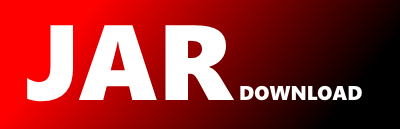
com.pulumi.azurenative.compute.VirtualMachineScaleSetExtension Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.compute;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.compute.VirtualMachineScaleSetExtensionArgs;
import com.pulumi.azurenative.compute.outputs.KeyVaultSecretReferenceResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Describes a Virtual Machine Scale Set Extension.
* Azure REST API version: 2023-03-01. Prior API version in Azure Native 1.x: 2021-03-01.
*
* Other available API versions: 2021-11-01, 2023-07-01, 2023-09-01, 2024-03-01, 2024-07-01.
*
* ## Example Usage
* ### VirtualMachineScaleSetExtension_CreateOrUpdate_MaximumSet_Gen
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.VirtualMachineScaleSetExtension;
* import com.pulumi.azurenative.compute.VirtualMachineScaleSetExtensionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var virtualMachineScaleSetExtension = new VirtualMachineScaleSetExtension("virtualMachineScaleSetExtension", VirtualMachineScaleSetExtensionArgs.builder()
* .autoUpgradeMinorVersion(true)
* .enableAutomaticUpgrade(true)
* .forceUpdateTag("aaaaaaaaa")
* .name("{extension-name}")
* .protectedSettings()
* .provisionAfterExtensions("aa")
* .publisher("{extension-Publisher}")
* .resourceGroupName("rgcompute")
* .settings()
* .suppressFailures(true)
* .type("{extension-Type}")
* .typeHandlerVersion("{handler-version}")
* .vmScaleSetName("aaaaaaa")
* .vmssExtensionName("aaaaaaaaaaaaaaaaaaaaa")
* .build());
*
* }
* }
*
* }
*
* ### VirtualMachineScaleSetExtension_CreateOrUpdate_MinimumSet_Gen
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.VirtualMachineScaleSetExtension;
* import com.pulumi.azurenative.compute.VirtualMachineScaleSetExtensionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var virtualMachineScaleSetExtension = new VirtualMachineScaleSetExtension("virtualMachineScaleSetExtension", VirtualMachineScaleSetExtensionArgs.builder()
* .resourceGroupName("rgcompute")
* .vmScaleSetName("aaaaaaaaaaa")
* .vmssExtensionName("aaaaaaaaaaa")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:compute:VirtualMachineScaleSetExtension {extension-name} /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmScaleSetName}/extensions/{vmssExtensionName}
* ```
*
*/
@ResourceType(type="azure-native:compute:VirtualMachineScaleSetExtension")
public class VirtualMachineScaleSetExtension extends com.pulumi.resources.CustomResource {
/**
* Indicates whether the extension should use a newer minor version if one is available at deployment time. Once deployed, however, the extension will not upgrade minor versions unless redeployed, even with this property set to true.
*
*/
@Export(name="autoUpgradeMinorVersion", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> autoUpgradeMinorVersion;
/**
* @return Indicates whether the extension should use a newer minor version if one is available at deployment time. Once deployed, however, the extension will not upgrade minor versions unless redeployed, even with this property set to true.
*
*/
public Output> autoUpgradeMinorVersion() {
return Codegen.optional(this.autoUpgradeMinorVersion);
}
/**
* Indicates whether the extension should be automatically upgraded by the platform if there is a newer version of the extension available.
*
*/
@Export(name="enableAutomaticUpgrade", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableAutomaticUpgrade;
/**
* @return Indicates whether the extension should be automatically upgraded by the platform if there is a newer version of the extension available.
*
*/
public Output> enableAutomaticUpgrade() {
return Codegen.optional(this.enableAutomaticUpgrade);
}
/**
* If a value is provided and is different from the previous value, the extension handler will be forced to update even if the extension configuration has not changed.
*
*/
@Export(name="forceUpdateTag", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> forceUpdateTag;
/**
* @return If a value is provided and is different from the previous value, the extension handler will be forced to update even if the extension configuration has not changed.
*
*/
public Output> forceUpdateTag() {
return Codegen.optional(this.forceUpdateTag);
}
/**
* The name of the extension.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> name;
/**
* @return The name of the extension.
*
*/
public Output> name() {
return Codegen.optional(this.name);
}
/**
* The extension can contain either protectedSettings or protectedSettingsFromKeyVault or no protected settings at all.
*
*/
@Export(name="protectedSettings", refs={Object.class}, tree="[0]")
private Output* @Nullable */ Object> protectedSettings;
/**
* @return The extension can contain either protectedSettings or protectedSettingsFromKeyVault or no protected settings at all.
*
*/
public Output> protectedSettings() {
return Codegen.optional(this.protectedSettings);
}
/**
* The extensions protected settings that are passed by reference, and consumed from key vault
*
*/
@Export(name="protectedSettingsFromKeyVault", refs={KeyVaultSecretReferenceResponse.class}, tree="[0]")
private Output* @Nullable */ KeyVaultSecretReferenceResponse> protectedSettingsFromKeyVault;
/**
* @return The extensions protected settings that are passed by reference, and consumed from key vault
*
*/
public Output> protectedSettingsFromKeyVault() {
return Codegen.optional(this.protectedSettingsFromKeyVault);
}
/**
* Collection of extension names after which this extension needs to be provisioned.
*
*/
@Export(name="provisionAfterExtensions", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> provisionAfterExtensions;
/**
* @return Collection of extension names after which this extension needs to be provisioned.
*
*/
public Output>> provisionAfterExtensions() {
return Codegen.optional(this.provisionAfterExtensions);
}
/**
* The provisioning state, which only appears in the response.
*
*/
@Export(name="provisioningState", refs={String.class}, tree="[0]")
private Output provisioningState;
/**
* @return The provisioning state, which only appears in the response.
*
*/
public Output provisioningState() {
return this.provisioningState;
}
/**
* The name of the extension handler publisher.
*
*/
@Export(name="publisher", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> publisher;
/**
* @return The name of the extension handler publisher.
*
*/
public Output> publisher() {
return Codegen.optional(this.publisher);
}
/**
* Json formatted public settings for the extension.
*
*/
@Export(name="settings", refs={Object.class}, tree="[0]")
private Output* @Nullable */ Object> settings;
/**
* @return Json formatted public settings for the extension.
*
*/
public Output> settings() {
return Codegen.optional(this.settings);
}
/**
* Indicates whether failures stemming from the extension will be suppressed (Operational failures such as not connecting to the VM will not be suppressed regardless of this value). The default is false.
*
*/
@Export(name="suppressFailures", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> suppressFailures;
/**
* @return Indicates whether failures stemming from the extension will be suppressed (Operational failures such as not connecting to the VM will not be suppressed regardless of this value). The default is false.
*
*/
public Output> suppressFailures() {
return Codegen.optional(this.suppressFailures);
}
/**
* Resource type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Resource type
*
*/
public Output type() {
return this.type;
}
/**
* Specifies the version of the script handler.
*
*/
@Export(name="typeHandlerVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> typeHandlerVersion;
/**
* @return Specifies the version of the script handler.
*
*/
public Output> typeHandlerVersion() {
return Codegen.optional(this.typeHandlerVersion);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public VirtualMachineScaleSetExtension(java.lang.String name) {
this(name, VirtualMachineScaleSetExtensionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public VirtualMachineScaleSetExtension(java.lang.String name, VirtualMachineScaleSetExtensionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public VirtualMachineScaleSetExtension(java.lang.String name, VirtualMachineScaleSetExtensionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:compute:VirtualMachineScaleSetExtension", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private VirtualMachineScaleSetExtension(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:compute:VirtualMachineScaleSetExtension", name, null, makeResourceOptions(options, id), false);
}
private static VirtualMachineScaleSetExtensionArgs makeArgs(VirtualMachineScaleSetExtensionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? VirtualMachineScaleSetExtensionArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:compute/v20170330:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20171201:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20180401:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20180601:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20181001:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20190301:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20190701:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20191201:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20200601:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20201201:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20210301:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20210401:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20210701:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20211101:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20220301:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20220801:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20221101:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230301:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230701:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20230901:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20240301:VirtualMachineScaleSetExtension").build()),
Output.of(Alias.builder().type("azure-native:compute/v20240701:VirtualMachineScaleSetExtension").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static VirtualMachineScaleSetExtension get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new VirtualMachineScaleSetExtension(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy