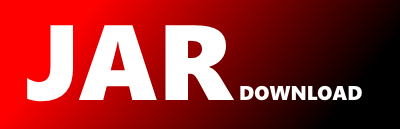
com.pulumi.azurenative.azurestackhci.outputs.NicDetailResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.azurestackhci.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class NicDetailResponse {
/**
* @return Adapter Name of NIC
*
*/
private String adapterName;
/**
* @return Component Id of NIC
*
*/
private @Nullable String componentId;
/**
* @return Default Gateway of NIC
*
*/
private @Nullable String defaultGateway;
/**
* @return Default Isolation of Management NIC
*
*/
private @Nullable String defaultIsolationId;
/**
* @return DNS Servers for NIC
*
*/
private @Nullable List dnsServers;
/**
* @return Driver Version of NIC
*
*/
private @Nullable String driverVersion;
/**
* @return Interface Description of NIC
*
*/
private @Nullable String interfaceDescription;
/**
* @return Subnet Mask of NIC
*
*/
private @Nullable String ip4Address;
/**
* @return Subnet Mask of NIC
*
*/
private @Nullable String subnetMask;
private NicDetailResponse() {}
/**
* @return Adapter Name of NIC
*
*/
public String adapterName() {
return this.adapterName;
}
/**
* @return Component Id of NIC
*
*/
public Optional componentId() {
return Optional.ofNullable(this.componentId);
}
/**
* @return Default Gateway of NIC
*
*/
public Optional defaultGateway() {
return Optional.ofNullable(this.defaultGateway);
}
/**
* @return Default Isolation of Management NIC
*
*/
public Optional defaultIsolationId() {
return Optional.ofNullable(this.defaultIsolationId);
}
/**
* @return DNS Servers for NIC
*
*/
public List dnsServers() {
return this.dnsServers == null ? List.of() : this.dnsServers;
}
/**
* @return Driver Version of NIC
*
*/
public Optional driverVersion() {
return Optional.ofNullable(this.driverVersion);
}
/**
* @return Interface Description of NIC
*
*/
public Optional interfaceDescription() {
return Optional.ofNullable(this.interfaceDescription);
}
/**
* @return Subnet Mask of NIC
*
*/
public Optional ip4Address() {
return Optional.ofNullable(this.ip4Address);
}
/**
* @return Subnet Mask of NIC
*
*/
public Optional subnetMask() {
return Optional.ofNullable(this.subnetMask);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(NicDetailResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String adapterName;
private @Nullable String componentId;
private @Nullable String defaultGateway;
private @Nullable String defaultIsolationId;
private @Nullable List dnsServers;
private @Nullable String driverVersion;
private @Nullable String interfaceDescription;
private @Nullable String ip4Address;
private @Nullable String subnetMask;
public Builder() {}
public Builder(NicDetailResponse defaults) {
Objects.requireNonNull(defaults);
this.adapterName = defaults.adapterName;
this.componentId = defaults.componentId;
this.defaultGateway = defaults.defaultGateway;
this.defaultIsolationId = defaults.defaultIsolationId;
this.dnsServers = defaults.dnsServers;
this.driverVersion = defaults.driverVersion;
this.interfaceDescription = defaults.interfaceDescription;
this.ip4Address = defaults.ip4Address;
this.subnetMask = defaults.subnetMask;
}
@CustomType.Setter
public Builder adapterName(String adapterName) {
if (adapterName == null) {
throw new MissingRequiredPropertyException("NicDetailResponse", "adapterName");
}
this.adapterName = adapterName;
return this;
}
@CustomType.Setter
public Builder componentId(@Nullable String componentId) {
this.componentId = componentId;
return this;
}
@CustomType.Setter
public Builder defaultGateway(@Nullable String defaultGateway) {
this.defaultGateway = defaultGateway;
return this;
}
@CustomType.Setter
public Builder defaultIsolationId(@Nullable String defaultIsolationId) {
this.defaultIsolationId = defaultIsolationId;
return this;
}
@CustomType.Setter
public Builder dnsServers(@Nullable List dnsServers) {
this.dnsServers = dnsServers;
return this;
}
public Builder dnsServers(String... dnsServers) {
return dnsServers(List.of(dnsServers));
}
@CustomType.Setter
public Builder driverVersion(@Nullable String driverVersion) {
this.driverVersion = driverVersion;
return this;
}
@CustomType.Setter
public Builder interfaceDescription(@Nullable String interfaceDescription) {
this.interfaceDescription = interfaceDescription;
return this;
}
@CustomType.Setter
public Builder ip4Address(@Nullable String ip4Address) {
this.ip4Address = ip4Address;
return this;
}
@CustomType.Setter
public Builder subnetMask(@Nullable String subnetMask) {
this.subnetMask = subnetMask;
return this;
}
public NicDetailResponse build() {
final var _resultValue = new NicDetailResponse();
_resultValue.adapterName = adapterName;
_resultValue.componentId = componentId;
_resultValue.defaultGateway = defaultGateway;
_resultValue.defaultIsolationId = defaultIsolationId;
_resultValue.dnsServers = dnsServers;
_resultValue.driverVersion = driverVersion;
_resultValue.interfaceDescription = interfaceDescription;
_resultValue.ip4Address = ip4Address;
_resultValue.subnetMask = subnetMask;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy