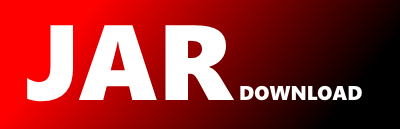
com.pulumi.azurenative.cdn.CdnFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cdn;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.cdn.inputs.GetAFDCustomDomainArgs;
import com.pulumi.azurenative.cdn.inputs.GetAFDCustomDomainPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetAFDEndpointArgs;
import com.pulumi.azurenative.cdn.inputs.GetAFDEndpointPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetAFDOriginArgs;
import com.pulumi.azurenative.cdn.inputs.GetAFDOriginGroupArgs;
import com.pulumi.azurenative.cdn.inputs.GetAFDOriginGroupPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetAFDOriginPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetAFDTargetGroupArgs;
import com.pulumi.azurenative.cdn.inputs.GetAFDTargetGroupPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetCustomDomainArgs;
import com.pulumi.azurenative.cdn.inputs.GetCustomDomainPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetEndpointArgs;
import com.pulumi.azurenative.cdn.inputs.GetEndpointPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetKeyGroupArgs;
import com.pulumi.azurenative.cdn.inputs.GetKeyGroupPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetOriginArgs;
import com.pulumi.azurenative.cdn.inputs.GetOriginGroupArgs;
import com.pulumi.azurenative.cdn.inputs.GetOriginGroupPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetOriginPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetPolicyArgs;
import com.pulumi.azurenative.cdn.inputs.GetPolicyPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetProfileArgs;
import com.pulumi.azurenative.cdn.inputs.GetProfilePlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetProfileSupportedOptimizationTypesArgs;
import com.pulumi.azurenative.cdn.inputs.GetProfileSupportedOptimizationTypesPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetRouteArgs;
import com.pulumi.azurenative.cdn.inputs.GetRoutePlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetRuleArgs;
import com.pulumi.azurenative.cdn.inputs.GetRulePlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetRuleSetArgs;
import com.pulumi.azurenative.cdn.inputs.GetRuleSetPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetSecretArgs;
import com.pulumi.azurenative.cdn.inputs.GetSecretPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetSecurityPolicyArgs;
import com.pulumi.azurenative.cdn.inputs.GetSecurityPolicyPlainArgs;
import com.pulumi.azurenative.cdn.inputs.GetTunnelPolicyArgs;
import com.pulumi.azurenative.cdn.inputs.GetTunnelPolicyPlainArgs;
import com.pulumi.azurenative.cdn.outputs.GetAFDCustomDomainResult;
import com.pulumi.azurenative.cdn.outputs.GetAFDEndpointResult;
import com.pulumi.azurenative.cdn.outputs.GetAFDOriginGroupResult;
import com.pulumi.azurenative.cdn.outputs.GetAFDOriginResult;
import com.pulumi.azurenative.cdn.outputs.GetAFDTargetGroupResult;
import com.pulumi.azurenative.cdn.outputs.GetCustomDomainResult;
import com.pulumi.azurenative.cdn.outputs.GetEndpointResult;
import com.pulumi.azurenative.cdn.outputs.GetKeyGroupResult;
import com.pulumi.azurenative.cdn.outputs.GetOriginGroupResult;
import com.pulumi.azurenative.cdn.outputs.GetOriginResult;
import com.pulumi.azurenative.cdn.outputs.GetPolicyResult;
import com.pulumi.azurenative.cdn.outputs.GetProfileResult;
import com.pulumi.azurenative.cdn.outputs.GetProfileSupportedOptimizationTypesResult;
import com.pulumi.azurenative.cdn.outputs.GetRouteResult;
import com.pulumi.azurenative.cdn.outputs.GetRuleResult;
import com.pulumi.azurenative.cdn.outputs.GetRuleSetResult;
import com.pulumi.azurenative.cdn.outputs.GetSecretResult;
import com.pulumi.azurenative.cdn.outputs.GetSecurityPolicyResult;
import com.pulumi.azurenative.cdn.outputs.GetTunnelPolicyResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class CdnFunctions {
/**
* Gets an existing AzureFrontDoor domain with the specified domain name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getAFDCustomDomain(GetAFDCustomDomainArgs args) {
return getAFDCustomDomain(args, InvokeOptions.Empty);
}
/**
* Gets an existing AzureFrontDoor domain with the specified domain name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getAFDCustomDomainPlain(GetAFDCustomDomainPlainArgs args) {
return getAFDCustomDomainPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing AzureFrontDoor domain with the specified domain name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getAFDCustomDomain(GetAFDCustomDomainArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getAFDCustomDomain", TypeShape.of(GetAFDCustomDomainResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing AzureFrontDoor domain with the specified domain name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getAFDCustomDomainPlain(GetAFDCustomDomainPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getAFDCustomDomain", TypeShape.of(GetAFDCustomDomainResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing AzureFrontDoor endpoint with the specified endpoint name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getAFDEndpoint(GetAFDEndpointArgs args) {
return getAFDEndpoint(args, InvokeOptions.Empty);
}
/**
* Gets an existing AzureFrontDoor endpoint with the specified endpoint name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getAFDEndpointPlain(GetAFDEndpointPlainArgs args) {
return getAFDEndpointPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing AzureFrontDoor endpoint with the specified endpoint name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getAFDEndpoint(GetAFDEndpointArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getAFDEndpoint", TypeShape.of(GetAFDEndpointResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing AzureFrontDoor endpoint with the specified endpoint name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getAFDEndpointPlain(GetAFDEndpointPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getAFDEndpoint", TypeShape.of(GetAFDEndpointResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing origin within an origin group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getAFDOrigin(GetAFDOriginArgs args) {
return getAFDOrigin(args, InvokeOptions.Empty);
}
/**
* Gets an existing origin within an origin group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getAFDOriginPlain(GetAFDOriginPlainArgs args) {
return getAFDOriginPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing origin within an origin group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getAFDOrigin(GetAFDOriginArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getAFDOrigin", TypeShape.of(GetAFDOriginResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing origin within an origin group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getAFDOriginPlain(GetAFDOriginPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getAFDOrigin", TypeShape.of(GetAFDOriginResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing origin group within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getAFDOriginGroup(GetAFDOriginGroupArgs args) {
return getAFDOriginGroup(args, InvokeOptions.Empty);
}
/**
* Gets an existing origin group within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getAFDOriginGroupPlain(GetAFDOriginGroupPlainArgs args) {
return getAFDOriginGroupPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing origin group within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getAFDOriginGroup(GetAFDOriginGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getAFDOriginGroup", TypeShape.of(GetAFDOriginGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing origin group within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getAFDOriginGroupPlain(GetAFDOriginGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getAFDOriginGroup", TypeShape.of(GetAFDOriginGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing target group within a profile.
* Azure REST API version: 2024-06-01-preview.
*
*/
public static Output getAFDTargetGroup(GetAFDTargetGroupArgs args) {
return getAFDTargetGroup(args, InvokeOptions.Empty);
}
/**
* Gets an existing target group within a profile.
* Azure REST API version: 2024-06-01-preview.
*
*/
public static CompletableFuture getAFDTargetGroupPlain(GetAFDTargetGroupPlainArgs args) {
return getAFDTargetGroupPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing target group within a profile.
* Azure REST API version: 2024-06-01-preview.
*
*/
public static Output getAFDTargetGroup(GetAFDTargetGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getAFDTargetGroup", TypeShape.of(GetAFDTargetGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing target group within a profile.
* Azure REST API version: 2024-06-01-preview.
*
*/
public static CompletableFuture getAFDTargetGroupPlain(GetAFDTargetGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getAFDTargetGroup", TypeShape.of(GetAFDTargetGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing custom domain within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-10-02, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getCustomDomain(GetCustomDomainArgs args) {
return getCustomDomain(args, InvokeOptions.Empty);
}
/**
* Gets an existing custom domain within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-10-02, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getCustomDomainPlain(GetCustomDomainPlainArgs args) {
return getCustomDomainPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing custom domain within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-10-02, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getCustomDomain(GetCustomDomainArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getCustomDomain", TypeShape.of(GetCustomDomainResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing custom domain within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-10-02, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getCustomDomainPlain(GetCustomDomainPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getCustomDomain", TypeShape.of(GetCustomDomainResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing CDN endpoint with the specified endpoint name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-04-02, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getEndpoint(GetEndpointArgs args) {
return getEndpoint(args, InvokeOptions.Empty);
}
/**
* Gets an existing CDN endpoint with the specified endpoint name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-04-02, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getEndpointPlain(GetEndpointPlainArgs args) {
return getEndpointPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing CDN endpoint with the specified endpoint name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-04-02, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getEndpoint(GetEndpointArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getEndpoint", TypeShape.of(GetEndpointResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing CDN endpoint with the specified endpoint name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-04-02, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getEndpointPlain(GetEndpointPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getEndpoint", TypeShape.of(GetEndpointResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing KeyGroup within a profile.
* Azure REST API version: 2023-07-01-preview.
*
* Other available API versions: 2024-05-01-preview, 2024-06-01-preview.
*
*/
public static Output getKeyGroup(GetKeyGroupArgs args) {
return getKeyGroup(args, InvokeOptions.Empty);
}
/**
* Gets an existing KeyGroup within a profile.
* Azure REST API version: 2023-07-01-preview.
*
* Other available API versions: 2024-05-01-preview, 2024-06-01-preview.
*
*/
public static CompletableFuture getKeyGroupPlain(GetKeyGroupPlainArgs args) {
return getKeyGroupPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing KeyGroup within a profile.
* Azure REST API version: 2023-07-01-preview.
*
* Other available API versions: 2024-05-01-preview, 2024-06-01-preview.
*
*/
public static Output getKeyGroup(GetKeyGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getKeyGroup", TypeShape.of(GetKeyGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing KeyGroup within a profile.
* Azure REST API version: 2023-07-01-preview.
*
* Other available API versions: 2024-05-01-preview, 2024-06-01-preview.
*
*/
public static CompletableFuture getKeyGroupPlain(GetKeyGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getKeyGroup", TypeShape.of(GetKeyGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing origin within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getOrigin(GetOriginArgs args) {
return getOrigin(args, InvokeOptions.Empty);
}
/**
* Gets an existing origin within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getOriginPlain(GetOriginPlainArgs args) {
return getOriginPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing origin within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getOrigin(GetOriginArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getOrigin", TypeShape.of(GetOriginResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing origin within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getOriginPlain(GetOriginPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getOrigin", TypeShape.of(GetOriginResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing origin group within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getOriginGroup(GetOriginGroupArgs args) {
return getOriginGroup(args, InvokeOptions.Empty);
}
/**
* Gets an existing origin group within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getOriginGroupPlain(GetOriginGroupPlainArgs args) {
return getOriginGroupPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing origin group within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getOriginGroup(GetOriginGroupArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getOriginGroup", TypeShape.of(GetOriginGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing origin group within an endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getOriginGroupPlain(GetOriginGroupPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getOriginGroup", TypeShape.of(GetOriginGroupResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieve protection policy with specified name within a resource group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getPolicy(GetPolicyArgs args) {
return getPolicy(args, InvokeOptions.Empty);
}
/**
* Retrieve protection policy with specified name within a resource group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getPolicyPlain(GetPolicyPlainArgs args) {
return getPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Retrieve protection policy with specified name within a resource group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getPolicy(GetPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getPolicy", TypeShape.of(GetPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Retrieve protection policy with specified name within a resource group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getPolicyPlain(GetPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getPolicy", TypeShape.of(GetPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an Azure Front Door Standard or Azure Front Door Premium or CDN profile with the specified profile name under the specified subscription and resource group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-04-02, 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getProfile(GetProfileArgs args) {
return getProfile(args, InvokeOptions.Empty);
}
/**
* Gets an Azure Front Door Standard or Azure Front Door Premium or CDN profile with the specified profile name under the specified subscription and resource group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-04-02, 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getProfilePlain(GetProfilePlainArgs args) {
return getProfilePlain(args, InvokeOptions.Empty);
}
/**
* Gets an Azure Front Door Standard or Azure Front Door Premium or CDN profile with the specified profile name under the specified subscription and resource group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-04-02, 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getProfile(GetProfileArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getProfile", TypeShape.of(GetProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an Azure Front Door Standard or Azure Front Door Premium or CDN profile with the specified profile name under the specified subscription and resource group.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2016-04-02, 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getProfilePlain(GetProfilePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getProfile", TypeShape.of(GetProfileResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the supported optimization types for the current profile. A user can create an endpoint with an optimization type from the listed values.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getProfileSupportedOptimizationTypes(GetProfileSupportedOptimizationTypesArgs args) {
return getProfileSupportedOptimizationTypes(args, InvokeOptions.Empty);
}
/**
* Gets the supported optimization types for the current profile. A user can create an endpoint with an optimization type from the listed values.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getProfileSupportedOptimizationTypesPlain(GetProfileSupportedOptimizationTypesPlainArgs args) {
return getProfileSupportedOptimizationTypesPlain(args, InvokeOptions.Empty);
}
/**
* Gets the supported optimization types for the current profile. A user can create an endpoint with an optimization type from the listed values.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getProfileSupportedOptimizationTypes(GetProfileSupportedOptimizationTypesArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getProfileSupportedOptimizationTypes", TypeShape.of(GetProfileSupportedOptimizationTypesResult.class), args, Utilities.withVersion(options));
}
/**
* Gets the supported optimization types for the current profile. A user can create an endpoint with an optimization type from the listed values.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getProfileSupportedOptimizationTypesPlain(GetProfileSupportedOptimizationTypesPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getProfileSupportedOptimizationTypes", TypeShape.of(GetProfileSupportedOptimizationTypesResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing route with the specified route name under the specified subscription, resource group, profile, and AzureFrontDoor endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getRoute(GetRouteArgs args) {
return getRoute(args, InvokeOptions.Empty);
}
/**
* Gets an existing route with the specified route name under the specified subscription, resource group, profile, and AzureFrontDoor endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getRoutePlain(GetRoutePlainArgs args) {
return getRoutePlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing route with the specified route name under the specified subscription, resource group, profile, and AzureFrontDoor endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getRoute(GetRouteArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getRoute", TypeShape.of(GetRouteResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing route with the specified route name under the specified subscription, resource group, profile, and AzureFrontDoor endpoint.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getRoutePlain(GetRoutePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getRoute", TypeShape.of(GetRouteResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing delivery rule within a rule set.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getRule(GetRuleArgs args) {
return getRule(args, InvokeOptions.Empty);
}
/**
* Gets an existing delivery rule within a rule set.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getRulePlain(GetRulePlainArgs args) {
return getRulePlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing delivery rule within a rule set.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getRule(GetRuleArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getRule", TypeShape.of(GetRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing delivery rule within a rule set.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getRulePlain(GetRulePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getRule", TypeShape.of(GetRuleResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing AzureFrontDoor rule set with the specified rule set name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getRuleSet(GetRuleSetArgs args) {
return getRuleSet(args, InvokeOptions.Empty);
}
/**
* Gets an existing AzureFrontDoor rule set with the specified rule set name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getRuleSetPlain(GetRuleSetPlainArgs args) {
return getRuleSetPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing AzureFrontDoor rule set with the specified rule set name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getRuleSet(GetRuleSetArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getRuleSet", TypeShape.of(GetRuleSetResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing AzureFrontDoor rule set with the specified rule set name under the specified subscription, resource group and profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getRuleSetPlain(GetRuleSetPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getRuleSet", TypeShape.of(GetRuleSetResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing Secret within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getSecret(GetSecretArgs args) {
return getSecret(args, InvokeOptions.Empty);
}
/**
* Gets an existing Secret within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getSecretPlain(GetSecretPlainArgs args) {
return getSecretPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing Secret within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getSecret(GetSecretArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getSecret", TypeShape.of(GetSecretResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing Secret within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getSecretPlain(GetSecretPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getSecret", TypeShape.of(GetSecretResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing security policy within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getSecurityPolicy(GetSecurityPolicyArgs args) {
return getSecurityPolicy(args, InvokeOptions.Empty);
}
/**
* Gets an existing security policy within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getSecurityPolicyPlain(GetSecurityPolicyPlainArgs args) {
return getSecurityPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing security policy within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static Output getSecurityPolicy(GetSecurityPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getSecurityPolicy", TypeShape.of(GetSecurityPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing security policy within a profile.
* Azure REST API version: 2023-05-01.
*
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview, 2024-09-01.
*
*/
public static CompletableFuture getSecurityPolicyPlain(GetSecurityPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getSecurityPolicy", TypeShape.of(GetSecurityPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing tunnel policy within a profile.
* Azure REST API version: 2024-06-01-preview.
*
*/
public static Output getTunnelPolicy(GetTunnelPolicyArgs args) {
return getTunnelPolicy(args, InvokeOptions.Empty);
}
/**
* Gets an existing tunnel policy within a profile.
* Azure REST API version: 2024-06-01-preview.
*
*/
public static CompletableFuture getTunnelPolicyPlain(GetTunnelPolicyPlainArgs args) {
return getTunnelPolicyPlain(args, InvokeOptions.Empty);
}
/**
* Gets an existing tunnel policy within a profile.
* Azure REST API version: 2024-06-01-preview.
*
*/
public static Output getTunnelPolicy(GetTunnelPolicyArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:cdn:getTunnelPolicy", TypeShape.of(GetTunnelPolicyResult.class), args, Utilities.withVersion(options));
}
/**
* Gets an existing tunnel policy within a profile.
* Azure REST API version: 2024-06-01-preview.
*
*/
public static CompletableFuture getTunnelPolicyPlain(GetTunnelPolicyPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:cdn:getTunnelPolicy", TypeShape.of(GetTunnelPolicyResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy