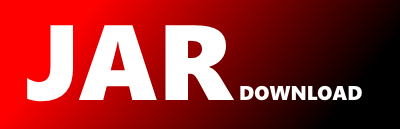
com.pulumi.azurenative.billing.inputs.BillingProfilePropertiesArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.billing.inputs;
import com.pulumi.azurenative.billing.inputs.AzurePlanArgs;
import com.pulumi.azurenative.billing.inputs.BillingProfilePropertiesBillToArgs;
import com.pulumi.azurenative.billing.inputs.BillingProfilePropertiesCurrentPaymentTermArgs;
import com.pulumi.azurenative.billing.inputs.BillingProfilePropertiesIndirectRelationshipInfoArgs;
import com.pulumi.azurenative.billing.inputs.BillingProfilePropertiesShipToArgs;
import com.pulumi.azurenative.billing.inputs.BillingProfilePropertiesSoldToArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A billing profile.
*
*/
public final class BillingProfilePropertiesArgs extends com.pulumi.resources.ResourceArgs {
public static final BillingProfilePropertiesArgs Empty = new BillingProfilePropertiesArgs();
/**
* Billing address.
*
*/
@Import(name="billTo")
private @Nullable Output billTo;
/**
* @return Billing address.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy