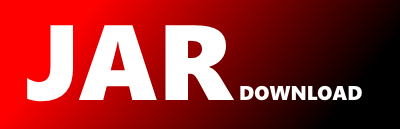
com.pulumi.azurenative.compute.ImageArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.compute.enums.HyperVGenerationTypes;
import com.pulumi.azurenative.compute.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.compute.inputs.ImageStorageProfileArgs;
import com.pulumi.azurenative.compute.inputs.SubResourceArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ImageArgs extends com.pulumi.resources.ResourceArgs {
public static final ImageArgs Empty = new ImageArgs();
/**
* The extended location of the Image.
*
*/
@Import(name="extendedLocation")
private @Nullable Output extendedLocation;
/**
* @return The extended location of the Image.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy