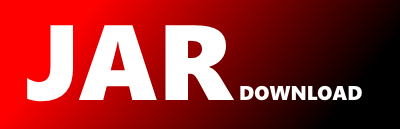
com.pulumi.azurenative.cdn.OriginGroupArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.cdn;
import com.pulumi.azurenative.cdn.inputs.HealthProbeParametersArgs;
import com.pulumi.azurenative.cdn.inputs.ResourceReferenceArgs;
import com.pulumi.azurenative.cdn.inputs.ResponseBasedOriginErrorDetectionParametersArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class OriginGroupArgs extends com.pulumi.resources.ResourceArgs {
public static final OriginGroupArgs Empty = new OriginGroupArgs();
/**
* Name of the endpoint under the profile which is unique globally.
*
*/
@Import(name="endpointName", required=true)
private Output endpointName;
/**
* @return Name of the endpoint under the profile which is unique globally.
*
*/
public Output endpointName() {
return this.endpointName;
}
/**
* Health probe settings to the origin that is used to determine the health of the origin.
*
*/
@Import(name="healthProbeSettings")
private @Nullable Output healthProbeSettings;
/**
* @return Health probe settings to the origin that is used to determine the health of the origin.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy