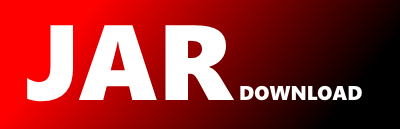
com.pulumi.azurenative.azurestackhci.UpdateArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurestackhci;
import com.pulumi.azurenative.azurestackhci.enums.AvailabilityType;
import com.pulumi.azurenative.azurestackhci.enums.State;
import com.pulumi.azurenative.azurestackhci.inputs.UpdatePrerequisiteArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class UpdateArgs extends com.pulumi.resources.ResourceArgs {
public static final UpdateArgs Empty = new UpdateArgs();
/**
* Extensible KV pairs serialized as a string. This is currently used to report the stamp OEM family and hardware model information when an update is flagged as Invalid for the stamp based on OEM type.
*
*/
@Import(name="additionalProperties")
private @Nullable Output additionalProperties;
/**
* @return Extensible KV pairs serialized as a string. This is currently used to report the stamp OEM family and hardware model information when an update is flagged as Invalid for the stamp based on OEM type.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy