLength;
_resultValue.privacyPolicy = privacyPolicy;
_resultValue.productKind = productKind;
_resultValue.productProperties = productProperties;
_resultValue.publisherDisplayName = publisherDisplayName;
_resultValue.publisherIdentifier = publisherIdentifier;
_resultValue.sku = sku;
_resultValue.type = type;
_resultValue.vmExtensionType = vmExtensionType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy