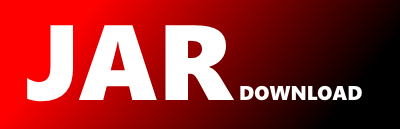
com.pulumi.azurenative.compute.DiskEncryptionSetArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.compute.enums.DiskEncryptionSetType;
import com.pulumi.azurenative.compute.inputs.EncryptionSetIdentityArgs;
import com.pulumi.azurenative.compute.inputs.KeyForDiskEncryptionSetArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DiskEncryptionSetArgs extends com.pulumi.resources.ResourceArgs {
public static final DiskEncryptionSetArgs Empty = new DiskEncryptionSetArgs();
/**
* The key vault key which is currently used by this disk encryption set.
*
*/
@Import(name="activeKey")
private @Nullable Output activeKey;
/**
* @return The key vault key which is currently used by this disk encryption set.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy