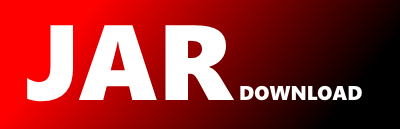
com.pulumi.azurenative.compute.DedicatedHostGroupArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.compute.inputs.DedicatedHostGroupPropertiesAdditionalCapabilitiesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DedicatedHostGroupArgs extends com.pulumi.resources.ResourceArgs {
public static final DedicatedHostGroupArgs Empty = new DedicatedHostGroupArgs();
/**
* Enables or disables a capability on the dedicated host group. Minimum api-version: 2022-03-01.
*
*/
@Import(name="additionalCapabilities")
private @Nullable Output additionalCapabilities;
/**
* @return Enables or disables a capability on the dedicated host group. Minimum api-version: 2022-03-01.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy