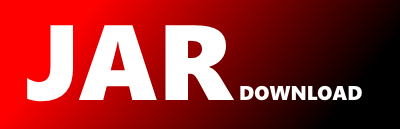
com.pulumi.azurenative.cache.Redis Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cache;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.cache.RedisArgs;
import com.pulumi.azurenative.cache.outputs.ManagedServiceIdentityResponse;
import com.pulumi.azurenative.cache.outputs.PrivateEndpointConnectionResponse;
import com.pulumi.azurenative.cache.outputs.RedisAccessKeysResponse;
import com.pulumi.azurenative.cache.outputs.RedisCommonPropertiesResponseRedisConfiguration;
import com.pulumi.azurenative.cache.outputs.RedisInstanceDetailsResponse;
import com.pulumi.azurenative.cache.outputs.RedisLinkedServerResponse;
import com.pulumi.azurenative.cache.outputs.SkuResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A single Redis item in List or Get Operation.
* Azure REST API version: 2023-04-01. Prior API version in Azure Native 1.x: 2020-06-01.
*
* Other available API versions: 2015-08-01, 2017-02-01, 2019-07-01, 2020-06-01, 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
*
* ## Example Usage
* ### RedisCacheCreate
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.cache.Redis;
* import com.pulumi.azurenative.cache.RedisArgs;
* import com.pulumi.azurenative.cache.inputs.RedisCommonPropertiesRedisConfigurationArgs;
* import com.pulumi.azurenative.cache.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var redis = new Redis("redis", RedisArgs.builder()
* .enableNonSslPort(true)
* .location("West US")
* .minimumTlsVersion("1.2")
* .name("cache1")
* .redisConfiguration(RedisCommonPropertiesRedisConfigurationArgs.builder()
* .maxmemoryPolicy("allkeys-lru")
* .build())
* .redisVersion("4")
* .replicasPerPrimary(2)
* .resourceGroupName("rg1")
* .shardCount(2)
* .sku(SkuArgs.builder()
* .capacity(1)
* .family("P")
* .name("Premium")
* .build())
* .staticIP("192.168.0.5")
* .subnetId("/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1")
* .zones("1")
* .build());
*
* }
* }
*
* }
*
* ### RedisCacheCreateDefaultVersion
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.cache.Redis;
* import com.pulumi.azurenative.cache.RedisArgs;
* import com.pulumi.azurenative.cache.inputs.RedisCommonPropertiesRedisConfigurationArgs;
* import com.pulumi.azurenative.cache.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var redis = new Redis("redis", RedisArgs.builder()
* .enableNonSslPort(true)
* .location("West US")
* .minimumTlsVersion("1.2")
* .name("cache1")
* .redisConfiguration(RedisCommonPropertiesRedisConfigurationArgs.builder()
* .maxmemoryPolicy("allkeys-lru")
* .build())
* .replicasPerPrimary(2)
* .resourceGroupName("rg1")
* .shardCount(2)
* .sku(SkuArgs.builder()
* .capacity(1)
* .family("P")
* .name("Premium")
* .build())
* .staticIP("192.168.0.5")
* .subnetId("/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1")
* .zones("1")
* .build());
*
* }
* }
*
* }
*
* ### RedisCacheCreateLatestVersion
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.cache.Redis;
* import com.pulumi.azurenative.cache.RedisArgs;
* import com.pulumi.azurenative.cache.inputs.RedisCommonPropertiesRedisConfigurationArgs;
* import com.pulumi.azurenative.cache.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var redis = new Redis("redis", RedisArgs.builder()
* .enableNonSslPort(true)
* .location("West US")
* .minimumTlsVersion("1.2")
* .name("cache1")
* .redisConfiguration(RedisCommonPropertiesRedisConfigurationArgs.builder()
* .maxmemoryPolicy("allkeys-lru")
* .build())
* .redisVersion("Latest")
* .replicasPerPrimary(2)
* .resourceGroupName("rg1")
* .shardCount(2)
* .sku(SkuArgs.builder()
* .capacity(1)
* .family("P")
* .name("Premium")
* .build())
* .staticIP("192.168.0.5")
* .subnetId("/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1")
* .zones("1")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:cache:Redis cache1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Cache/redis/{name}
* ```
*
*/
@ResourceType(type="azure-native:cache:Redis")
public class Redis extends com.pulumi.resources.CustomResource {
/**
* The keys of the Redis cache - not set if this object is not the response to Create or Update redis cache
*
*/
@Export(name="accessKeys", refs={RedisAccessKeysResponse.class}, tree="[0]")
private Output accessKeys;
/**
* @return The keys of the Redis cache - not set if this object is not the response to Create or Update redis cache
*
*/
public Output accessKeys() {
return this.accessKeys;
}
/**
* Specifies whether the non-ssl Redis server port (6379) is enabled.
*
*/
@Export(name="enableNonSslPort", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableNonSslPort;
/**
* @return Specifies whether the non-ssl Redis server port (6379) is enabled.
*
*/
public Output> enableNonSslPort() {
return Codegen.optional(this.enableNonSslPort);
}
/**
* Redis host name.
*
*/
@Export(name="hostName", refs={String.class}, tree="[0]")
private Output hostName;
/**
* @return Redis host name.
*
*/
public Output hostName() {
return this.hostName;
}
/**
* The identity of the resource.
*
*/
@Export(name="identity", refs={ManagedServiceIdentityResponse.class}, tree="[0]")
private Output* @Nullable */ ManagedServiceIdentityResponse> identity;
/**
* @return The identity of the resource.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* List of the Redis instances associated with the cache
*
*/
@Export(name="instances", refs={List.class,RedisInstanceDetailsResponse.class}, tree="[0,1]")
private Output> instances;
/**
* @return List of the Redis instances associated with the cache
*
*/
public Output> instances() {
return this.instances;
}
/**
* List of the linked servers associated with the cache
*
*/
@Export(name="linkedServers", refs={List.class,RedisLinkedServerResponse.class}, tree="[0,1]")
private Output> linkedServers;
/**
* @return List of the linked servers associated with the cache
*
*/
public Output> linkedServers() {
return this.linkedServers;
}
/**
* The geo-location where the resource lives
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The geo-location where the resource lives
*
*/
public Output location() {
return this.location;
}
/**
* Optional: requires clients to use a specified TLS version (or higher) to connect (e,g, '1.0', '1.1', '1.2')
*
*/
@Export(name="minimumTlsVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> minimumTlsVersion;
/**
* @return Optional: requires clients to use a specified TLS version (or higher) to connect (e,g, '1.0', '1.1', '1.2')
*
*/
public Output> minimumTlsVersion() {
return Codegen.optional(this.minimumTlsVersion);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Redis non-SSL port.
*
*/
@Export(name="port", refs={Integer.class}, tree="[0]")
private Output port;
/**
* @return Redis non-SSL port.
*
*/
public Output port() {
return this.port;
}
/**
* List of private endpoint connection associated with the specified redis cache
*
*/
@Export(name="privateEndpointConnections", refs={List.class,PrivateEndpointConnectionResponse.class}, tree="[0,1]")
private Output> privateEndpointConnections;
/**
* @return List of private endpoint connection associated with the specified redis cache
*
*/
public Output> privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* Redis instance provisioning status.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return Redis instance provisioning status.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Whether or not public endpoint access is allowed for this cache. Value is optional, but if passed in, must be 'Enabled' or 'Disabled'. If 'Disabled', private endpoints are the exclusive access method. Default value is 'Enabled'. Note: This setting is important for caches with private endpoints. It has *no effect* on caches that are joined to, or injected into, a virtual network subnet.
*
*/
@Export(name="publicNetworkAccess", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> publicNetworkAccess;
/**
* @return Whether or not public endpoint access is allowed for this cache. Value is optional, but if passed in, must be 'Enabled' or 'Disabled'. If 'Disabled', private endpoints are the exclusive access method. Default value is 'Enabled'. Note: This setting is important for caches with private endpoints. It has *no effect* on caches that are joined to, or injected into, a virtual network subnet.
*
*/
public Output> publicNetworkAccess() {
return Codegen.optional(this.publicNetworkAccess);
}
/**
* All Redis Settings. Few possible keys: rdb-backup-enabled,rdb-storage-connection-string,rdb-backup-frequency,maxmemory-delta,maxmemory-policy,notify-keyspace-events,maxmemory-samples,slowlog-log-slower-than,slowlog-max-len,list-max-ziplist-entries,list-max-ziplist-value,hash-max-ziplist-entries,hash-max-ziplist-value,set-max-intset-entries,zset-max-ziplist-entries,zset-max-ziplist-value etc.
*
*/
@Export(name="redisConfiguration", refs={RedisCommonPropertiesResponseRedisConfiguration.class}, tree="[0]")
private Output* @Nullable */ RedisCommonPropertiesResponseRedisConfiguration> redisConfiguration;
/**
* @return All Redis Settings. Few possible keys: rdb-backup-enabled,rdb-storage-connection-string,rdb-backup-frequency,maxmemory-delta,maxmemory-policy,notify-keyspace-events,maxmemory-samples,slowlog-log-slower-than,slowlog-max-len,list-max-ziplist-entries,list-max-ziplist-value,hash-max-ziplist-entries,hash-max-ziplist-value,set-max-intset-entries,zset-max-ziplist-entries,zset-max-ziplist-value etc.
*
*/
public Output> redisConfiguration() {
return Codegen.optional(this.redisConfiguration);
}
/**
* Redis version. This should be in the form 'major[.minor]' (only 'major' is required) or the value 'latest' which refers to the latest stable Redis version that is available. Supported versions: 4.0, 6.0 (latest). Default value is 'latest'.
*
*/
@Export(name="redisVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> redisVersion;
/**
* @return Redis version. This should be in the form 'major[.minor]' (only 'major' is required) or the value 'latest' which refers to the latest stable Redis version that is available. Supported versions: 4.0, 6.0 (latest). Default value is 'latest'.
*
*/
public Output> redisVersion() {
return Codegen.optional(this.redisVersion);
}
/**
* The number of replicas to be created per primary.
*
*/
@Export(name="replicasPerMaster", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> replicasPerMaster;
/**
* @return The number of replicas to be created per primary.
*
*/
public Output> replicasPerMaster() {
return Codegen.optional(this.replicasPerMaster);
}
/**
* The number of replicas to be created per primary.
*
*/
@Export(name="replicasPerPrimary", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> replicasPerPrimary;
/**
* @return The number of replicas to be created per primary.
*
*/
public Output> replicasPerPrimary() {
return Codegen.optional(this.replicasPerPrimary);
}
/**
* The number of shards to be created on a Premium Cluster Cache.
*
*/
@Export(name="shardCount", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> shardCount;
/**
* @return The number of shards to be created on a Premium Cluster Cache.
*
*/
public Output> shardCount() {
return Codegen.optional(this.shardCount);
}
/**
* The SKU of the Redis cache to deploy.
*
*/
@Export(name="sku", refs={SkuResponse.class}, tree="[0]")
private Output sku;
/**
* @return The SKU of the Redis cache to deploy.
*
*/
public Output sku() {
return this.sku;
}
/**
* Redis SSL port.
*
*/
@Export(name="sslPort", refs={Integer.class}, tree="[0]")
private Output sslPort;
/**
* @return Redis SSL port.
*
*/
public Output sslPort() {
return this.sslPort;
}
/**
* Static IP address. Optionally, may be specified when deploying a Redis cache inside an existing Azure Virtual Network; auto assigned by default.
*
*/
@Export(name="staticIP", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> staticIP;
/**
* @return Static IP address. Optionally, may be specified when deploying a Redis cache inside an existing Azure Virtual Network; auto assigned by default.
*
*/
public Output> staticIP() {
return Codegen.optional(this.staticIP);
}
/**
* The full resource ID of a subnet in a virtual network to deploy the Redis cache in. Example format: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/Microsoft.{Network|ClassicNetwork}/VirtualNetworks/vnet1/subnets/subnet1
*
*/
@Export(name="subnetId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> subnetId;
/**
* @return The full resource ID of a subnet in a virtual network to deploy the Redis cache in. Example format: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/Microsoft.{Network|ClassicNetwork}/VirtualNetworks/vnet1/subnets/subnet1
*
*/
public Output> subnetId() {
return Codegen.optional(this.subnetId);
}
/**
* Resource tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A dictionary of tenant settings
*
*/
@Export(name="tenantSettings", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tenantSettings;
/**
* @return A dictionary of tenant settings
*
*/
public Output>> tenantSettings() {
return Codegen.optional(this.tenantSettings);
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* A list of availability zones denoting where the resource needs to come from.
*
*/
@Export(name="zones", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> zones;
/**
* @return A list of availability zones denoting where the resource needs to come from.
*
*/
public Output>> zones() {
return Codegen.optional(this.zones);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Redis(java.lang.String name) {
this(name, RedisArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Redis(java.lang.String name, RedisArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Redis(java.lang.String name, RedisArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:cache:Redis", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Redis(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:cache:Redis", name, null, makeResourceOptions(options, id), false);
}
private static RedisArgs makeArgs(RedisArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? RedisArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:cache/v20150801:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20160401:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20170201:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20171001:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20180301:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20190701:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20200601:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20201201:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20210601:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20220501:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20220601:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20230401:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20230501preview:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20230801:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20240301:Redis").build()),
Output.of(Alias.builder().type("azure-native:cache/v20240401preview:Redis").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Redis get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Redis(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy