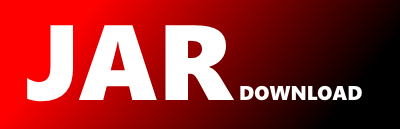
com.pulumi.azurenative.compute.GalleryImageArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.compute.enums.Architecture;
import com.pulumi.azurenative.compute.enums.HyperVGeneration;
import com.pulumi.azurenative.compute.enums.OperatingSystemStateTypes;
import com.pulumi.azurenative.compute.enums.OperatingSystemTypes;
import com.pulumi.azurenative.compute.inputs.DisallowedArgs;
import com.pulumi.azurenative.compute.inputs.GalleryImageFeatureArgs;
import com.pulumi.azurenative.compute.inputs.GalleryImageIdentifierArgs;
import com.pulumi.azurenative.compute.inputs.ImagePurchasePlanArgs;
import com.pulumi.azurenative.compute.inputs.RecommendedMachineConfigurationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GalleryImageArgs extends com.pulumi.resources.ResourceArgs {
public static final GalleryImageArgs Empty = new GalleryImageArgs();
/**
* The architecture of the image. Applicable to OS disks only.
*
*/
@Import(name="architecture")
private @Nullable Output> architecture;
/**
* @return The architecture of the image. Applicable to OS disks only.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy