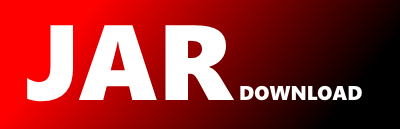
com.pulumi.azurenative.compute.GalleryApplicationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.compute.enums.OperatingSystemTypes;
import com.pulumi.azurenative.compute.inputs.GalleryApplicationCustomActionArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GalleryApplicationArgs extends com.pulumi.resources.ResourceArgs {
public static final GalleryApplicationArgs Empty = new GalleryApplicationArgs();
/**
* A list of custom actions that can be performed with all of the Gallery Application Versions within this Gallery Application.
*
*/
@Import(name="customActions")
private @Nullable Output> customActions;
/**
* @return A list of custom actions that can be performed with all of the Gallery Application Versions within this Gallery Application.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy