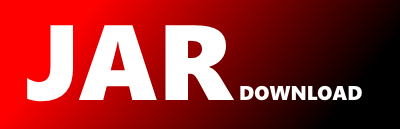
com.pulumi.azurenative.compute.DedicatedHostGroup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.compute.DedicatedHostGroupArgs;
import com.pulumi.azurenative.compute.outputs.DedicatedHostGroupInstanceViewResponse;
import com.pulumi.azurenative.compute.outputs.DedicatedHostGroupPropertiesResponseAdditionalCapabilities;
import com.pulumi.azurenative.compute.outputs.SubResourceReadOnlyResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Specifies information about the dedicated host group that the dedicated hosts should be assigned to. Currently, a dedicated host can only be added to a dedicated host group at creation time. An existing dedicated host cannot be added to another dedicated host group.
* Azure REST API version: 2023-03-01. Prior API version in Azure Native 1.x: 2020-12-01.
*
* Other available API versions: 2023-07-01, 2023-09-01, 2024-03-01, 2024-07-01.
*
* ## Example Usage
* ### Create or update a dedicated host group with Ultra SSD support.
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.DedicatedHostGroup;
* import com.pulumi.azurenative.compute.DedicatedHostGroupArgs;
* import com.pulumi.azurenative.compute.inputs.DedicatedHostGroupPropertiesAdditionalCapabilitiesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var dedicatedHostGroup = new DedicatedHostGroup("dedicatedHostGroup", DedicatedHostGroupArgs.builder()
* .additionalCapabilities(DedicatedHostGroupPropertiesAdditionalCapabilitiesArgs.builder()
* .ultraSSDEnabled(true)
* .build())
* .hostGroupName("myDedicatedHostGroup")
* .location("westus")
* .platformFaultDomainCount(3)
* .resourceGroupName("myResourceGroup")
* .supportAutomaticPlacement(true)
* .tags(Map.of("department", "finance"))
* .zones("1")
* .build());
*
* }
* }
*
* }
*
* ### Create or update a dedicated host group.
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.DedicatedHostGroup;
* import com.pulumi.azurenative.compute.DedicatedHostGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var dedicatedHostGroup = new DedicatedHostGroup("dedicatedHostGroup", DedicatedHostGroupArgs.builder()
* .hostGroupName("myDedicatedHostGroup")
* .location("westus")
* .platformFaultDomainCount(3)
* .resourceGroupName("myResourceGroup")
* .supportAutomaticPlacement(true)
* .tags(Map.of("department", "finance"))
* .zones("1")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:compute:DedicatedHostGroup myDedicatedHostGroup /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/hostGroups/{hostGroupName}
* ```
*
*/
@ResourceType(type="azure-native:compute:DedicatedHostGroup")
public class DedicatedHostGroup extends com.pulumi.resources.CustomResource {
/**
* Enables or disables a capability on the dedicated host group. Minimum api-version: 2022-03-01.
*
*/
@Export(name="additionalCapabilities", refs={DedicatedHostGroupPropertiesResponseAdditionalCapabilities.class}, tree="[0]")
private Output* @Nullable */ DedicatedHostGroupPropertiesResponseAdditionalCapabilities> additionalCapabilities;
/**
* @return Enables or disables a capability on the dedicated host group. Minimum api-version: 2022-03-01.
*
*/
public Output> additionalCapabilities() {
return Codegen.optional(this.additionalCapabilities);
}
/**
* A list of references to all dedicated hosts in the dedicated host group.
*
*/
@Export(name="hosts", refs={List.class,SubResourceReadOnlyResponse.class}, tree="[0,1]")
private Output> hosts;
/**
* @return A list of references to all dedicated hosts in the dedicated host group.
*
*/
public Output> hosts() {
return this.hosts;
}
/**
* The dedicated host group instance view, which has the list of instance view of the dedicated hosts under the dedicated host group.
*
*/
@Export(name="instanceView", refs={DedicatedHostGroupInstanceViewResponse.class}, tree="[0]")
private Output instanceView;
/**
* @return The dedicated host group instance view, which has the list of instance view of the dedicated hosts under the dedicated host group.
*
*/
public Output instanceView() {
return this.instanceView;
}
/**
* Resource location
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Resource location
*
*/
public Output location() {
return this.location;
}
/**
* Resource name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name
*
*/
public Output name() {
return this.name;
}
/**
* Number of fault domains that the host group can span.
*
*/
@Export(name="platformFaultDomainCount", refs={Integer.class}, tree="[0]")
private Output platformFaultDomainCount;
/**
* @return Number of fault domains that the host group can span.
*
*/
public Output platformFaultDomainCount() {
return this.platformFaultDomainCount;
}
/**
* Specifies whether virtual machines or virtual machine scale sets can be placed automatically on the dedicated host group. Automatic placement means resources are allocated on dedicated hosts, that are chosen by Azure, under the dedicated host group. The value is defaulted to 'false' when not provided. Minimum api-version: 2020-06-01.
*
*/
@Export(name="supportAutomaticPlacement", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> supportAutomaticPlacement;
/**
* @return Specifies whether virtual machines or virtual machine scale sets can be placed automatically on the dedicated host group. Automatic placement means resources are allocated on dedicated hosts, that are chosen by Azure, under the dedicated host group. The value is defaulted to 'false' when not provided. Minimum api-version: 2020-06-01.
*
*/
public Output> supportAutomaticPlacement() {
return Codegen.optional(this.supportAutomaticPlacement);
}
/**
* Resource tags
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Resource type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type
*
*/
public Output type() {
return this.type;
}
/**
* Availability Zone to use for this host group. Only single zone is supported. The zone can be assigned only during creation. If not provided, the group supports all zones in the region. If provided, enforces each host in the group to be in the same zone.
*
*/
@Export(name="zones", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> zones;
/**
* @return Availability Zone to use for this host group. Only single zone is supported. The zone can be assigned only during creation. If not provided, the group supports all zones in the region. If provided, enforces each host in the group to be in the same zone.
*
*/
public Output>> zones() {
return Codegen.optional(this.zones);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public DedicatedHostGroup(java.lang.String name) {
this(name, DedicatedHostGroupArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public DedicatedHostGroup(java.lang.String name, DedicatedHostGroupArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public DedicatedHostGroup(java.lang.String name, DedicatedHostGroupArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:compute:DedicatedHostGroup", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private DedicatedHostGroup(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:compute:DedicatedHostGroup", name, null, makeResourceOptions(options, id), false);
}
private static DedicatedHostGroupArgs makeArgs(DedicatedHostGroupArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? DedicatedHostGroupArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:compute/v20190301:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20190701:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20191201:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20200601:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20201201:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20210301:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20210401:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20210701:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20211101:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20220301:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20220801:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20221101:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230301:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230701:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230901:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20240301:DedicatedHostGroup").build()),
Output.of(Alias.builder().type("azure-native:compute/v20240701:DedicatedHostGroup").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static DedicatedHostGroup get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new DedicatedHostGroup(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy