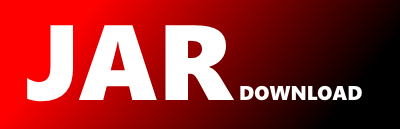
com.pulumi.azurenative.compute.Snapshot Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.compute.SnapshotArgs;
import com.pulumi.azurenative.compute.outputs.CopyCompletionErrorResponse;
import com.pulumi.azurenative.compute.outputs.CreationDataResponse;
import com.pulumi.azurenative.compute.outputs.DiskSecurityProfileResponse;
import com.pulumi.azurenative.compute.outputs.EncryptionResponse;
import com.pulumi.azurenative.compute.outputs.EncryptionSettingsCollectionResponse;
import com.pulumi.azurenative.compute.outputs.ExtendedLocationResponse;
import com.pulumi.azurenative.compute.outputs.PurchasePlanResponse;
import com.pulumi.azurenative.compute.outputs.SnapshotSkuResponse;
import com.pulumi.azurenative.compute.outputs.SupportedCapabilitiesResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Snapshot resource.
* Azure REST API version: 2022-07-02. Prior API version in Azure Native 1.x: 2020-12-01.
*
* Other available API versions: 2016-04-30-preview, 2017-03-30, 2018-06-01, 2023-01-02, 2023-04-02, 2023-10-02, 2024-03-02.
*
* ## Example Usage
* ### Create a snapshot by importing an unmanaged blob from a different subscription.
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.Snapshot;
* import com.pulumi.azurenative.compute.SnapshotArgs;
* import com.pulumi.azurenative.compute.inputs.CreationDataArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var snapshot = new Snapshot("snapshot", SnapshotArgs.builder()
* .creationData(CreationDataArgs.builder()
* .createOption("Import")
* .sourceUri("https://mystorageaccount.blob.core.windows.net/osimages/osimage.vhd")
* .storageAccountId("subscriptions/{subscription-id}/resourceGroups/myResourceGroup/providers/Microsoft.Storage/storageAccounts/myStorageAccount")
* .build())
* .location("West US")
* .resourceGroupName("myResourceGroup")
* .snapshotName("mySnapshot1")
* .build());
*
* }
* }
*
* }
*
* ### Create a snapshot by importing an unmanaged blob from the same subscription.
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.Snapshot;
* import com.pulumi.azurenative.compute.SnapshotArgs;
* import com.pulumi.azurenative.compute.inputs.CreationDataArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var snapshot = new Snapshot("snapshot", SnapshotArgs.builder()
* .creationData(CreationDataArgs.builder()
* .createOption("Import")
* .sourceUri("https://mystorageaccount.blob.core.windows.net/osimages/osimage.vhd")
* .build())
* .location("West US")
* .resourceGroupName("myResourceGroup")
* .snapshotName("mySnapshot1")
* .build());
*
* }
* }
*
* }
*
* ### Create a snapshot from an existing snapshot in the same or a different subscription in a different region.
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.Snapshot;
* import com.pulumi.azurenative.compute.SnapshotArgs;
* import com.pulumi.azurenative.compute.inputs.CreationDataArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var snapshot = new Snapshot("snapshot", SnapshotArgs.builder()
* .creationData(CreationDataArgs.builder()
* .createOption("CopyStart")
* .sourceResourceId("subscriptions/{subscription-id}/resourceGroups/myResourceGroup/providers/Microsoft.Compute/snapshots/mySnapshot1")
* .build())
* .location("West US")
* .resourceGroupName("myResourceGroup")
* .snapshotName("mySnapshot2")
* .build());
*
* }
* }
*
* }
*
* ### Create a snapshot from an existing snapshot in the same or a different subscription.
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.Snapshot;
* import com.pulumi.azurenative.compute.SnapshotArgs;
* import com.pulumi.azurenative.compute.inputs.CreationDataArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var snapshot = new Snapshot("snapshot", SnapshotArgs.builder()
* .creationData(CreationDataArgs.builder()
* .createOption("Copy")
* .sourceResourceId("subscriptions/{subscription-id}/resourceGroups/myResourceGroup/providers/Microsoft.Compute/snapshots/mySnapshot1")
* .build())
* .location("West US")
* .resourceGroupName("myResourceGroup")
* .snapshotName("mySnapshot2")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:compute:Snapshot mySnapshot2 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/snapshots/{snapshotName}
* ```
*
*/
@ResourceType(type="azure-native:compute:Snapshot")
public class Snapshot extends com.pulumi.resources.CustomResource {
/**
* Percentage complete for the background copy when a resource is created via the CopyStart operation.
*
*/
@Export(name="completionPercent", refs={Double.class}, tree="[0]")
private Output* @Nullable */ Double> completionPercent;
/**
* @return Percentage complete for the background copy when a resource is created via the CopyStart operation.
*
*/
public Output> completionPercent() {
return Codegen.optional(this.completionPercent);
}
/**
* Indicates the error details if the background copy of a resource created via the CopyStart operation fails.
*
*/
@Export(name="copyCompletionError", refs={CopyCompletionErrorResponse.class}, tree="[0]")
private Output* @Nullable */ CopyCompletionErrorResponse> copyCompletionError;
/**
* @return Indicates the error details if the background copy of a resource created via the CopyStart operation fails.
*
*/
public Output> copyCompletionError() {
return Codegen.optional(this.copyCompletionError);
}
/**
* Disk source information. CreationData information cannot be changed after the disk has been created.
*
*/
@Export(name="creationData", refs={CreationDataResponse.class}, tree="[0]")
private Output creationData;
/**
* @return Disk source information. CreationData information cannot be changed after the disk has been created.
*
*/
public Output creationData() {
return this.creationData;
}
/**
* Additional authentication requirements when exporting or uploading to a disk or snapshot.
*
*/
@Export(name="dataAccessAuthMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> dataAccessAuthMode;
/**
* @return Additional authentication requirements when exporting or uploading to a disk or snapshot.
*
*/
public Output> dataAccessAuthMode() {
return Codegen.optional(this.dataAccessAuthMode);
}
/**
* ARM id of the DiskAccess resource for using private endpoints on disks.
*
*/
@Export(name="diskAccessId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> diskAccessId;
/**
* @return ARM id of the DiskAccess resource for using private endpoints on disks.
*
*/
public Output> diskAccessId() {
return Codegen.optional(this.diskAccessId);
}
/**
* The size of the disk in bytes. This field is read only.
*
*/
@Export(name="diskSizeBytes", refs={Double.class}, tree="[0]")
private Output diskSizeBytes;
/**
* @return The size of the disk in bytes. This field is read only.
*
*/
public Output diskSizeBytes() {
return this.diskSizeBytes;
}
/**
* If creationData.createOption is Empty, this field is mandatory and it indicates the size of the disk to create. If this field is present for updates or creation with other options, it indicates a resize. Resizes are only allowed if the disk is not attached to a running VM, and can only increase the disk's size.
*
*/
@Export(name="diskSizeGB", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> diskSizeGB;
/**
* @return If creationData.createOption is Empty, this field is mandatory and it indicates the size of the disk to create. If this field is present for updates or creation with other options, it indicates a resize. Resizes are only allowed if the disk is not attached to a running VM, and can only increase the disk's size.
*
*/
public Output> diskSizeGB() {
return Codegen.optional(this.diskSizeGB);
}
/**
* The state of the snapshot.
*
*/
@Export(name="diskState", refs={String.class}, tree="[0]")
private Output diskState;
/**
* @return The state of the snapshot.
*
*/
public Output diskState() {
return this.diskState;
}
/**
* Encryption property can be used to encrypt data at rest with customer managed keys or platform managed keys.
*
*/
@Export(name="encryption", refs={EncryptionResponse.class}, tree="[0]")
private Output* @Nullable */ EncryptionResponse> encryption;
/**
* @return Encryption property can be used to encrypt data at rest with customer managed keys or platform managed keys.
*
*/
public Output> encryption() {
return Codegen.optional(this.encryption);
}
/**
* Encryption settings collection used be Azure Disk Encryption, can contain multiple encryption settings per disk or snapshot.
*
*/
@Export(name="encryptionSettingsCollection", refs={EncryptionSettingsCollectionResponse.class}, tree="[0]")
private Output* @Nullable */ EncryptionSettingsCollectionResponse> encryptionSettingsCollection;
/**
* @return Encryption settings collection used be Azure Disk Encryption, can contain multiple encryption settings per disk or snapshot.
*
*/
public Output> encryptionSettingsCollection() {
return Codegen.optional(this.encryptionSettingsCollection);
}
/**
* The extended location where the snapshot will be created. Extended location cannot be changed.
*
*/
@Export(name="extendedLocation", refs={ExtendedLocationResponse.class}, tree="[0]")
private Output* @Nullable */ ExtendedLocationResponse> extendedLocation;
/**
* @return The extended location where the snapshot will be created. Extended location cannot be changed.
*
*/
public Output> extendedLocation() {
return Codegen.optional(this.extendedLocation);
}
/**
* The hypervisor generation of the Virtual Machine. Applicable to OS disks only.
*
*/
@Export(name="hyperVGeneration", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> hyperVGeneration;
/**
* @return The hypervisor generation of the Virtual Machine. Applicable to OS disks only.
*
*/
public Output> hyperVGeneration() {
return Codegen.optional(this.hyperVGeneration);
}
/**
* Whether a snapshot is incremental. Incremental snapshots on the same disk occupy less space than full snapshots and can be diffed.
*
*/
@Export(name="incremental", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> incremental;
/**
* @return Whether a snapshot is incremental. Incremental snapshots on the same disk occupy less space than full snapshots and can be diffed.
*
*/
public Output> incremental() {
return Codegen.optional(this.incremental);
}
/**
* Incremental snapshots for a disk share an incremental snapshot family id. The Get Page Range Diff API can only be called on incremental snapshots with the same family id.
*
*/
@Export(name="incrementalSnapshotFamilyId", refs={String.class}, tree="[0]")
private Output incrementalSnapshotFamilyId;
/**
* @return Incremental snapshots for a disk share an incremental snapshot family id. The Get Page Range Diff API can only be called on incremental snapshots with the same family id.
*
*/
public Output incrementalSnapshotFamilyId() {
return this.incrementalSnapshotFamilyId;
}
/**
* Resource location
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Resource location
*
*/
public Output location() {
return this.location;
}
/**
* Unused. Always Null.
*
*/
@Export(name="managedBy", refs={String.class}, tree="[0]")
private Output managedBy;
/**
* @return Unused. Always Null.
*
*/
public Output managedBy() {
return this.managedBy;
}
/**
* Resource name
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Resource name
*
*/
public Output name() {
return this.name;
}
/**
* Policy for accessing the disk via network.
*
*/
@Export(name="networkAccessPolicy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> networkAccessPolicy;
/**
* @return Policy for accessing the disk via network.
*
*/
public Output> networkAccessPolicy() {
return Codegen.optional(this.networkAccessPolicy);
}
/**
* The Operating System type.
*
*/
@Export(name="osType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> osType;
/**
* @return The Operating System type.
*
*/
public Output> osType() {
return Codegen.optional(this.osType);
}
/**
* The disk provisioning state.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The disk provisioning state.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* Policy for controlling export on the disk.
*
*/
@Export(name="publicNetworkAccess", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> publicNetworkAccess;
/**
* @return Policy for controlling export on the disk.
*
*/
public Output> publicNetworkAccess() {
return Codegen.optional(this.publicNetworkAccess);
}
/**
* Purchase plan information for the image from which the source disk for the snapshot was originally created.
*
*/
@Export(name="purchasePlan", refs={PurchasePlanResponse.class}, tree="[0]")
private Output* @Nullable */ PurchasePlanResponse> purchasePlan;
/**
* @return Purchase plan information for the image from which the source disk for the snapshot was originally created.
*
*/
public Output> purchasePlan() {
return Codegen.optional(this.purchasePlan);
}
/**
* Contains the security related information for the resource.
*
*/
@Export(name="securityProfile", refs={DiskSecurityProfileResponse.class}, tree="[0]")
private Output* @Nullable */ DiskSecurityProfileResponse> securityProfile;
/**
* @return Contains the security related information for the resource.
*
*/
public Output> securityProfile() {
return Codegen.optional(this.securityProfile);
}
/**
* The snapshots sku name. Can be Standard_LRS, Premium_LRS, or Standard_ZRS. This is an optional parameter for incremental snapshot and the default behavior is the SKU will be set to the same sku as the previous snapshot
*
*/
@Export(name="sku", refs={SnapshotSkuResponse.class}, tree="[0]")
private Output* @Nullable */ SnapshotSkuResponse> sku;
/**
* @return The snapshots sku name. Can be Standard_LRS, Premium_LRS, or Standard_ZRS. This is an optional parameter for incremental snapshot and the default behavior is the SKU will be set to the same sku as the previous snapshot
*
*/
public Output> sku() {
return Codegen.optional(this.sku);
}
/**
* List of supported capabilities for the image from which the source disk from the snapshot was originally created.
*
*/
@Export(name="supportedCapabilities", refs={SupportedCapabilitiesResponse.class}, tree="[0]")
private Output* @Nullable */ SupportedCapabilitiesResponse> supportedCapabilities;
/**
* @return List of supported capabilities for the image from which the source disk from the snapshot was originally created.
*
*/
public Output> supportedCapabilities() {
return Codegen.optional(this.supportedCapabilities);
}
/**
* Indicates the OS on a snapshot supports hibernation.
*
*/
@Export(name="supportsHibernation", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> supportsHibernation;
/**
* @return Indicates the OS on a snapshot supports hibernation.
*
*/
public Output> supportsHibernation() {
return Codegen.optional(this.supportsHibernation);
}
/**
* Resource tags
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Resource tags
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The time when the snapshot was created.
*
*/
@Export(name="timeCreated", refs={String.class}, tree="[0]")
private Output timeCreated;
/**
* @return The time when the snapshot was created.
*
*/
public Output timeCreated() {
return this.timeCreated;
}
/**
* Resource type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type
*
*/
public Output type() {
return this.type;
}
/**
* Unique Guid identifying the resource.
*
*/
@Export(name="uniqueId", refs={String.class}, tree="[0]")
private Output uniqueId;
/**
* @return Unique Guid identifying the resource.
*
*/
public Output uniqueId() {
return this.uniqueId;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Snapshot(java.lang.String name) {
this(name, SnapshotArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Snapshot(java.lang.String name, SnapshotArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Snapshot(java.lang.String name, SnapshotArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:compute:Snapshot", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Snapshot(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:compute:Snapshot", name, null, makeResourceOptions(options, id), false);
}
private static SnapshotArgs makeArgs(SnapshotArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? SnapshotArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:compute/v20160430preview:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20170330:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20180401:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20180601:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20180930:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20190301:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20190701:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20191101:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20200501:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20200630:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20200930:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20201201:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20210401:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20210801:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20211201:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20220302:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20220702:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230102:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230402:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20231002:Snapshot").build()),
Output.of(Alias.builder().type("azure-native:compute/v20240302:Snapshot").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Snapshot get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Snapshot(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy